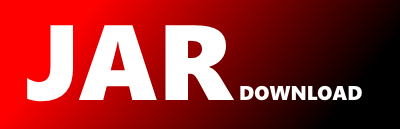
com.ecfeed.core.model.StaticStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.List;
import com.ecfeed.core.utils.EvaluationResult;
import com.ecfeed.core.utils.MessageStack;
public class StaticStatement extends AbstractStatement {
public static final String STATIC_STATEMENT_TRUE_VALUE = "true";
public static final String STATIC_STATEMENT_FALSE_VALUE = "false";
public static final String STATIC_STATEMENT_NULL_VALUE = "null";
private EvaluationResult fValue;
public StaticStatement(EvaluationResult value, IModelChangeRegistrator modelChangeRegistrator) {
super(modelChangeRegistrator);
fValue = value;
}
public StaticStatement(boolean value, IModelChangeRegistrator modelChangeRegistrator) {
super(modelChangeRegistrator);
if (value) {
fValue = EvaluationResult.TRUE;
} else {
fValue = EvaluationResult.FALSE;
}
}
@Override
public EvaluationResult evaluate(List values) {
return fValue;
}
@Override
public String toString() {
return convertToString(fValue);
}
public static String convertToString(EvaluationResult result) {
switch(result) {
case TRUE:
return STATIC_STATEMENT_TRUE_VALUE;
case FALSE:
return STATIC_STATEMENT_FALSE_VALUE;
case INSUFFICIENT_DATA:
return STATIC_STATEMENT_NULL_VALUE;
}
return STATIC_STATEMENT_NULL_VALUE;
}
@Override
public StaticStatement getCopy(){
return new StaticStatement(fValue, getModelChangeRegistrator());
}
@Override
public boolean updateReferences(MethodNode method){
return true;
}
@Override
public boolean compare(IStatement statement){
if(statement instanceof StaticStatement == false){
return false;
}
StaticStatement compared = (StaticStatement)statement;
return getValue() == compared.getValue();
}
@Override
public Object accept(IStatementVisitor visitor) throws Exception {
return visitor.visit(this);
}
@Override
public boolean mentions(int methodParameterIndex) {
return false;
}
public String getLeftOperandName(){
return toString();
}
public EvaluationResult getValue(){
return fValue;
}
public void setValue(boolean value) {
fValue = EvaluationResult.convertFromBoolean(value);
}
@Override
public boolean isAmbiguous(List> values, MessageStack messageStack) {
return false;
}
@Override
public List getListOfChoices() {
return new ArrayList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy