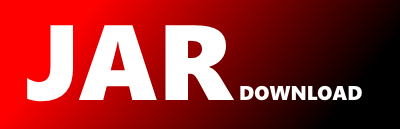
com.ecfeed.core.operations.FactoryRenameOperation Maven / Gradle / Ivy
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.operations;
import java.util.ArrayList;
import java.util.List;
import com.ecfeed.core.model.AbstractNode;
import com.ecfeed.core.model.ChoiceNode;
import com.ecfeed.core.model.ClassNode;
import com.ecfeed.core.model.ClassNodeHelper;
import com.ecfeed.core.model.ConstraintNode;
import com.ecfeed.core.model.GlobalParameterNode;
import com.ecfeed.core.model.IModelVisitor;
import com.ecfeed.core.model.MethodNode;
import com.ecfeed.core.model.MethodParameterNode;
import com.ecfeed.core.model.ModelOperationException;
import com.ecfeed.core.model.RootNode;
import com.ecfeed.core.model.TestCaseNode;
import com.ecfeed.core.utils.JavaLanguageHelper;
import com.ecfeed.core.utils.StringHelper;
import com.ecfeed.core.utils.SystemLogger;
public class FactoryRenameOperation {
private static final String PARTITION_NAME_NOT_UNIQUE_PROBLEM = "Choice name must be unique within a parameter or parent choice";
private static final String CLASS_NAME_CONTAINS_KEYWORD_PROBLEM = "The new class name contains Java keyword";
private static class ClassOperationRename extends GenericOperationRename {
public ClassOperationRename(AbstractNode target, String newName) {
super(target, newName);
}
@Override
public IModelOperation getReverseOperation() {
return new ClassOperationRename(getOwnNode(), getOriginalName());
}
@Override
protected void verifyNewName(String newName) throws ModelOperationException {
for(String token : getNewName().split("\\.")){
if(JavaLanguageHelper.isJavaKeyword(token)){
ModelOperationException.report(CLASS_NAME_CONTAINS_KEYWORD_PROBLEM);
}
}
if(getOwnNode().getSibling(getNewName()) != null){
ModelOperationException.report(OperationMessages.CLASS_NAME_DUPLICATE_PROBLEM);
}
}
}
private static class MethodOperationRename extends GenericOperationRename {
public MethodOperationRename(MethodNode target, String newName){
super(target, newName);
}
@Override
public IModelOperation getReverseOperation() {
return new MethodOperationRename((MethodNode)getOwnNode(), getOriginalName());
}
@Override
protected void verifyNewName(String newName) throws ModelOperationException {
List problems = new ArrayList();
MethodNode target = (MethodNode)getOwnNode();
if(ClassNodeHelper.isNewMethodSignatureValid(target.getClassNode(), getNewName(), target.getParameterTypes(), problems) == false) {
ClassNodeHelper.updateNewMethodsSignatureProblemList(target.getClassNode(), getNewName(), target.getParameterTypes(), problems);
ModelOperationException.report(StringHelper.convertToMultilineString(problems));
}
}
}
private static class GlobalParameterOperationRename extends GenericOperationRename {
public GlobalParameterOperationRename(AbstractNode target, String newName) {
super(target, newName);
}
@Override
public IModelOperation getReverseOperation() {
return new GlobalParameterOperationRename(getOwnNode(), getOriginalName());
}
@Override
protected void verifyNewName(String newName) throws ModelOperationException {
GlobalParameterNode target = (GlobalParameterNode) getOwnNode();
if(JavaLanguageHelper.isJavaKeyword(newName)){
ModelOperationException.report(OperationMessages.CATEGORY_NAME_REGEX_PROBLEM);
}
if(target.getParametersParent().getParameter(newName) != null){
ModelOperationException.report(OperationMessages.CATEGORY_NAME_DUPLICATE_PROBLEM);
}
}
}
private static class MethodParameterOperationRename extends GenericOperationRename {
public MethodParameterOperationRename(AbstractNode target, String newName) {
super(target, newName);
}
@Override
public IModelOperation getReverseOperation() {
return new MethodParameterOperationRename(getOwnNode(), getOriginalName());
}
@Override
protected void verifyNewName(String newName) throws ModelOperationException {
MethodParameterNode target = (MethodParameterNode)getOwnNode();
if(JavaLanguageHelper.isJavaKeyword(newName)){
ModelOperationException.report(OperationMessages.CATEGORY_NAME_REGEX_PROBLEM);
}
if(target.getMethod().getParameter(newName) != null){
ModelOperationException.report(OperationMessages.CATEGORY_NAME_DUPLICATE_PROBLEM);
}
}
}
private static class ChoiceOperationRename extends GenericOperationRename {
public ChoiceOperationRename(ChoiceNode target, String newName){
super(target, newName);
}
@Override
public IModelOperation getReverseOperation() {
return new ChoiceOperationRename((ChoiceNode)getOwnNode(), getOriginalName());
}
@Override
protected void verifyNewName(String newName)throws ModelOperationException{
if(getOwnNode().getSibling(getNewName()) != null){
ModelOperationException.report(PARTITION_NAME_NOT_UNIQUE_PROBLEM);
}
}
}
private static class RenameOperationProvider implements IModelVisitor{
private String fNewName;
public RenameOperationProvider(String newName) {
fNewName = newName;
}
@Override
public Object visit(RootNode node) throws Exception {
return new GenericOperationRename(node, fNewName);
}
@Override
public Object visit(ClassNode node) throws Exception {
return new ClassOperationRename(node, fNewName);
}
@Override
public Object visit(MethodNode node) throws Exception {
return new MethodOperationRename(node, fNewName);
}
@Override
public Object visit(MethodParameterNode node) throws Exception {
return new MethodParameterOperationRename(node, fNewName);
}
@Override
public Object visit(GlobalParameterNode node) throws Exception {
return new GlobalParameterOperationRename(node, fNewName);
}
@Override
public Object visit(TestCaseNode node) throws Exception {
return new GenericOperationRename(node, fNewName);
}
@Override
public Object visit(ConstraintNode node) throws Exception {
return new GenericOperationRename(node, fNewName);
}
@Override
public Object visit(ChoiceNode node) throws Exception {
return new ChoiceOperationRename(node, fNewName);
}
}
public static IModelOperation getRenameOperation(AbstractNode target, String newName){
try{
return (IModelOperation)target.accept(new RenameOperationProvider(newName));
}catch(Exception e){SystemLogger.logCatch(e);}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy