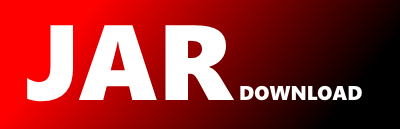
com.ecfeed.core.parser.ArgsAndChoicesParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.core.parser; // TODO - rename package to com.ecfeed.core.genservice.parser ?
import com.ecfeed.core.utils.ExceptionHelper;
import java.util.List;
import java.util.Map;
public class ArgsAndChoicesParser { // TODO - REUSE IN JUNIT5
private enum ChoicesValueType {
NORMAL,
ALL,
NONE
}
private static final String specialValueAllChoices = "ALL";
private static final String specialValueNoneChoices = "NONE";
private ArgsAndChoicesParser.ChoicesValueType fChoicesValueType;
private Map> fArgAndChoiceNames = null;
@SuppressWarnings("unchecked")
public ArgsAndChoicesParser(Object choicesObject) {
if (choicesObject == null) {
fChoicesValueType = ArgsAndChoicesParser.ChoicesValueType.ALL;
return;
}
if (choicesObject instanceof String) {
fChoicesValueType = getChoiceValueType((String) choicesObject);
return;
}
try {
fArgAndChoiceNames = (Map>) choicesObject;
} catch (Exception e) {
ExceptionHelper.reportRuntimeException("Invalid type of choices object. Can not convert to map of arguments with choice names.");
}
if (fArgAndChoiceNames.size() == 0) {
ExceptionHelper.reportRuntimeException("Requested list of choices should not be empty.");
}
validateMap(fArgAndChoiceNames);
}
private void validateMap(Map> argAndChoiceNames) {
for (Map.Entry> mapEntry : argAndChoiceNames.entrySet()) {
validateMapEntry(mapEntry);
}
}
private void validateMapEntry(Map.Entry> mapEntry) {
String parameterName = mapEntry.getKey();
if (parameterName == null) {
ExceptionHelper.reportRuntimeException("Parameter name in choices must not be empty.");
}
List choices = mapEntry.getValue();
if (choices.size() == 0) {
ExceptionHelper.reportRuntimeException("List of choices for parameter: " + parameterName + " must not be empty.");
}
}
public boolean isAllArgsAndChoices() {
if (fChoicesValueType == ArgsAndChoicesParser.ChoicesValueType.ALL) {
return true;
}
return false;
}
public boolean isNoneChoices() {
if (fChoicesValueType == ArgsAndChoicesParser.ChoicesValueType.NONE) {
return true;
}
return false;
}
public Map> getParametersWithChoiceNames() {
return fArgAndChoiceNames;
}
private ArgsAndChoicesParser.ChoicesValueType getChoiceValueType(String choicesString) {
if (choicesString.equals(specialValueNoneChoices)) {
return ArgsAndChoicesParser.ChoicesValueType.NONE;
}
if (choicesString.equals(specialValueAllChoices)) {
return ArgsAndChoicesParser.ChoicesValueType.ALL;
}
ExceptionHelper.reportRuntimeException("Invalid special value for choices: " + choicesString);
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy