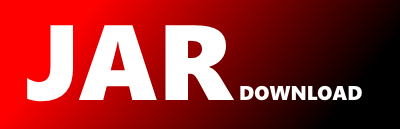
com.ecfeed.core.parser.ConstraintsParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.core.parser;
import com.ecfeed.core.utils.ExceptionHelper;
import java.util.List;
public class ConstraintsParser { // TODO - REUSE IN JUNIT5
private enum ConstraintsValueType {
NORMAL,
ALL,
NONE
}
private static final String specialValueAllConstraints = "ALL";
private static final String specialValueNoneConstraints = "NONE";
private ConstraintsParser.ConstraintsValueType fConstraintsValueType;
private List fConstraintNames = null;
@SuppressWarnings("unchecked")
public ConstraintsParser(Object constraintsObject) {
if (constraintsObject == null) {
fConstraintsValueType = ConstraintsParser.ConstraintsValueType.ALL;
return;
}
if (constraintsObject instanceof String) {
fConstraintsValueType = getConstraintsFromString((String) constraintsObject);
return;
}
try {
fConstraintNames = (List) constraintsObject;
} catch (Exception e) {
ExceptionHelper.reportRuntimeException("Invalid type of constraints object. Can not convert to list of names.");
}
if (fConstraintNames.size() == 0) {
ExceptionHelper.reportRuntimeException("Requested list of constraints should not be empty.");
}
}
public boolean isAllConstraints() {
if (fConstraintsValueType == ConstraintsValueType.ALL) {
return true;
}
return false;
}
public boolean isNoneConstraints() {
if (fConstraintsValueType == ConstraintsValueType.NONE) {
return true;
}
return false;
}
public List getConstraintNames() {
return fConstraintNames;
}
private ConstraintsValueType getConstraintsFromString(String constraintsString) {
if (constraintsString.equals(specialValueNoneConstraints)) {
return ConstraintsValueType.NONE;
}
if (constraintsString.equals(specialValueAllConstraints)) {
return ConstraintsValueType.ALL;
}
ExceptionHelper.reportRuntimeException("Invalid special value for constraints: " + constraintsString);
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy