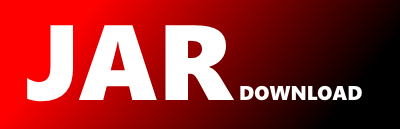
com.ecfeed.core.utils.StreamHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.utils;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Scanner;
public class StreamHelper {
private final static String UTF_8_CODING = "UTF-8";
private final static String REGEX_BEGINNING_OF_TEXT = "\\A";
public static String streamToString(InputStream is) {
Scanner scanner = new Scanner(is, UTF_8_CODING);
String result = null;
try {
result = readFromScanner(scanner);
} finally {
scanner.close();
}
return result;
}
private static String readFromScanner(Scanner scanner) {
if (!scanner.hasNext()) {
return new String();
}
// the entire content will be read
return scanner.useDelimiter(REGEX_BEGINNING_OF_TEXT).next();
}
public static FileOutputStream requireCreateFileOutputStream(String pathWithFileName) {
FileOutputStream outputStream = null;
try {
outputStream = new FileOutputStream(pathWithFileName);
} catch (FileNotFoundException e) {
ExceptionHelper.reportRuntimeException("Can not create output stream." + e.getMessage());
}
return outputStream;
}
public static FileInputStream openInputStream(String filePath) throws EcException {
FileInputStream inputStream = null;
try {
inputStream = new FileInputStream(filePath);
} catch (FileNotFoundException e) {
EcException.report(e.getMessage());
}
return inputStream;
}
public static void closeInputStream(FileInputStream inputStream) throws EcException {
try {
inputStream.close();
} catch (IOException e) {
EcException.report(e.getMessage());
}
}
public static void writeAndFlushLine(String strg, OutputStream outputStream) throws IOException {
outputStream.write(strg.getBytes());
outputStream.write("\n".getBytes());
outputStream.flush();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy