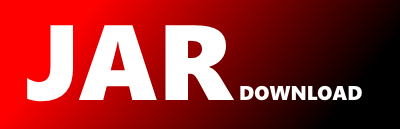
com.ecfeed.core.utils.SystemLogger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.utils;
import java.io.IOException;
import java.util.List;
public class SystemLogger {
private static final String EC_FEED_ERROR = "ECFEEDERR";
private static final String EC_FEED_INFO = "ECFEEDINF";
private static final int ONE_LEVEL_DOWN_ON_STACK = 1; // level1: logCatch
private static final int TWO_LEVELS_DOWN_ON_STACK = 2; // level1: logThrow, level2 SomeException.report
private static final String LOG = "ecFeedLog.txt";
private static boolean fLogToFile = false;
private static boolean fFirstLogError = true;
public static void setLogToFileAndOutput() {
// if (ApplicationContext.isApplicationTypeRemoteRap()) {
// return;
// }
fLogToFile = true;
}
public static void logThrow(String message) {
logSimpleLine( EC_FEED_ERROR + ": Exception thrown");
logIndentedLine("Message: " + message);
StackTraceElement[] stackElements = new Throwable().getStackTrace();
StackTraceElement currentElement = stackElements[TWO_LEVELS_DOWN_ON_STACK];
logCurrentStackElement(currentElement);
logStack(stackElements);
}
public static void logCatch(Exception e) {
logSimpleLine(EC_FEED_ERROR + ": Exception caught");
logIndentedLine("Message: " + ExceptionHelper.createErrorMessage(e));
StackTraceElement element = new Throwable().getStackTrace()[ONE_LEVEL_DOWN_ON_STACK];
logCurrentStackElement(element);
}
private static void logCurrentStackElement(StackTraceElement element) {
logIndentedLine("File: " + element.getFileName());
logIndentedLine("Class: " + element.getClassName());
logIndentedLine("Method: " + element.getMethodName());
logIndentedLine("Line: " + element.getLineNumber());
}
public static void logInfoWithStack(String message) {
logSimpleLine(EC_FEED_INFO + ": " + message);
StackTraceElement[] stackElements = new Throwable().getStackTrace();
StackTraceElement element = stackElements[ONE_LEVEL_DOWN_ON_STACK];
logCurrentStackElement(element);
logStack(stackElements);
}
private static void logStack(StackTraceElement[] stackElements) {
logIndentedLine("Stack:");
for (StackTraceElement element : stackElements) {
logStackElement(element);
}
logEmptyLine();
}
private static void logStackElement(StackTraceElement element) {
logIndented2Line(
"Class: " + element.getClassName() + ", " +
"Method: " + element.getMethodName() + ", " +
"Line: " + element.getLineNumber());
}
private static void logIndentedLine(String line) {
logLine("\t" + line);
}
private static void logIndented2Line(String line) {
logLine("\t\t" + line);
}
private static void logSimpleLine(String line) {
logLine(line);
}
private static void logEmptyLine() {
logLine("");
}
public static void logWarning(String line) {
logLine("WARNING! " + line);
}
public static void logLine(String line) {
System.out.println(line);
if (!fLogToFile) {
return;
}
try {
TextFileHelper.appendLine(LOG, line);
} catch (IOException e) {
if (fFirstLogError) {
fFirstLogError = false;
final String MSG = "Can not write to log file !";
System.out.println(MSG);
}
}
}
public static void logTimedLine(String line) {
logLine(TimeStringHelper.getTimeLongString() + " - " + line);
}
public static void logListOfElements(String message, List list) {
StringBuilder sb = new StringBuilder();
sb.append(message + " - ");
boolean firstTime = true;
for (T element : list) {
if (!firstTime) {
sb.append(", ");
}
sb.append(ObjectHelper.convertToExtendedString(element));
firstTime = false;
}
logLine(sb.toString());
}
public static void logObject(Object object) {
logLine(ObjectHelper.convertToExtendedString(object));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy