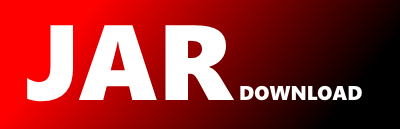
com.ecfeed.junit.AnnotationProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.junit;
import java.lang.annotation.Annotation;
import java.lang.reflect.AnnotatedElement;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.util.Optional;
import com.ecfeed.junit.annotation.*;
import org.apache.commons.codec.digest.DigestUtils;
import org.junit.jupiter.api.extension.ExtensionContext;
import com.ecfeed.core.json.TestCasesUserInputParser;
import com.ecfeed.core.utils.TestCasesUserInput;
import com.ecfeed.core.webservice.client.SecurityHelper;
import com.ecfeed.junit.utils.Localization;
import com.ecfeed.junit.utils.Logger;
public final class AnnotationProcessor {
private AnnotationProcessor() {
RuntimeException exception = new RuntimeException(Localization.bundle.getString("classInitializationError"));
Logger.exception(exception);
throw exception;
}
public static String extractMethodName(ExtensionContext context) {
Optional element = context.getElement();
if (element.isPresent()) {
return element.get().toString();
}
RuntimeException exception = new RuntimeException(Localization.bundle.getString("annotationProcessorNotResolvableMethodNameError"));
Logger.exception(exception);
throw exception;
}
public static Optional extractUserInput(ExtensionContext context) {
return getAnnotationFromRunner(context, EcFeedInput.class);
}
public static Optional extractModel(ExtensionContext context) {
return getAnnotationFromRunner(context, EcFeedModel.class);
}
public static Optional extractEcFeed(ExtensionContext context) {
return getAnnotationFromRunner(context, EcFeed.class);
}
public static Optional extractService(ExtensionContext context) {
return getAnnotationFromRunner(context, EcFeedService.class);
}
public static Optional extractKeyStore(ExtensionContext context) {
return getAnnotationFromRunner(context, EcFeedKeyStore.class);
}
public static String processInput(ExtensionContext context) {
Optional annotation = extractUserInput(context);
if (annotation.isPresent()) {
String extractedInput = annotation.get().value();
Logger.message(Localization.bundle.getString("annotationProcessorInput") + " " + extractedInput);
return extractedInput;
}
return processEcFeedInput(context);
}
public static String processEcFeedInput(ExtensionContext context) {
Optional annotation = extractEcFeed(context);
if (annotation.isPresent()) {
String extractedInput = annotation.get().input();
if (extractedInput.equals(AnnotationDefaultValue.DEFAULT_INPUT)) {
Logger.message(
Localization.bundle.getString("annotationProcessorInputDefault")
+ " " + AnnotationDefaultValue.DEFAULT_INPUT);
} else {
Logger.message(
Localization.bundle.getString("annotationProcessorInput")
+ " " + extractedInput);
}
return extractedInput;
}
Logger.message(
Localization.bundle.getString("annotationProcessorInputDefault")
+ " " + AnnotationDefaultValue.DEFAULT_INPUT);
return AnnotationDefaultValue.DEFAULT_INPUT;
}
public static TestCasesUserInput processInputSchema(ExtensionContext context) {
String extractedInput = "{" + processInput(context).replace("'", "\"") + "}";
return TestCasesUserInputParser.parseRequest(extractedInput);
}
public static String processModelName(ExtensionContext context) {
Optional annotation = extractModel(context);
if (annotation.isPresent()) {
String extractedModelName = annotation.get().value();
Logger.message(Localization.bundle.getString("annotationProcessorModelName") + " " + extractedModelName);
return extractedModelName;
}
return processEcFeedModelName(context);
}
public static String processEcFeedModelName(ExtensionContext context) {
Optional annotation = extractEcFeed(context);
if (annotation.isPresent()) {
String extractedModelName = annotation.get().value();
if (extractedModelName.equals(AnnotationDefaultValue.DEFAULT_MODEL_NAME)) {
Logger.message(
Localization.bundle.getString("annotationProcessorModelNameDefault")
+ " " + AnnotationDefaultValue.DEFAULT_MODEL_NAME);
} else {
Logger.message(
Localization.bundle.getString("annotationProcessorModelName")
+ " " + extractedModelName);
}
return extractedModelName;
}
Logger.message(
Localization.bundle.getString("annotationProcessorModelNameDefault")
+ " " + AnnotationDefaultValue.DEFAULT_MODEL_NAME);
return AnnotationDefaultValue.DEFAULT_MODEL_NAME;
}
public static String processService(ExtensionContext context) {
Optional annotation = extractService(context);
if (annotation.isPresent()) {
String extractedTarget = annotation.get().value();
Logger.message(Localization.bundle.getString("annotationProcessorTarget") + " " + extractedTarget);
return extractedTarget;
}
return processEcFeedService(context);
}
public static String processEcFeedService(ExtensionContext context) {
Optional annotation = extractEcFeed(context);
if (annotation.isPresent()) {
String extractedTarget = annotation.get().target();
if (extractedTarget.equals(AnnotationDefaultValue.DEFAULT_ECFEED_SERVICE)) {
Logger.message(
Localization.bundle.getString("annotationProcessorTargetDefault")
+ " " + AnnotationDefaultValue.DEFAULT_ECFEED_SERVICE);
} else {
Logger.message(
Localization.bundle.getString("annotationProcessorTarget")
+ " " + extractedTarget);
}
return extractedTarget;
}
Logger.message(
Localization.bundle.getString("annotationProcessorTargetDefault")
+ " " + AnnotationDefaultValue.DEFAULT_ECFEED_SERVICE);
return AnnotationDefaultValue.DEFAULT_ECFEED_SERVICE;
}
public static String processKeyStore(ExtensionContext context) {
Optional annotation = extractKeyStore(context);
if (annotation.isPresent()) {
return annotation.get().value();
}
return processEcFeedKeyStore(context);
}
public static String processEcFeedKeyStore(ExtensionContext context) {
Optional annotation = extractEcFeed(context);
if (annotation.isPresent()) {
return annotation.get().keystore();
}
return AnnotationDefaultValue.DEFAULT_KEYSTORE;
}
public static PublicKey processPublicKey(ExtensionContext context) {
PublicKey publicKey = null;
try {
Optional path = Optional.of(processKeyStore(context));
SecurityHelper.getKeyStore(path);
publicKey = SecurityHelper.getPublicKey(null, SecurityHelper.ALIAS_CLIENT);
Logger.message(
Localization.bundle.getString("annotationProcessorPublicKeyRetrieved")
+ " " + DigestUtils.sha256Hex(publicKey.getEncoded()));
} catch (IllegalArgumentException e) {
RuntimeException exception =
new RuntimeException(
Localization.bundle.getString("annotationProcessorPublicKeyNotRetrieved"), e);
exception.addSuppressed(e);
Logger.exception(exception);
throw exception;
}
return publicKey;
}
public static PrivateKey processPrivateKey(ExtensionContext context) {
PrivateKey privateKey = null;
try {
Optional path = Optional.of(processKeyStore(context));
SecurityHelper.getKeyStore(path);
privateKey = SecurityHelper.getPrivateKey(SecurityHelper.ALIAS_CLIENT, SecurityHelper.UNIVERSAL_PASSWORD);
Logger.message(
Localization.bundle.getString("annotationProcessorPrivateKeyRetrieved")
+ " " + DigestUtils.sha256Hex(privateKey.getEncoded()));
} catch (IllegalArgumentException e) {
RuntimeException exception =
new RuntimeException(Localization.bundle.getString("annotationProcessorPrivateKeyNotRetrieved"), e);
exception.addSuppressed(e);
Logger.exception(exception);
throw exception;
}
return privateKey;
}
private static Optional getAnnotationFromRunner(
ExtensionContext context, Class annotation) {
Optional annotatedElement;
annotatedElement = getAnnotationFromMethod(context, annotation);
if (!annotatedElement.isPresent()) {
annotatedElement = getAnnotationFromClass(context, annotation);
}
if (annotatedElement.isPresent()) {
return annotatedElement;
}
return Optional.empty();
}
private static Optional getAnnotationFromMethod(
ExtensionContext context, Class annotation) {
return getAnnotationFromMethod(context.getElement(), annotation);
}
private static Optional getAnnotationFromMethod(
Optional context, Class annotation) {
if (context.isPresent()) {
AnnotatedElement annotatedElement = context.get();
return Optional.ofNullable((T) annotatedElement.getAnnotation(annotation));
}
return Optional.empty();
}
private static Optional getAnnotationFromClass(
ExtensionContext context, Class annotation) {
return getAnnotationFromClass(context.getTestClass(), annotation);
}
private static Optional getAnnotationFromClass(
Optional> element, Class annotation) {
if (element.isPresent()) {
Optional annotatedElement = Optional.ofNullable(element.get().getAnnotation(annotation));
if (annotatedElement.isPresent()) {
return annotatedElement;
}
return getAnnotationFromClass(Optional.ofNullable(element.get().getEnclosingClass()), annotation);
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy