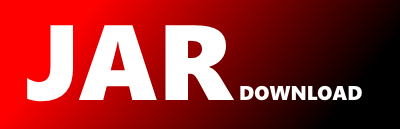
com.ecfeed.junit.main.InputProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.junit.main;
import com.ecfeed.junit.utils.Localization;
import joptsimple.OptionSet;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Optional;
import static com.ecfeed.junit.main.CommandLineConstants.*;
public final class InputProcessor {
static Optional extractFileInputPath(OptionSet options) {
Optional pathProvided = checkRedundantArguments(options, FILE_INPUT_LONG, FILE_INPUT_SHORT);
return pathProvided.isPresent() ? validateFileInput(pathProvided.get()) : Optional.empty();
}
static private Optional checkRedundantArguments(OptionSet options, String nameLong, String nameShort) {
Object parameter = null;
if (options.hasArgument(nameLong)) {
parameter = options.valueOf(nameLong);
}
if (options.hasArgument(nameShort)) {
if (parameter != null) {
throw new IllegalArgumentException(Localization.bundle.getString("inputProcessorDuplicateArguments")
+ nameLong + "', '" + nameShort + "'");
}
parameter = options.valueOf(nameShort);
}
return parameter == null ? Optional.empty() : Optional.of(parameter.toString());
}
static private Optional validateFileInput(String pathProvided) {
Path path = Paths.get(pathProvided);
if (!Files.exists(path)) {
throw new NullPointerException(Localization.bundle.getString("inputProcessorMissingFile") + path);
}
if (!Files.isRegularFile(path)) {
throw new IllegalArgumentException(Localization.bundle.getString("inputProcessorDirectory") + path);
}
if (!Files.isReadable(path)) {
throw new IllegalArgumentException(Localization.bundle.getString("inputProcessorNotReadable") + path);
}
return Optional.ofNullable(path);
}
static Optional extractUserData(OptionSet options) {
return checkRedundantArguments(options, USER_INPUT_LONG, USER_INPUT_SHORT);
}
static boolean extractVerbose(OptionSet options) {
if (options.has(VERBOSE_LONG) || options.has(VERBOSE_SHORT)) {
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy