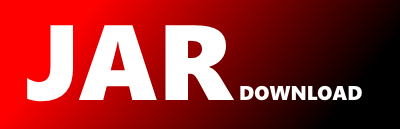
com.ecfeed.junit.main.Main Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.junit.main;
import com.ecfeed.core.generators.algorithms.*;
import com.ecfeed.core.json.TestCasesUserInputParser;
import com.ecfeed.core.model.ChoiceNode;
import com.ecfeed.core.model.IConstraint;
import com.ecfeed.core.model.MethodNode;
import com.ecfeed.core.utils.DataSource;
import com.ecfeed.core.utils.TestCasesUserInput;
import com.ecfeed.junit.AnnotationDefaultValue;
import com.ecfeed.junit.main.processor.TupleProcessorDynamic;
import com.ecfeed.junit.main.processor.TupleProcessorStatic;
import com.ecfeed.junit.runner.UserInputHelper;
import com.ecfeed.junit.utils.Localization;
import joptsimple.OptionParser;
import joptsimple.OptionSet;
import java.nio.file.Path;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import static com.ecfeed.junit.main.CommandLineConstants.*;
public class Main {
private static Path fFileInput;
private static TestCasesUserInput fUserInput;
private static boolean fVerbose;
public static void main(String[] args) throws Exception {
processConsoleInput(parseConsoleInput(args));
Optional> generator = initializeGenerator(fUserInput);
Optional>> list = initializeList(fUserInput);
if (fVerbose) {
new TupleProcessorDynamic(generator, System.out::println).process();
new TupleProcessorStatic(list, System.out::println).process();
} else {
int[] counter = new int[2];
new TupleProcessorDynamic(generator, t -> counter[0]++).process();
new TupleProcessorStatic(list, t -> counter[1]++).process();
System.out.println(counter[0] + " : " + counter[1]);
}
}
private static OptionSet parseConsoleInput(String[] args) {
OptionParser parser = new OptionParser();
parser.accepts(FILE_INPUT_LONG).withRequiredArg();
parser.accepts(FILE_INPUT_SHORT).withRequiredArg();
parser.accepts(USER_INPUT_LONG).withRequiredArg();
parser.accepts(USER_INPUT_SHORT).withRequiredArg();
parser.accepts(VERBOSE_LONG);
parser.accepts(VERBOSE_SHORT);
return parser.parse(args);
}
private static void processConsoleInput(OptionSet options) {
fVerbose = InputProcessor.extractVerbose(options);
fFileInput = InputProcessor.extractFileInputPath(options).orElse(DEFAULT_FILE_INPUT_PATH);
String userDataString = InputProcessor.extractUserData(options).orElse(AnnotationDefaultValue.DEFAULT_INPUT);
userDataString = "{" + userDataString.replaceAll("'", "\"") + "}";
fUserInput = TestCasesUserInputParser.parseRequest(userDataString);
}
private static Optional> initializeGenerator(TestCasesUserInput userData) throws Exception {
Optional> generator = getGenerator(userData);
if (generator.isPresent()) {
setGenerator(generator.get(), userData);
return generator;
}
return Optional.empty();
}
private static Optional>> initializeList(TestCasesUserInput userData) throws Exception {
MethodNode methodNode = getMethodNode(userData);
return Optional.of(UserInputHelper.getTestsFromEcFeedModel(methodNode, Optional.ofNullable(userData.getTestSuites())));
}
private static Optional> getGenerator(TestCasesUserInput userData) throws Exception {
DataSource dataSource = DataSource.parse(userData.getDataSource());
switch (dataSource) {
case GEN_N_WISE :
return Optional.of(new RandomizedNWiseAlgorithm<>(
Integer.parseInt(userData.getN()),
Integer.parseInt(userData.getCoverage())));
case GEN_CARTESIAN :
return Optional.of(new CartesianProductAlgorithm<>());
case GEN_RANDOM:
return Optional.of(new RandomAlgorithm<>(
Integer.parseInt(userData.getLength()),
Boolean.parseBoolean(userData.getDuplicates())));
case GEN_ADAPTIVE_RANDOM :
return Optional.of(new AdaptiveRandomAlgorithm<>(
Integer.parseInt(userData.getDepth()),
Integer.parseInt(userData.getCandidates()),
Integer.parseInt(userData.getLength()),
Boolean.parseBoolean(userData.getDuplicates())));
case STATIC:
return Optional.empty();
default :
RuntimeException exception = new RuntimeException(Localization.bundle.getString("serviceLocalInvalidGeneratorName"));
throw exception;
}
}
private static void setGenerator(AbstractAlgorithm generator, TestCasesUserInput userData) throws Exception {
MethodNode methodNode = getMethodNode(userData);
Collection> generatorDataConstraints = UserInputHelper.getConstraintsFromEcFeedModel(
methodNode,
Optional.ofNullable(userData.getConstraints()));
List> generatorDataInput = UserInputHelper.getChoicesFromEcFeedModel(
methodNode,
Optional.ofNullable(userData.getChoices()));
generator.initialize(generatorDataInput, generatorDataConstraints, null);
}
private static MethodNode getMethodNode(TestCasesUserInput userData) throws Exception {
return UserInputHelper.getMethodNodeFromEcFeedModel(null,
fFileInput.toAbsolutePath().toString(), Optional.ofNullable(userData.getMethod()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy