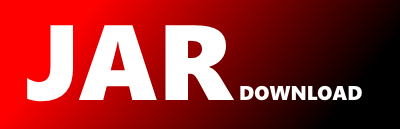
com.echobox.api.linkedin.connection.v2.AssetsConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebx-linkedin-sdk Show documentation
Show all versions of ebx-linkedin-sdk Show documentation
ebx-linkedin-sdk is a pure Java LinkedIn API client. It implements the v2 LinkedIn API.
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.echobox.api.linkedin.connection.v2;
import com.echobox.api.linkedin.client.BinaryAttachment;
import com.echobox.api.linkedin.client.LinkedInClient;
import com.echobox.api.linkedin.client.Parameter;
import com.echobox.api.linkedin.client.WebRequestor;
import com.echobox.api.linkedin.exception.LinkedInNetworkException;
import com.echobox.api.linkedin.types.assets.CheckStatusUpload;
import com.echobox.api.linkedin.types.assets.CompleteMultiPartUploadBody;
import com.echobox.api.linkedin.types.assets.RegisterUpload;
import com.echobox.api.linkedin.types.assets.RegisterUploadRequestBody;
import com.echobox.api.linkedin.types.urn.URN;
import com.echobox.api.linkedin.util.ValidationUtils;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
/**
* Assets connection
* Find the process to upload an asset to Linked in at:
* @see
* Assets API
* @author Joanna
*/
@Deprecated
public class AssetsConnection extends ConnectionBaseV2 {
private static final String ASSETS = "/assets";
private static final String ACTION_KEY = "action";
private static final String REGISTER_UPLOAD = "registerUpload";
private static final String COMPLETE_MULTIPART_UPLOAD = "completeMultiPartUpload";
/**
* Register upload request service relationships identifier
*/
public static final String SERVICE_RELATIONSHIPS_IDENTIFIER = "urn:li:userGeneratedContent";
/**
* Initialise the assets connection
* @param linkedInClient the LinkedIn client
*/
public AssetsConnection(LinkedInClient linkedInClient) {
super(linkedInClient);
}
/**
* Upload an image asset
* @see
*
* Register an Upload for Images and
*
* Upload the Image
* @param registerUploadRequestBody the register upload request body
* @param filename the file name
* @param file the file to upload as an image asset
* @return the digital asset URN
* @throws IOException IOException
*/
public URN uploadImageAsset(RegisterUploadRequestBody registerUploadRequestBody, String filename,
File file) throws IOException {
try (InputStream videoInputStream = new FileInputStream(file)) {
byte[] bytes = new byte[(int) file.length()];
videoInputStream.read(bytes);
return uploadImageAsset(registerUploadRequestBody, filename, bytes);
}
}
/**
* Upload an image asset
* @see
*
* Register an Upload for Images and
*
* Upload the Image
* @param registerUploadRequestBody the register upload request body
* @param filename the file name
* @param bytes the image bytes to upload as an image asset
* @return the digital asset URN
* @throws MalformedURLException MalformedURLException
*/
public URN uploadImageAsset(RegisterUploadRequestBody registerUploadRequestBody, String filename,
byte[] bytes) throws MalformedURLException {
// Register the file upload
RegisterUpload registerUploadResponse = registerUpload(registerUploadRequestBody);
// Upload the image
AssetsConnection.uploadAsset(linkedinClient.getWebRequestor(),
new URL(registerUploadResponse.getValue().getUploadMechanism().getMediaUploadHttpRequest()
.getUploadUrl()), new HashMap<>(), filename, bytes);
return registerUploadResponse.getValue().getAsset();
}
/**
* Register an upload to declare the upcoming upload
* @see
* Register an Upload
* @param registerUploadRequestBody the register upload request body
* @return the register upload response
*/
public RegisterUpload registerUpload(RegisterUploadRequestBody registerUploadRequestBody) {
return linkedinClient.publish(ASSETS, RegisterUpload.class, registerUploadRequestBody,
Parameter.with(ACTION_KEY, REGISTER_UPLOAD));
}
/**
* Upload the asset file
* @see
* Upload the Asset
* @param webRequestor the web requestor - Note that it must not have any existing authorization
* tokens
* @param uploadURL the uploadUrl from the register upload response
* @param headers the headers from the register upload response
* @param filename the file name of the file to be uploaded
* @param file the file to upload
* @return the map of headers from the request
* @throws IOException IOException
*/
public static Map uploadAsset(WebRequestor webRequestor, URL uploadURL,
Map headers, String filename, File file) throws IOException {
try (InputStream videoInputStream = new FileInputStream(file)) {
byte[] bytes = new byte[(int) file.length()];
videoInputStream.read(bytes);
return uploadAsset(webRequestor, uploadURL, headers, filename, bytes);
}
}
// CPD-OFF
/**
* Upload the asset bytes, this should be used to upload each asset chunk
* @see
* Upload the Asset
* @param webRequestor the web requestor - Note that it must not have any existing authorization
* tokens
* @param uploadURL the uploadUrl from the register upload response
* @param headers the headers from the register upload response
* @param filename the file name of the file to be uploaded
* @param bytes the bytes to upload
* @return the map of headers from the request
*/
public static Map uploadAsset(WebRequestor webRequestor, URL uploadURL,
Map headers, String filename, byte[] bytes) {
WebRequestor.Response response;
try {
response = webRequestor.executePut(uploadURL.toString(), null, null, headers,
BinaryAttachment.with(filename, bytes));
} catch (Exception ex) {
throw new LinkedInNetworkException("LinkedIn request failed to upload the asset", ex);
}
ValidationUtils.validateResponse(response);
return response.getHeaders();
}
// CPD-ON
/**
* Complete the multipart upload
* @see
* Complete Multi-Part Upload
* @param completeMultiPartUploadBody the complete multipart upload body
*/
public void completeMultiPartUpload(CompleteMultiPartUploadBody completeMultiPartUploadBody) {
linkedinClient.publish(ASSETS, String.class, completeMultiPartUploadBody, Parameter.with(
ACTION_KEY, COMPLETE_MULTIPART_UPLOAD));
}
/**
* Retrieve the asset information using the asset ID
* @see
* Check Status of Upload
* @param digitalMediaAsset the asset id
* @return the check status upload response
*/
public CheckStatusUpload checkStatusOfUpload(String digitalMediaAsset) {
return linkedinClient.fetchObject(ASSETS + "/" + digitalMediaAsset, CheckStatusUpload.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy