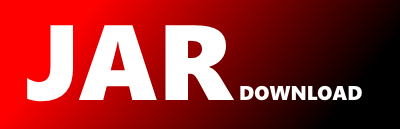
com.echobox.api.tiktok.client.WebRequestor Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2017 Mark Allen, Norbert Bartels.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
//Source - https://restfb.com/
package com.echobox.api.tiktok.client;
import static java.lang.String.format;
import org.apache.commons.lang3.StringUtils;
import java.io.IOException;
import java.util.Map;
/**
* Specifies how a class that sends {@code HTTP} requests to API endpoints must operate.
*
* @author paulp
*/
public interface WebRequestor {
/**
* Encapsulates an HTTP response body and status code.
*
* @author paulp
*/
class Response {
/**
* HTTP response status code (e.g. 200).
*/
private final Integer statusCode;
/**
* HTTP response body as text.
*/
private final String body;
/**
* HTTP response headers (e.g. Content-Type).
*/
private final Map headers;
/**
* Creates a response with the given HTTP status code and response body as text.
*
* @param statusCode The HTTP status code of the response.
* @param headers The headers from the response.
* @param body The response body as text.
*/
public Response(Integer statusCode, Map headers, String body) {
this.statusCode = statusCode;
this.headers = headers;
this.body = StringUtils.trimToEmpty(body);
}
/**
* Gets the HTTP status code.
*
* @return The HTTP status code.
*/
public Integer getStatusCode() {
return statusCode;
}
/**
* Gets the HTTP response body as text.
*
* @return The HTTP response body as text.
*/
public String getBody() {
return body;
}
/**
* Gets the headers of the reponse.
*
* @return The headers of the response.
*/
public Map getHeaders() {
return headers;
}
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
if (StringUtils.isBlank(getBody())) {
return format("HTTP status code %d and an empty response body.", getStatusCode());
}
return format("HTTP status code %d and response body: %s", getStatusCode(), getBody());
}
}
/**
* Given an endpoint URL, execute a {@code GET} against it.
*
* @param url The URL to make a {@code GET} request for, including URL parameters.
*
* @return HTTP response data.
* @throws IOException If an error occurs while performing the {@code GET} operation.
*/
Response executeGet(String url) throws IOException;
/**
* Given an endpoint URL and parameter string, execute a {@code POST} to the endpoint URL.
*
* @param url The URL to {@code POST} to.
* @param jsonBody The POST JSON body.
* @param parameters The parameters to be {@code POST}ed.
*
* @return HTTP response data.
* @throws IOException If an error occurs while performing the {@code POST}.
*/
Response executePost(String url, String jsonBody, String parameters) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy