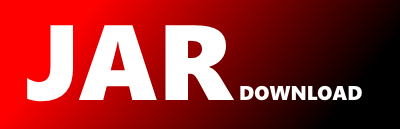
com.eclecticlogic.orc.impl.SchemaSpiImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eclectic-orc Show documentation
Show all versions of eclectic-orc Show documentation
Supports writing Java objects to ORC files.
The newest version!
/*
* Copyright (c) 2017 Eclectic Logic LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.eclecticlogic.orc.impl;
import com.eclecticlogic.orc.Schema;
import com.eclecticlogic.orc.impl.bootstrap.GeneratorUtil;
import com.eclecticlogic.orc.impl.schema.ListChildSchemaColumn;
import com.eclecticlogic.orc.impl.schema.SchemaColumn;
import org.apache.orc.TypeDescription.Category;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Created by kabram
*/
public class SchemaSpiImpl implements SchemaSpi {
final Class schemaClz;
final T proxy, delegateProxy;
Object delegate;
Class> delegateClass;
final List columns = new ArrayList<>();
SchemaColumn currentSchemaColumn;
String lastAccessedProperty;
public SchemaSpiImpl(Class clz) {
schemaClz = clz;
proxy = new ProxyManager<>(this).generate(clz);
delegateProxy = new ProxyManager<>((SchemaSpiImpl)null).generate(clz);
}
@Override
public Schema withDelegate(Class delegate) {
this.delegateClass = delegate;
this.delegate = new ProxyManager<>(this).generate(delegate, new Class[] {schemaClz}, delegateProxy);
return this;
}
@Override
public Class getSchemaClass() {
return schemaClz;
}
@Override
public Schema column(Function columnFunction) {
return column(() -> lastAccessedProperty, (Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy