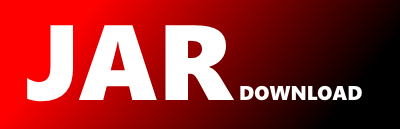
com.eclipsesource.tabris.tracking.TrackingEvent Maven / Gradle / Ivy
Show all versions of tabris-tracking Show documentation
/*******************************************************************************
* Copyright (c) 2014 EclipseSource and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* EclipseSource - initial API and implementation
******************************************************************************/
package com.eclipsesource.tabris.tracking;
import static com.eclipsesource.tabris.internal.Clauses.when;
import static com.eclipsesource.tabris.internal.Clauses.whenNull;
import java.io.Serializable;
import com.eclipsesource.tabris.ui.Page;
/**
*
* A {@link TrackingEvent} will be generated by a {@link Tracking} instance and dispatched to a {@link Tracker}. The
* event contains information regarding the event, the user and the environment.
*
*
* Please note: You not get in touch with a {@link TrackingEvent} unless you will implement your own
* {@link Tracker}.
*
*
* @see Tracker
*
* @since 1.4
*/
@SuppressWarnings("restriction")
public class TrackingEvent implements Serializable {
/**
*
* The {@link EventType} describes the type of the event.
*
*/
public static enum EventType {
/**
*
* A page view event describe when a {@link Page} in the Tabris UI is activated. The id of the page will be in the
* event's details object. See {@link TrackingEvent#getDetail()}.
*
*/
PAGE_VIEW,
/**
*
* An action event describes the Action in a Tabris UI that was executed. The id of the action will be in the
* event's details object. See {@link TrackingEvent#getDetail()}.
*
*/
ACTION,
/**
*
* A search event describes the search in a Tabris UI that was triggered. The query of the search will be in the
* event's details object. See {@link TrackingEvent#getDetail()}.
*
*/
SEARCH,
/**
*
* An order event describes the order that was submitted. The {@link Order} object will be in the
* event's details object. See {@link TrackingEvent#getDetail()}.
*
*/
ORDER,
/**
*
* A simple event describes a custom event that submitted. The id of the event will be in the
* event's details object. See {@link TrackingEvent#getDetail()}.
*
*/
EVENT
}
private final EventType type;
private final TrackingInfo info;
private final Object detail;
private final long timestamp;
public TrackingEvent( EventType type, TrackingInfo info, Object detail, long timestamp ) {
validateArguments( type, info, detail, timestamp );
this.type = type;
this.info = info;
this.detail = detail;
this.timestamp = timestamp;
}
private void validateArguments( EventType type, TrackingInfo info, Object detail, long timestamp ) {
whenNull( type ).throwIllegalArgument( "EventType must not be null." );
whenNull( info ).throwIllegalArgument( "TrackingInfo must not be null." );
whenNull( detail ).throwIllegalArgument( "Detail must not be null." );
when( timestamp < 0 ).throwIllegalArgument( "Timestamp must be > 0 but was " + timestamp );
}
/**
*
* Returns the type of the event.
*
*/
public EventType getType() {
return type;
}
/**
*
* Returns the {@link TrackingInfo} of the event.
*
*/
public TrackingInfo getInfo() {
return info;
}
/**
*
* Returns the details of the event. May be different depending on the type of the event.
*
*/
public Object getDetail() {
return detail;
}
/**
*
* Returns the timestamp of the event. Will be taken from the server time.
*
*/
public long getTimestamp() {
return timestamp;
}
}