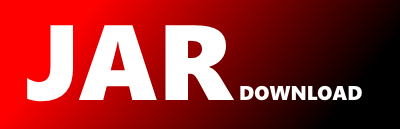
com.ecwid.clickhouse.raw.RawValues.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickhouse-client Show documentation
Show all versions of clickhouse-client Show documentation
Java client for ClickHouse HTTP API (https://clickhouse.com)
package com.ecwid.clickhouse.raw
import java.util.*
class RawValues {
private val values = TreeMap()
fun addScalar(field: String, value: String?) {
values[field] = transform(value)
}
fun addArray(field: String, array: List) {
values[field] = transform(array)
}
fun addMap(field: String, map: Map) {
values[field] = transform(map)
}
fun getFieldsSql(): String {
return values.keys.joinToString(
separator = ",",
prefix = "(",
postfix = ")"
)
}
fun getValues(): String {
return values.values.joinToString(
separator = ",",
prefix = "(",
postfix = ")"
)
}
// if String -> same string
// if List -> ClickHouse array representation [A, B, C....]
// if Map -> ClickHouse map representation {'key1':1,'key2':2....}
private fun transform(value: Any?): String {
return when (value) {
is String? -> value.toString()
is List<*> -> value.joinToString(
separator = ",",
prefix = "[",
postfix = "]"
)
is Map<*, *> -> value.map { kv ->
"${kv.key}:${kv.value}"
}.joinToString(
separator = ",",
prefix = "{",
postfix = "}"
)
else -> throw IllegalArgumentException("Can't convert unknown type into String: $value")
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy