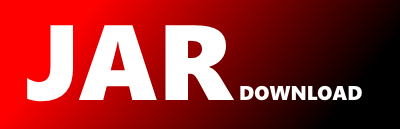
com.edmunds.rest.databricks.service.GroupsServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-rest-client Show documentation
Show all versions of databricks-rest-client Show documentation
A simple java rest client to interact with the Databricks Rest Service
https://docs.databricks.com/api/latest/index.html
package com.edmunds.rest.databricks.service;
import com.edmunds.rest.databricks.DTO.groups.PrincipalNameDTO;
import com.edmunds.rest.databricks.DatabricksRestException;
import com.edmunds.rest.databricks.RequestMethod;
import com.edmunds.rest.databricks.restclient.DatabricksRestClient;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
/**
* The implementation of GroupsService.
*/
public class GroupsServiceImpl extends DatabricksService implements GroupsService {
private static Logger log = LogManager.getLogger(GroupsServiceImpl.class);
private static final PrincipalNameDTO[] EMPTY_MEMBERS_ARRAY = {};
private static final String[] EMPTY_STRING_ARRAY = {};
public GroupsServiceImpl(final DatabricksRestClient client) {
super(client);
}
@Override
public void addUserToGroup(String userName, String parentName) throws IOException, DatabricksRestException {
Map data = new HashMap<>();
data.put("user_name", userName);
data.put("parent_name", parentName);
client.performQuery(RequestMethod.POST, "/groups/add-member", data);
}
@Override
public void addGroupToGroup(String groupName, String parentName) throws IOException, DatabricksRestException {
Map data = new HashMap<>();
data.put("group_name", groupName);
data.put("parent_name", parentName);
client.performQuery(RequestMethod.POST, "/groups/add-member", data);
}
@Override
public String createGroup(String groupName) throws IOException, DatabricksRestException {
boolean groupExists = groupExists(groupName);
// Doing this check and logging rather than letting API throw a "RESOURCE_ALREADY_EXISTS" exception
if (!groupExists) {
Map data = new HashMap<>();
data.put("group_name", groupName);
byte[] responseBody = client.performQuery(RequestMethod.POST, "/groups/create", data);
Map response = this.mapper.readValue(responseBody, Map.class);
Object returnedGroupName = response.get("group_name");
if (returnedGroupName != null) {
return (String) returnedGroupName;
} else {
throw new DatabricksRestException(String.format("There was an issue creating group [%s]. "
+ "No group_name was returned. You may need to reach out to Databricks Support for further diagnosis.",
groupName));
}
} else {
log.info(String.format("Group with name [%s] already exists", groupName));
return groupName;
}
}
@Override
public PrincipalNameDTO[] listMembers(String groupName) throws IOException, DatabricksRestException {
Map data = new HashMap<>();
data.put("group_name", groupName);
byte[] responseBody = client.performQuery(RequestMethod.GET, "/groups/list-members", data);
Map jsonObject = this.mapper
.readValue(responseBody, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy