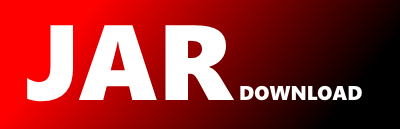
com.edmunds.rest.databricks.service.InstanceProfilesServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-rest-client Show documentation
Show all versions of databricks-rest-client Show documentation
A simple java rest client to interact with the Databricks Rest Service
https://docs.databricks.com/api/latest/index.html
package com.edmunds.rest.databricks.service;
import com.edmunds.rest.databricks.DTO.instance_profiles.InstanceProfileDTO;
import com.edmunds.rest.databricks.DatabricksRestException;
import com.edmunds.rest.databricks.RequestMethod;
import com.edmunds.rest.databricks.restclient.DatabricksRestClient;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class InstanceProfilesServiceImpl extends DatabricksService implements InstanceProfilesService {
private static Logger log = LogManager.getLogger(InstanceProfilesServiceImpl.class);
private static final InstanceProfileDTO[] EMPTY_PROFILE_ARRAY = {};
public InstanceProfilesServiceImpl(final DatabricksRestClient client) {
super(client);
}
@Override
public void add(String instanceProfileArn) throws IOException, DatabricksRestException {
add(instanceProfileArn, false);
}
@Override
public void add(String instanceProfileArn, boolean skipValidation) throws IOException, DatabricksRestException {
Map data = new HashMap<>();
data.put("instance_profile_arn", instanceProfileArn);
data.put("skip_validation", skipValidation);
client.performQuery(RequestMethod.POST, "/instance-profiles/add", data);
}
@Override
public InstanceProfileDTO[] list() throws IOException, DatabricksRestException {
byte[] responseBody = client.performQuery(RequestMethod.GET, "/instance-profiles/list", null);
Map jsonObject = this.mapper
.readValue(responseBody, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy