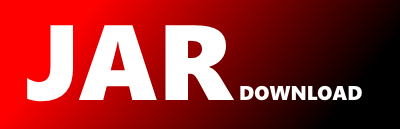
com.edmunds.rest.databricks.service.ScimService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-rest-client Show documentation
Show all versions of databricks-rest-client Show documentation
A simple java rest client to interact with the Databricks Rest Service
https://docs.databricks.com/api/latest/index.html
/*
* Copyright 2018 Edmunds.com, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edmunds.rest.databricks.service;
import com.edmunds.rest.databricks.DTO.scim.ListResponseDTO;
import com.edmunds.rest.databricks.DTO.scim.group.GroupDTO;
import com.edmunds.rest.databricks.DTO.scim.user.UserDTO;
import com.edmunds.rest.databricks.DatabricksRestException;
import java.io.IOException;
/**
* The wrapper around the databricks SCIM API. Note that currently the API is in public preview mode - modifications are
* expected.
*
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#
*/
public interface ScimService {
/**
* Gets an user by id.
*
* @param id user id
* @return POJO representing an user
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-user-by-id
*/
UserDTO getUser(long id) throws IOException, DatabricksRestException;
/**
* Inactivates an user.
*
* @param id user id
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#delete-user-by-id
*/
void deleteUser(long id) throws IOException, DatabricksRestException;
/**
* Retrieve a list of users associated with a Databricks workspace.
*
* @param filters query filters, can be null @see https://docs.databricks.com/dev-tools/api/latest/scim.html#scim-filters
* @return query response
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-users
*/
ListResponseDTO listUsers(String filters) throws IOException, DatabricksRestException;
/**
* Retrieve a list of users associated with a Databricks workspace. No search criteria are provided.
*
* @return query response
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-users
*/
ListResponseDTO listUsers() throws IOException, DatabricksRestException;
/**
* Create a user in the Databricks workspace.
*
* @param userDTO POJO representing an user
* @return the user id
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#create-user
*/
long createUser(UserDTO userDTO) throws IOException, DatabricksRestException;
/**
* Updates an user.
*
* @param userDTO POJO representing an user
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#update-user-by-id-put
*/
void editUser(UserDTO userDTO) throws IOException, DatabricksRestException;
/**
* Gets a group by id.
*
* @param id the group id
* @return POJO representing an group
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-group-by-id
*/
GroupDTO getGroup(long id) throws IOException, DatabricksRestException;
/**
* Removes a group from the workspace. The users associated are not removed
*
* @param id group id
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#delete-group
*/
void deleteGroup(long id) throws IOException, DatabricksRestException;
/**
* Retrieve a list of groups associated with a Databricks workspace.
*
* @param filters query filters, can be null @see https://docs.databricks.com/dev-tools/api/latest/scim.html#scim-filters
* @return query response
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-groups
*/
ListResponseDTO listGroups(String filters) throws IOException, DatabricksRestException;
/**
* Retrieve a list of groups associated with a Databricks workspace. No search criteria are provided.
*
* @return query response
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#get-groups
*/
ListResponseDTO listGroups() throws IOException, DatabricksRestException;
/**
* Creates a group in the Databricks workspace.
*
* @param group POJO representing an group
* @return the group id
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#create-group
*/
long createGroup(GroupDTO group) throws IOException, DatabricksRestException;
/**
* Update a group in Databricks by adding new members.
*
* @param groupId group id
* @param userIds user ids
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#update-group
*/
void addUsersToGroup(long groupId, long[] userIds) throws IOException, DatabricksRestException;
/**
* Update a group in Databricks by removing members.
*
* @param groupId group id
* @param userIds user ids
* @throws IOException any other errors
* @throws DatabricksRestException any errors with request
* @see https://docs.databricks.com/dev-tools/api/latest/scim.html#update-group
*/
void removeUsersFromGroup(long groupId, long[] userIds) throws IOException, DatabricksRestException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy