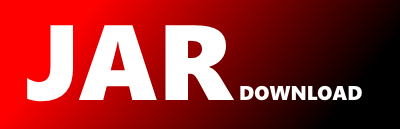
com.edmunds.rest.databricks.service.ScimServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-rest-client Show documentation
Show all versions of databricks-rest-client Show documentation
A simple java rest client to interact with the Databricks Rest Service
https://docs.databricks.com/api/latest/index.html
/*
* Copyright 2018 Edmunds.com, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edmunds.rest.databricks.service;
import com.edmunds.rest.databricks.DTO.scim.ListResponseDTO;
import com.edmunds.rest.databricks.DTO.scim.Operation;
import com.edmunds.rest.databricks.DTO.scim.OperationsDTO;
import com.edmunds.rest.databricks.DTO.scim.group.GroupDTO;
import com.edmunds.rest.databricks.DTO.scim.user.AddUsersToGroupOperation;
import com.edmunds.rest.databricks.DTO.scim.user.RemoveUserFromGroupOperation;
import com.edmunds.rest.databricks.DTO.scim.user.UserDTO;
import com.edmunds.rest.databricks.DatabricksRestException;
import com.edmunds.rest.databricks.RequestMethod;
import com.edmunds.rest.databricks.restclient.DatabricksRestClient;
import com.fasterxml.jackson.core.type.TypeReference;
import java.io.IOException;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.Map;
public class ScimServiceImpl extends DatabricksService implements ScimService {
private static final String SCIM_USERS = "/preview/scim/v2/Users";
private static final String SCIM_GROUPS = "/preview/scim/v2/Groups";
public ScimServiceImpl(final DatabricksRestClient client) {
super(client);
}
private String path(String path, long id) {
return path + "/" + id;
}
@Override
public UserDTO getUser(long id) throws IOException, DatabricksRestException {
byte[] response = client.performQuery(RequestMethod.GET, path(SCIM_USERS, id));
return this.mapper.readValue(response, UserDTO.class);
}
@Override
public void deleteUser(long id) throws DatabricksRestException {
client.performQuery(RequestMethod.DELETE, path(SCIM_USERS, id));
}
@Override
public ListResponseDTO listUsers() throws IOException, DatabricksRestException {
return listUsers(null, 1);
}
@Override
public ListResponseDTO listUsers(String filters) throws IOException, DatabricksRestException {
return listUsers(filters, 1);
}
private ListResponseDTO listUsers(String filters, int startIndex)
throws IOException, DatabricksRestException {
Map params = new HashMap<>();
if (filters != null) {
params.put("filter", URLEncoder.encode(filters, "UTF-8"));
}
params.put("startIndex", startIndex);
byte[] response = client.performQuery(RequestMethod.GET, SCIM_USERS, params);
return mapper.readValue(response, new TypeReference>() {
});
}
@Override
public long createUser(UserDTO userDTO) throws IOException, DatabricksRestException {
String marshalled = mapper.writeValueAsString(userDTO);
Map data = mapper.readValue(marshalled, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy