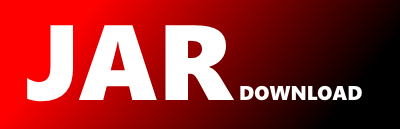
com.efeichong.util.EntityUtils Maven / Gradle / Ivy
package com.efeichong.util;
import com.efeichong.cache.EntityCache;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.ArrayUtils;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import org.springframework.lang.Nullable;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Array;
import java.util.*;
import static com.efeichong.util.StringUtils.isBlank;
/**
* @author lxk
* @date 2020/11/26
* @description 对象操作工具类
*/
@Slf4j
public class EntityUtils {
/**
* 判断两个对象的属性值是否相同
*
* @param source
* @param target
* @param properties 指定字段,默认所有字段一 一对比
* @return
*/
public static boolean propertyHasSimilar(Object source, Object target, boolean ignoreCase, String... properties) {
BeanWrapper src = new BeanWrapperImpl(source);
BeanWrapper trg = new BeanWrapperImpl(target);
boolean isSimilar = true;
if (properties != null && properties.length > 0) {
for (String property : properties) {
isSimilar = propertyHasSimilar(src, trg, property, ignoreCase);
if (!isSimilar) {
return isSimilar;
}
}
} else {
PropertyDescriptor[] pds = src.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
isSimilar = propertyHasSimilar(src, trg, pd.getName(), ignoreCase);
if (!isSimilar) {
return isSimilar;
}
}
}
return isSimilar;
}
/**
* 判断值是否相等
*
* @param src
* @param trg
* @param property
* @param ignoreCase
* @return
*/
private static boolean propertyHasSimilar(BeanWrapper src, BeanWrapper trg, String property, boolean ignoreCase) {
Object srcValue = src.getPropertyValue(property);
Object trgValue = trg.getPropertyValue(property);
if (ignoreCase) {
return equalsIgnoreCase(srcValue, trgValue);
}
return equals(srcValue, trgValue);
}
/**
* 判断两个对象是否相等
*
* @param object1
* @param object2
* @return
*/
public static boolean equals(Object object1, Object object2) {
return object1 == object2 ? true : (object1 != null && object2 != null ? object1.equals(object2) : false);
}
/**
* 判断两个对象是不否相等
*
* @param object1
* @param object2
* @return
*/
public static boolean notEquals(Object object1, Object object2) {
return !equals(object1, object2);
}
/**
* 判断两个对象是否相等,字符串类型忽略大小写
*
* @param object1
* @param object2
* @return
*/
public static boolean equalsIgnoreCase(Object object1, Object object2) {
return object1 == object2 ? true : (object1 != null && object2 != null ?
object1.toString().equalsIgnoreCase(object2.toString()) : false);
}
/**
* 对象字段拷贝(浅拷贝)
*
* @param source
* @param target
* @param ignoreProperties
*/
public static void copyProperties(@NonNull Object source, @NonNull Object target, String... ignoreProperties) {
BeanUtils.copyProperties(source, target, ignoreProperties);
}
/**
* 对象转map
*
* @param source 对象
* @param target map
* @param ignoreProperties
*/
public static void copyProperties(@NonNull Object source, @NonNull Map target, String... ignoreProperties) {
List ignoreList = (ignoreProperties != null ? Arrays.asList(ignoreProperties) : null);
EntityCache entityCache = EntityCache.forClass(source.getClass());
PropertyDescriptor[] propDescriptors = entityCache.getPropDescriptors();
for (PropertyDescriptor propDescriptor : propDescriptors) {
String name = propDescriptor.getName();
if ("class".equals(name)) {
continue;
}
if (ignoreList != null && !ignoreList.contains(name)) {
target.put(name, entityCache.getValue(source, name));
}
}
}
/**
* map转对象
*
* @param source
* @param target
* @param ignoreProperties
*/
public static void copyProperties(@NonNull Map source, @NonNull Object target, String... ignoreProperties) {
List ignoreList = (ignoreProperties != null ? Arrays.asList(ignoreProperties) : null);
EntityCache entityCache = EntityCache.forClass(target.getClass());
PropertyDescriptor[] propDescriptors = entityCache.getPropDescriptors();
for (PropertyDescriptor propDescriptor : propDescriptors) {
String name = propDescriptor.getName();
if (ignoreList != null && !ignoreList.contains(name)) {
entityCache.setValue(target, name, source.get(name));
}
}
}
/**
* 对象字段拷贝(浅拷贝) 忽略空,空字符,空集合,空格,空map
*
* @param source
* @param target
* @param ignoreProperties
*/
public static void copyPropertiesIgnoreNull(Object source, Object target, String... ignoreProperties) {
copyProperties(source, target, getNullPropertyNamesAndConcat(source, ignoreProperties));
}
/**
* 对象字段拷贝(浅拷贝) 忽略空,空字符,空集合,空格,空map
*
* @param source
* @param target
* @param ignoreProperties
* @param canBeNullProps
*/
public static void copyPropertiesIgnoreNull(Object source, Object target, String[] ignoreProperties, String[] canBeNullProps) {
Set nullPropertyNames = getNullPropertyNames(source);
if (ArrayUtils.isNotEmpty(canBeNullProps)) {
Iterator iterator = nullPropertyNames.iterator();
while (iterator.hasNext()) {
boolean anyMatch = Arrays.stream(canBeNullProps).anyMatch(prop -> StringUtils.equalsIgnoreCase(prop, iterator.next()));
if (anyMatch) {
iterator.remove();
}
}
}
int ignorePropertiesSize = 0;
if (ignoreProperties != null) {
ignorePropertiesSize = ignoreProperties.length;
nullPropertyNames.addAll(Arrays.asList(ignoreProperties));
}
String[] result = new String[nullPropertyNames.size() + ignorePropertiesSize];
copyProperties(source, target, nullPropertyNames.toArray(result));
}
/**
* 对象字段拷贝 指定字段
*
* @param source
* @param target
* @param properties
*/
public static void copyPropertiesWithProps(Object source, Object target, String... properties) {
copyProperties(source, target, getIgnorePropertyNames(source, properties));
}
/**
* 对象字段拷贝 指定字段且非空
*
* @param source
* @param target
* @param properties
*/
public static void copyPropertiesWithPropsIgnoreNull(Object source, Object target, String... properties) {
copyProperties(source, target, getNullPropertyNamesAndConcat(source, getIgnorePropertyNames(source, properties)));
}
/**
* 对象字段拷贝 指定字段且非空
*
* @param source
* @param target
* @param properties
*/
public static void copyPropertiesWithPropsIgnoreNull(Object source, Object target, String[] properties, String[] canBeNullProps) {
Set nullPropertyNames = getNullPropertyNames(source);
if (ArrayUtils.isNotEmpty(canBeNullProps)) {
Iterator iterator = nullPropertyNames.iterator();
while (iterator.hasNext()) {
boolean anyMatch = Arrays.stream(canBeNullProps).anyMatch(prop -> StringUtils.equalsIgnoreCase(prop, iterator.next()));
if (anyMatch) {
iterator.remove();
}
}
}
String[] ignoreProperties = getIgnorePropertyNames(source, properties);
int ignorePropertiesSize = 0;
if (ignoreProperties != null) {
ignorePropertiesSize = ignoreProperties.length;
nullPropertyNames.addAll(Arrays.asList(ignoreProperties));
}
String[] result = new String[nullPropertyNames.size() + ignorePropertiesSize];
copyProperties(source, target, nullPropertyNames.toArray(result));
}
/**
* 判断对象是否为空,空字符,空集合,空格,空map
*
* @param obj
* @return
*/
public static boolean isEmpty(@Nullable Object obj) {
if (obj == null) {
return true;
}
if (obj instanceof Optional) {
return !((Optional) obj).isPresent();
}
if (obj instanceof CharSequence) {
CharSequence charSequence = (CharSequence) obj;
return charSequence.length() == 0 && isBlank(charSequence);
}
if (obj.getClass().isArray()) {
return Array.getLength(obj) == 0;
}
if (obj instanceof Collection) {
return ((Collection) obj).isEmpty();
}
if (obj instanceof Map) {
return ((Map) obj).isEmpty();
}
return false;
}
/**
* 判断对象是否不为空,空字符,空集合,空格,空map
*
* @param obj
* @return
*/
public static boolean isNotEmpty(@Nullable Object obj) {
return !isEmpty(obj);
}
/**
* 查询为空的字段(空,空字符,空集合,空格,空map)
*
* @param source
* @param ignoreProperties
* @return
*/
private static String[] getNullPropertyNamesAndConcat(Object source, String[] ignoreProperties) {
BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set emptyNames = new HashSet();
for (PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (isEmpty(srcValue)) {
emptyNames.add(pd.getName());
}
}
int ignorePropertiesSize = 0;
if (ignoreProperties != null) {
ignorePropertiesSize = ignoreProperties.length;
emptyNames.addAll(Arrays.asList(ignoreProperties));
}
String[] result = new String[emptyNames.size() + ignorePropertiesSize];
return emptyNames.toArray(result);
}
/**
* 查询为空的字段(空,空字符,空集合,空格,空map)
*
* @param source
* @return
*/
private static Set getNullPropertyNames(Object source) {
BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set emptyNames = new HashSet();
for (PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (isEmpty(srcValue)) {
emptyNames.add(pd.getName());
}
}
return emptyNames;
}
/**
* 筛选需要忽略拷贝的字段
*
* @param source
* @return
*/
private static String[] getIgnorePropertyNames(Object source, String[] properties) {
BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set ignoreNames = new HashSet();
for (PropertyDescriptor pd : pds) {
if (!ArrayUtils.contains(properties, pd.getName())) {
ignoreNames.add(pd.getName());
}
}
return ignoreNames.toArray(new String[ignoreNames.size()]);
}
/**
* 是否为cglib代理类
*
* @param clazz
* @return
*/
public static boolean isCglibProxy(Class> clazz) {
return clazz.getName().contains("$$BeanGeneratorByCGLIB");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy