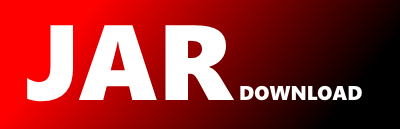
com.efeichong.util.IoUtils Maven / Gradle / Ivy
package com.efeichong.util;
import lombok.Cleanup;
import lombok.SneakyThrows;
import lombok.extern.slf4j.Slf4j;
import java.io.*;
/**
* @author lxk
* @date 2020/12/17
* @description
*/
@Slf4j
public class IoUtils {
/**
* 输入流复制
*
* @param input
* @return
*/
@SneakyThrows
public static InputStream clone(InputStream input) {
@Cleanup ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = input.read(buffer)) > -1) {
baos.write(buffer, 0, len);
}
baos.flush();
return new ByteArrayInputStream(baos.toByteArray());
}
/**
* byte转输入流
*
* @param bytes
* @return
*/
public static InputStream byteToInputStream(byte[] bytes) {
return new ByteArrayInputStream(bytes);
}
/**
* 输入流转byte
*
* @param inputStream
* @return
*/
@SneakyThrows
public static byte[] inputStreamToByte(InputStream inputStream) {
@Cleanup ByteArrayOutputStream output = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int n = 0;
while (-1 != (n = inputStream.read(buffer))) {
output.write(buffer, 0, n);
}
return output.toByteArray();
}
/**
* 文件转byte
*
* @param file
* @return
*/
@SneakyThrows
public static byte[] FileToByte(File file) {
@Cleanup FileInputStream fis = new FileInputStream(file);
@Cleanup ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
int n;
while ((n = fis.read(b)) != -1) {
bos.write(b, 0, n);
}
return bos.toByteArray();
}
/**
* 关闭流
*
* @param closeable
*/
public static void close(Closeable closeable) {
if (closeable != null) {
try {
closeable.close();
} catch (Exception e) {
log.warn("流关闭失败", e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy