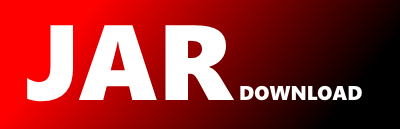
com.eg.agent.android.AndroidAgentImpl Maven / Gradle / Ivy
package com.eg.agent.android;
import android.app.ActivityManager;
import android.app.Application;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager;
import android.content.res.Configuration;
import android.location.Address;
import android.location.Geocoder;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.BatteryManager;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.os.StatFs;
import android.util.Log;
import com.eg.agent.android.analytics.AnalyticAttribute;
import com.eg.agent.android.analytics.AnalyticsControllerImpl;
import com.eg.agent.android.common.TransactionData;
import com.eg.agent.android.crash.crashReport;
import com.eg.agent.android.harvest.ConnectInformation;
import com.eg.agent.android.harvest.Harvest;
import com.eg.agent.android.metric.MetricNames;
import com.eg.agent.android.sample.eGSampler;
import com.eg.agent.android.stats.StatsEngine;
import com.eg.agent.android.util.ActivityLifecycleBackgroundListener;
import com.eg.agent.android.util.ConnectivityInformation;
import com.eg.agent.android.util.DeviceForm;
import com.eg.agent.android.util.Encoder;
import com.eg.agent.android.util.ErrorData;
import com.eg.agent.android.util.UiBackgroundListener;
import com.eg.google.gson.JsonObject;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Timer;
import java.util.concurrent.TimeUnit;
public class AndroidAgentImpl implements MainInterface {
private SavedState savedState;
private Context context;
AgentConfiguration agentConfiguration=new AgentConfiguration();
private ApplicationInformation applicationInformation = ApplicationInformation.getInstance();
private DeviceInformation deviceInformation = DeviceInformation.getInstance();
private EnvironmentInformation environmentInformation = EnvironmentInformation.getInstance();
private MemoryInformation memoryInformation = MemoryInformation.getInstance();
private ResourceInforamtion resourceInforamtion = ResourceInforamtion.getInstance();
private LocationListener locationListener;
String countryCode;
String adminArea;
String deviceform;
String Tag = "eGAgent";
int threadTime = 15000;
//String deviceName = "";
public AndroidAgentImpl() {
}
public AndroidAgentImpl(Context context, AgentConfiguration agentConfiguration) {
this.context = appContext(context);
if (context.getPackageManager().checkPermission("android.permission.ACCESS_FINE_LOCATION", context.getPackageName()) == PackageManager.PERMISSION_GRANTED) {
Log.e("eG", "Location enabled");
try {
addLocationListener();
} catch (Exception e) {
Log.e(Tag, e.getMessage());
}
}
/* if(context!=null)
{
deviceName=Settings.System.getString(context.getContentResolver(), "device_name");
}*/
this.savedState = new SavedState(this.context);
Timer time = new Timer();
eGSampler eGSampler = new eGSampler(context);
time.schedule(eGSampler, 0, threadTime);
crashReport c = new crashReport();
//CrashReporter.initialize(agentConfiguration);
//Application Information
PackageInfo packageInfo = null;
try {
packageInfo = context.getPackageManager().getPackageInfo(context.getPackageName(), 0);
applicationInformation.setPackage_name(packageInfo.packageName);
applicationInformation.setPackage_versionCode(packageInfo.versionCode);
applicationInformation.setAppVersion(packageInfo.versionName);
int stringAppId = context.getApplicationInfo().labelRes;
applicationInformation.setAppName(context.getString(stringAppId));
} catch (PackageManager.NameNotFoundException e) {
e.printStackTrace();
}
//Environment Information
ActivityManager activityManager = (ActivityManager) this.context.getSystemService(Context.ACTIVITY_SERVICE);
long[] free = new long[2];
try {
StatFs rootStatFs = new StatFs(Environment.getRootDirectory().getAbsolutePath());
StatFs externalStatFs = new StatFs(Environment.getExternalStorageDirectory().getAbsolutePath());
if (Build.VERSION.SDK_INT >= 18) {
free[0] = (rootStatFs.getAvailableBlocksLong() * rootStatFs.getBlockSizeLong());
free[1] = (externalStatFs.getAvailableBlocksLong() * rootStatFs.getBlockSizeLong());
} else {
free[0] = (rootStatFs.getAvailableBlocks() * rootStatFs.getBlockSize());
free[1] = (externalStatFs.getAvailableBlocks() * externalStatFs.getBlockSize());
}
} catch (Exception e) {
//AgentHealth.noticeException(e);
} finally {
if (free[0] < 0L) {
free[0] = 0L;
free[0] = 0L;
}
if (free[1] < 0L) {
free[1] = 0L;
}
//Size in bytes
// memoryInformation.setDiskAvailable(free);
}
// memoryInformation.setMemoryUsage(this.context.getWallpaperDesiredMinimumHeight());
int ori = this.context.getResources().getConfiguration().orientation;
String appori;
if (ori == 1) {
appori = "Portrait";
} else {
appori = "Landscape";
}
resourceInforamtion.setOrientation(appori);
resourceInforamtion.setNetworkStatus(getNetworkCarrier());
resourceInforamtion.setNetworkWanType(getNetworkWanType());
//Log.e("countryCode", countryCode);
//Log.e("adminArea",adminArea);
resourceInforamtion.setCountry(countryCode);
resourceInforamtion.setAdminArea(adminArea);
BufferedReader in = null;
try {
Process process = null;
process = Runtime.getRuntime().exec("top -n 1 -d 1");
in = new BufferedReader(new
InputStreamReader(process.getInputStream()));
String line = "";
String content = "";
while ((line = in.readLine()) != null) {
if (line.indexOf(context.getPackageName()) > -1) {
//Log.e("eG","MMM");
//PID PR CPU% S #THR VSS RSS PCY UID Name
//memoryInformation.setPhoneAppMemory(line);
}
}
System.out.println(content);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
//Battery Information
Intent intent = context.registerReceiver(null, new IntentFilter(Intent.ACTION_BATTERY_CHANGED));
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, 0);
int scale = intent.getIntExtra(BatteryManager.EXTRA_SCALE, 100);
int percent = (level * 100) / scale;
resourceInforamtion.setBatterylevel(String.valueOf(percent) + "%");
int status = intent.getIntExtra(BatteryManager.EXTRA_STATUS, -1);
boolean isCharging = status == BatteryManager.BATTERY_STATUS_CHARGING || status == BatteryManager.BATTERY_STATUS_FULL;
if (isCharging) {
resourceInforamtion.setBatteryStatus("Battery Charging");
} else {
resourceInforamtion.setBatteryStatus("Battery Not Charging");
}
// Battery charging type
int chargePlug = intent.getIntExtra(BatteryManager.EXTRA_PLUGGED, -1);
boolean usbCharge = chargePlug == BatteryManager.BATTERY_PLUGGED_USB;
boolean acCharge = chargePlug == BatteryManager.BATTERY_PLUGGED_AC;
if (usbCharge) {
resourceInforamtion.setBatteryChargingType("USB Charging");
} else if (acCharge) {
resourceInforamtion.setBatteryChargingType("AC Charging");
} else
resourceInforamtion.setBatteryChargingType("None");
//battery used by this app //Shanmugapriya 09-Nov-2016
//Check Device type
boolean isTablet = (context.getResources().getConfiguration().screenLayout & Configuration.SCREENLAYOUT_SIZE_MASK) >= Configuration.SCREENLAYOUT_SIZE_LARGE;
if (isTablet) {
this.deviceform = "Tablet";
} else {
this.deviceform = "Phone";
}
//Log.e("eG",deviceform);
resourceInforamtion.setDeviceform(deviceform);
resourceInforamtion.setCarrierName(ConnectivityInformation.carrierNameFromTelephonyManager(context));
if (Build.VERSION.SDK_INT >= 14) {
UiBackgroundListener backgroundListener;
backgroundListener = new ActivityLifecycleBackgroundListener();
if ((backgroundListener instanceof Application.ActivityLifecycleCallbacks)) {
try {
if ((context.getApplicationContext() instanceof Application)) {
Application application = (Application) context.getApplicationContext();
application.registerActivityLifecycleCallbacks((Application.ActivityLifecycleCallbacks) backgroundListener);
}
} catch (Exception e) {
}
} else {
backgroundListener = new UiBackgroundListener();
}
context.registerComponentCallbacks(backgroundListener);
}
}
private void addLocationListener() {
LocationManager locationManager = (LocationManager) this.context.getSystemService(Context.LOCATION_SERVICE);
// getting GPS status
boolean isGPSEnabled = locationManager
.isProviderEnabled(LocationManager.GPS_PROVIDER);
// getting network status
boolean isNetworkEnabled = locationManager
.isProviderEnabled(LocationManager.NETWORK_PROVIDER);
System.out.print(locationManager);
this.locationListener = new LocationListener() {
@Override
public void onLocationChanged(Location location) {
// Log.e("eG", "Location onLocationChanged");
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// Log.e("eG", "Location onStatusChanged");
}
@Override
public void onProviderEnabled(String provider) {
// Log.e("eG", "Location onProviderEnabled");
}
@Override
public void onProviderDisabled(String provider) {
//Log.e("eG", "Location onProviderDisabled");
}
};
if (!isGPSEnabled && !isNetworkEnabled) {
Log.e("eG", "no network provider is enabled");
} else {
if (isNetworkEnabled) {
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 1000L, 0.0F, this.locationListener);
Location location;
location = locationManager.getLastKnownLocation(LocationManager.NETWORK_PROVIDER);
if (location != null) {
double latitude = location.getLatitude();
double longitude = location.getLongitude();
Geocoder coder = new Geocoder(this.context);
List addresses;
try {
addresses = coder.getFromLocation(latitude, longitude, 1);
Address address = (Address) addresses.get(0);
if (address == null) {
return;
}
countryCode = address.getCountryCode();
adminArea = address.getAdminArea();
Log.e("eG", countryCode + adminArea);
setLocation(countryCode, adminArea);
} catch (IOException e) {
}
}
} else {
if (isGPSEnabled) {
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 1000L, 0.0F, this.locationListener);
Location location;
location = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (location != null) {
double latitude = location.getLatitude();
double longitude = location.getLongitude();
Geocoder coder = new Geocoder(this.context);
List addresses;
try {
addresses = coder.getFromLocation(latitude, longitude, 1);
Address address = (Address) addresses.get(0);
if (address == null) {
return;
}
countryCode = address.getCountryCode();
adminArea = address.getAdminArea();
resourceInforamtion.setCountry(countryCode);
resourceInforamtion.setAdminArea(adminArea);
setLocation(countryCode, adminArea);
} catch (IOException e) {
}
}
}
}
}
}
public void setLocation(String countryCode, String adminRegion) {
if ((countryCode == null) || (adminRegion == null)) {
throw new IllegalArgumentException("Country code and administrative region are required.");
}
}
@Override
public boolean waitForConnect(long var1, TimeUnit var3) throws InterruptedException {
return false;
}
@Override
public long getSessionDurationMillis() {
return Harvest.getMillisSinceStart();
}
@Override
public boolean waitForHarvest(long var1, TimeUnit var3) throws InterruptedException {
return false;
}
@Override
public Encoder getEncoder() {
return null;
}
private static Context appContext(Context context) {
if (!(context instanceof Application)) {
return context.getApplicationContext();
}
return context;
}
public static void init(Context context, AgentConfiguration agentConfiguration) {
new AndroidAgentImpl(context, agentConfiguration);
}
@Override
public String getNetworkCarrier() {
return ConnectivityInformation.carrierNameFromContext(this.context);
}
@Override
public String getNetworkWanType() {
return ConnectivityInformation.wanType(this.context);
}
@Override
public DeviceInformation getDeviceInformation() {
DeviceInformation devInfo = new DeviceInformation();
devInfo.setOsName("Android");
devInfo.setOsVersion(Build.VERSION.RELEASE);
devInfo.setAgentName("eGAndroidAgent");
devInfo.setAgentVersion(Agent.getVersion());
devInfo.setManufacturer(Build.MANUFACTURER);
devInfo.setDeviceName(Build.DEVICE);
devInfo.setKernel_version(System.getProperty("os.version"));
devInfo.setID(Build.ID);
devInfo.setOsBuild(Build.VERSION.INCREMENTAL);
devInfo.setModel(Build.MODEL);
devInfo.setDeviceId(Build.ID);
devInfo.setArchitecture(System.getProperty("os.arch"));
devInfo.setRunTime(System.getProperty("java.vm.version"));
devInfo.setSize(deviceForm(this.context).name().toLowerCase());
devInfo.setApplicationPlatform(this.agentConfiguration.getApplicationPlatform());
devInfo.setApplicationPlatformVersion(this.agentConfiguration.getApplicationPlatformVersion());
this.deviceInformation = devInfo;
//devInfo.setDeviceId(System.getProperty( Secure.getString(context.getContentResolver(),Secure.ANDROID_ID)));
//JsonObject dev_info_JSONObj= deviceInformation.asJsonObject();
//return deviceInformation.newFromJson(dev_info_JSONObj);
return this.deviceInformation;
}
public JsonObject getDeviceInformationAsJSON() {
return this.deviceInformation.asJsonObject();
}
@Override
public ApplicationInformation getApplicationInformation() {
return this.applicationInformation;
}
@Override
public EnvironmentInformation getEnvironmentInformation() {
return this.environmentInformation;
}
@Override
public Map eGdata() {
DeviceInformation deviceInformation = getDeviceInformation();
ApplicationInformation applicationInformation = getApplicationInformation();
EnvironmentInformation environmentInformation = getEnvironmentInformation();
HashMap hashMap = new HashMap();
hashMap.put("DeviceInformation1", deviceInformation);
hashMap.put("ApplicationInformation1", applicationInformation);
hashMap.put("EnvironmentInformation", environmentInformation);
return hashMap;
}
@Override
public void addTransactionData(TransactionData var1) {
}
@Override
public List getAndClearTransactionData() {
return null;
}
@Override
public void mergeTransactionData(List var1) {
}
@Override
public void addErrorData(ErrorData var1) {
}
@Override
public List getAndClearErrorData() {
return null;
}
@Override
public void mergeErrorData(List var1) {
}
@Override
public String getCrossProcessId() {
return null;
}
@Override
public int getStackTraceLimit() {
return 0;
}
@Override
public int getResponseBodyLimit() {
return 0;
}
@Override
public void start() {
}
@Override
public void stop() {
}
@Override
public void disable() {
}
@Override
public boolean isDisabled() {
return false;
}
@Override
public MemoryInformation getMemoryInformation() {
return this.memoryInformation;
}
@Override
public ResourceInforamtion getResourceInforamtion() {
return this.resourceInforamtion;
}
private static DeviceForm deviceForm(Context context) {
if(context!=null) {
int deviceSize = context.getResources().getConfiguration().screenLayout & 15;
switch (deviceSize) {
case 1:
return DeviceForm.SMALL;
case 2:
return DeviceForm.NORMAL;
case 3:
return DeviceForm.LARGE;
default:
if (deviceSize > 3) {
return DeviceForm.XLARGE;
}
return DeviceForm.UNKNOWN;
}
}
else
{
return DeviceForm.UNKNOWN;
}
}
@Override
public boolean updateSavedConnectInformation() {
ConnectInformation savedConnectInformation = this.savedState.getConnectInformation();
ConnectInformation newConnectInformation = new ConnectInformation(getApplicationInformation(), getDeviceInformation());
String savedAppToken = this.savedState.getAppToken();
if (newConnectInformation.equals(savedConnectInformation) && this.agentConfiguration.getApplicationToken().equals(savedAppToken)) {
return false;
}
if (newConnectInformation.getApplicationInformation().isAppUpgrade(savedConnectInformation.getApplicationInformation())) {
StatsEngine.get().inc(MetricNames.MOBILE_APP_UPGRADE);
AnalyticsControllerImpl.getInstance().addAttributeUnchecked(new AnalyticAttribute(AnalyticAttribute.APP_UPGRADE_ATTRIBUTE, savedConnectInformation.getApplicationInformation().getAppVersion()), false);
}
this.savedState.clear();
this.savedState.saveConnectInformation(newConnectInformation);
this.savedState.saveAppToken(this.agentConfiguration.getApplicationToken());
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy