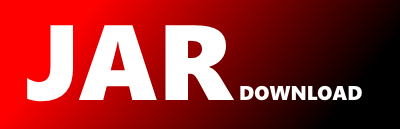
com.eg.agent.android.DeviceInformation Maven / Gradle / Ivy
package com.eg.agent.android;
import com.eg.agent.android.analytics.AnalyticAttribute;
import com.eg.agent.android.harvest.type.HarvestableArray;
import com.eg.google.gson.Gson;
import com.eg.google.gson.JsonArray;
import com.eg.google.gson.JsonObject;
import com.eg.google.gson.JsonPrimitive;
import java.util.HashMap;
import java.util.Map;
public class DeviceInformation extends HarvestableArray {
private ApplicationPlatform applicationPlatform;
private String applicationPlatformVersion;
private String architecture;
public ApplicationPlatform getApplicationPlatform() {
return applicationPlatform;
}
public void setApplicationPlatform(ApplicationPlatform applicationPlatform) {
this.applicationPlatform = applicationPlatform;
}
public String getApplicationPlatformVersion() {
return applicationPlatformVersion;
}
public void setApplicationPlatformVersion(String applicationPlatformVersion) {
this.applicationPlatformVersion = applicationPlatformVersion;
}
public String getArchitecture() {
return architecture;
}
public void setArchitecture(String architecture) {
this.architecture = architecture;
}
public Map getMisc() {
return misc;
}
public void setMisc(Map misc) {
this.misc = misc;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getOsBuild() {
return osBuild;
}
public void setOsBuild(String osBuild) {
this.osBuild = osBuild;
}
public String getRunTime() {
return runTime;
}
public void setRunTime(String runTime) {
this.runTime = runTime;
}
public String getSize() {
return size;
}
public void setSize(String size) {
this.size = size;
}
private Map misc = new HashMap();
private String model;
private String osBuild;
private String runTime;
private String size;
private String osName;
private String osVersion;
private String agentName;
private String agentVersion;
private String deviceId;
public String getDeviceName() {
return deviceName;
}
public void setDeviceName(String deviceName) {
this.deviceName = deviceName;
}
private String deviceName;
private String countryCode;
private String regionCode;
private String manufacturer;
private String kernel_version;
private String ID;
private static DeviceInformation instance=new DeviceInformation();
public static DeviceInformation getInstance(){
return instance;
}
public void setOsName(String osName)
{
this.osName = osName;
}
public void setOsVersion(String osVersion)
{
this.osVersion = osVersion;
}
public void setManufacturer(String manufacturer)
{
this.manufacturer = manufacturer;
}
public void setCountryCode(String countryCode)
{
this.countryCode = countryCode;
}
public void setRegionCode(String regionCode)
{
this.regionCode = regionCode;
}
public void setAgentName(String agentName)
{
this.agentName = agentName;
}
public void setAgentVersion(String agentVersion)
{
this.agentVersion = agentVersion;
}
public void setDeviceId(String deviceId)
{
this.deviceId = deviceId;
}
public String getOsName()
{
return this.osName;
}
public String getOsVersion()
{
return this.osVersion;
}
public String getAgentName()
{
return this.agentName;
}
public String getAgentVersion()
{
return this.agentVersion;
}
public String getDeviceId()
{
return this.deviceId;
}
public String getCountryCode()
{
return this.countryCode;
}
public String getRegionCode()
{
return this.regionCode;
}
public String getManufacturer()
{
return this.manufacturer;
}
public String getKernel_version() {
return this.kernel_version;
}
public void setKernel_version(String kernel_version) {
this.kernel_version = kernel_version;
}
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
public JsonObject asJsonObject()
{
JsonObject data = new JsonObject();
data.add("osName",new JsonPrimitive(this.osName));
data.add("osVersion",new JsonPrimitive(this.osVersion));
data.add("agentName",new JsonPrimitive(this.agentName));
data.add("agentVersion",new JsonPrimitive(this.agentVersion));
data.add("deviceId",new JsonPrimitive(this.deviceId));
data.add("countryCode",new JsonPrimitive(this.countryCode));
data.add("regionCode",new JsonPrimitive(this.regionCode));
data.add("manufacturer",new JsonPrimitive(this.manufacturer));
data.add("kernel_version",new JsonPrimitive(this.kernel_version));
data.add("ID",new JsonPrimitive(this.ID));
data.add("osBuild",new JsonPrimitive(this.osBuild));
data.add("osBuild",new JsonPrimitive(this.osBuild));
data.add("deviceName",new JsonPrimitive(this.deviceName));
return data;
}
public DeviceInformation newFromJson(JsonObject jsonObject)
{
DeviceInformation info = new DeviceInformation();
info.osName=jsonObject.get("osName").getAsString();
info.osVersion=jsonObject.get("osVersion").getAsString();
info.agentName=jsonObject.get("agentName").getAsString();
info.agentVersion=jsonObject.get("agentVersion").getAsString();
info.deviceId=jsonObject.get("deviceId").getAsString();
info.countryCode=jsonObject.get("countryCode").getAsString();
info.regionCode=jsonObject.get("regionCode").getAsString();
info.manufacturer=jsonObject.get("manufacturer").getAsString();
info.kernel_version=jsonObject.get("kernel_version").getAsString();
info.ID=jsonObject.get("ID").getAsString();
info.deviceName=jsonObject.get("deviceName").getAsString();
return info;
}
public JsonArray asJsonArray() {
JsonArray array = new JsonArray();
notEmpty(this.osName);
array.add(new JsonPrimitive(this.osName));
notEmpty(this.osVersion);
array.add(new JsonPrimitive(this.osVersion));
notEmpty(this.manufacturer);
notEmpty(this.model);
array.add(new JsonPrimitive(this.manufacturer + " " + this.model));
notEmpty(this.agentName);
array.add(new JsonPrimitive(this.agentName));
notEmpty(this.agentVersion);
array.add(new JsonPrimitive(this.agentVersion));
notEmpty(this.deviceId);
array.add(new JsonPrimitive(this.deviceId));
array.add(new JsonPrimitive(optional(this.countryCode)));
array.add(new JsonPrimitive(optional(this.regionCode)));
array.add(new JsonPrimitive(this.manufacturer));
Map miscMap = new HashMap();
if (!(this.misc == null || this.misc.isEmpty())) {
miscMap.putAll(this.misc);
}
if (this.applicationPlatform != null) {
miscMap.put(AnalyticAttribute.APPLICATION_PLATFORM_ATTRIBUTE, this.applicationPlatform.toString());
if (this.applicationPlatformVersion != null) {
miscMap.put(AnalyticAttribute.APPLICATION_PLATFORM_VERSION_ATTRIBUTE, this.applicationPlatformVersion);
}
}
array.add(new Gson().toJsonTree(miscMap, GSON_STRING_MAP_TYPE));
return array;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy