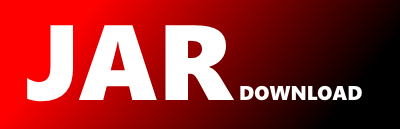
com.eg.agent.android.analytics.AnalyticsControllerImpl Maven / Gradle / Ivy
package com.eg.agent.android.analytics;
/*import com.newrelic.agent.android.AgentConfiguration;
import com.newrelic.agent.android.AgentImpl;
import com.newrelic.agent.android.harvest.DeviceInformation1;
import com.newrelic.agent.android.harvest.EnvironmentInformation;
import com.newrelic.agent.android.logging.AgentLog;
import com.newrelic.agent.android.logging.AgentLogManager;
import com.newrelic.agent.android.tracing.ActivityTrace;
import com.newrelic.agent.android.tracing.TraceLifecycleAware;
import com.newrelic.agent.android.tracing.TraceMachine;*/
import com.eg.agent.android.AgentConfiguration;
import com.eg.agent.android.AndroidAgentImpl;
import com.eg.agent.android.DeviceInformation;
import com.eg.agent.android.EnvironmentInformation;
import com.eg.agent.android.logging.AgentLog;
import com.eg.agent.android.logging.AgentLogManager;
import com.eg.agent.android.trace.ActivityTrace;
import com.eg.agent.android.trace.TraceLifecycleAware;
import com.eg.agent.android.trace.TraceMachine;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicBoolean;
public class AnalyticsControllerImpl implements AnalyticsController {
protected static final int MAX_ATTRIBUTES = 64;
private static final String NEW_RELIC_PREFIX = "newRelic";
private static final String NR_PREFIX = "nr.";
private static final AtomicBoolean initialized = new AtomicBoolean(false);
private static final AnalyticsControllerImpl instance = new AnalyticsControllerImpl();
static final AgentLog log = AgentLogManager.getAgentLog();
private static final List reservedNames = new ArrayList();
private AgentConfiguration agentConfiguration;
private AndroidAgentImpl agentImpl;
private EventManager eventManager = new EventManagerImpl();
private boolean isEnabled;
private InteractionCompleteListener listener = new InteractionCompleteListener();
private Set systemAttributes = Collections.synchronizedSet(new HashSet());
private Set userAttributes = Collections.synchronizedSet(new HashSet());
class InteractionCompleteListener implements TraceLifecycleAware {
InteractionCompleteListener() {
}
public void onEnterMethod() {
}
public void onExitMethod() {
}
public void onTraceStart(ActivityTrace activityTrace) {
}
public void onTraceComplete(ActivityTrace activityTrace) {
AnalyticsControllerImpl.log.verbose("AnalyticsControllerImpl.InteractionCompleteListener.onTraceComplete invoke.");
AnalyticsControllerImpl.getInstance().addEvent(createTraceEvent(activityTrace));
}
private AnalyticsEvent createTraceEvent(ActivityTrace activityTrace) {
float durationInSec = activityTrace.rootTrace.getDurationAsSeconds();
Set attrs = new HashSet();
attrs.add(new AnalyticAttribute(AnalyticAttribute.INTERACTION_DURATION_ATTRIBUTE, durationInSec));
return AnalyticsEventFactory.createEvent(activityTrace.rootTrace.displayName, AnalyticsEventCategory.Interaction, AnalyticAttribute.EVENT_TYPE_ATTRIBUTE_MOBILE, attrs);
}
}
public static void initialize(AgentConfiguration agentConfiguration, AndroidAgentImpl agentImpl) {
log.verbose("AnalyticsControllerImpl.initialize invoked.");
if (initialized.compareAndSet(false, true)) {
instance.clear();
reservedNames.add(AnalyticAttribute.EVENT_TYPE_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.TYPE_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.EVENT_TIMESTAMP_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.EVENT_CATEGORY_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.ACCOUNT_ID_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APP_ID_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APP_NAME_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.UUID_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.SESSION_ID_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.OS_NAME_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.OS_VERSION_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.OS_MAJOR_VERSION_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.DEVICE_MANUFACTURER_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.DEVICE_MODEL_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.MEM_USAGE_MB_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.CARRIER_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.NEW_RELIC_VERSION_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.INTERACTION_DURATION_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APP_INSTALL_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APP_UPGRADE_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APPLICATION_PLATFORM_ATTRIBUTE);
reservedNames.add(AnalyticAttribute.APPLICATION_PLATFORM_VERSION_ATTRIBUTE);
instance.reinitialize(agentConfiguration, agentImpl);
TraceMachine.addTraceListener(instance.listener);
log.info("Analytics Controller started.");
return;
}
log.verbose("AnalyticsControllerImpl has already been initialized. Bypassing..");
}
public static void shutdown() {
TraceMachine.removeTraceListener(instance.listener);
initialized.compareAndSet(true, false);
instance.getEventManager().shutdown();
}
private AnalyticsControllerImpl() {
}
void reinitialize(AgentConfiguration agentConfiguration, AndroidAgentImpl agentImpl) {
String osMajorVersion;
this.agentImpl = agentImpl;
this.agentConfiguration = agentConfiguration;
this.eventManager.initialize();
this.isEnabled = agentConfiguration.getEnableAnalyticsEvents();
loadPersistentAttributes();
DeviceInformation deviceInformation = agentImpl.getDeviceInformation();
String osVersion = deviceInformation.getOsVersion().replace(" ", "");
String[] osMajorVersionArr = osVersion.split("[.:-]");
if (osMajorVersionArr.length > 0) {
osMajorVersion = osMajorVersionArr[0];
} else {
osMajorVersion = osVersion;
}
EnvironmentInformation environmentInformation = agentImpl.getEnvironmentInformation();
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.OS_NAME_ATTRIBUTE, deviceInformation.getOsName()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.OS_VERSION_ATTRIBUTE, osVersion));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.OS_MAJOR_VERSION_ATTRIBUTE, osMajorVersion));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.DEVICE_MANUFACTURER_ATTRIBUTE, deviceInformation.getManufacturer()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.DEVICE_MODEL_ATTRIBUTE, deviceInformation.getModel()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.UUID_ATTRIBUTE, deviceInformation.getDeviceId()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.CARRIER_ATTRIBUTE, agentImpl.getNetworkCarrier()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.NEW_RELIC_VERSION_ATTRIBUTE, deviceInformation.getAgentVersion()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.MEM_USAGE_MB_ATTRIBUTE, (float) environmentInformation.getMemoryUsage()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.SESSION_ID_ATTRIBUTE, agentConfiguration.getSessionID()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.APPLICATION_PLATFORM_ATTRIBUTE, agentConfiguration.getApplicationPlatform().toString()));
this.systemAttributes.add(new AnalyticAttribute(AnalyticAttribute.APPLICATION_PLATFORM_VERSION_ATTRIBUTE, agentConfiguration.getApplicationPlatformVersion()));
}
public AnalyticAttribute getAttribute(String name) {
log.verbose("AnalyticsControllerImpl.getAttribute - retrieving " + name);
AnalyticAttribute attribute = getUserAttribute(name);
if (attribute == null) {
return getSystemAttribute(name);
}
return attribute;
}
public Set getSystemAttributes() {
Set attrs = new HashSet(this.systemAttributes.size());
for (AnalyticAttribute attr : this.systemAttributes) {
attrs.add(new AnalyticAttribute(attr));
}
return Collections.unmodifiableSet(attrs);
}
public Set getUserAttributes() {
Set attrs = new HashSet(this.userAttributes.size());
for (AnalyticAttribute attr : this.userAttributes) {
attrs.add(new AnalyticAttribute(attr));
}
return Collections.unmodifiableSet(attrs);
}
public Set getSessionAttributes() {
Set attrs = new HashSet(getSessionAttributeCount());
attrs.addAll(getSystemAttributes());
attrs.addAll(getUserAttributes());
return Collections.unmodifiableSet(attrs);
}
public int getSystemAttributeCount() {
return this.systemAttributes.size();
}
public int getUserAttributeCount() {
return this.userAttributes.size();
}
public int getSessionAttributeCount() {
return this.systemAttributes.size() + this.userAttributes.size();
}
public boolean setAttribute(String name, String value) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value);
return setAttribute(name, value, true);
}
public boolean setAttribute(String name, String value, boolean persistent) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value + (persistent ? " (persistent)" : " (transient)"));
if (!isInitializedAndEnabled() || !isAttributeNameValid(name) || !isStringValueValid(name, value)) {
return false;
}
AnalyticAttribute attribute = getAttribute(name);
boolean stored;
if (attribute != null) {
attribute.setStringValue(value);
attribute.setPersistent(persistent);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
this.agentConfiguration.getAnalyticAttributeStore().delete(attribute);
} else if (this.userAttributes.size() < 64) {
attribute = new AnalyticAttribute(name, value, persistent);
this.userAttributes.add(attribute);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
} else {
log.warning("Attribute limit exceeded: at most 64 are allowed.");
log.warning("Currently defined attributes:");
for (AnalyticAttribute attr : this.userAttributes) {
log.warning("\t" + attr.getName() + ": " + attr.valueAsString());
}
}
return true;
}
public boolean setAttribute(String name, float value) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value);
return setAttribute(name, value, true);
}
public boolean setAttribute(String name, float value, boolean persistent) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value + (persistent ? " (persistent)" : " (transient)"));
if (!isInitializedAndEnabled() || !isAttributeNameValid(name)) {
return false;
}
AnalyticAttribute attribute = getAttribute(name);
boolean stored;
if (attribute != null) {
attribute.setFloatValue(value);
attribute.setPersistent(persistent);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
this.agentConfiguration.getAnalyticAttributeStore().delete(attribute);
} else if (this.userAttributes.size() < 64) {
attribute = new AnalyticAttribute(name, value, persistent);
this.userAttributes.add(attribute);
if (attribute.isPersistent()) {
this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
} else {
log.warning("Attribute limit exceeded: at most 64 are allowed.");
log.warning("Currently defined attributes:");
for (AnalyticAttribute attr : this.userAttributes) {
log.warning("\t" + attr.getName() + ": " + attr.valueAsString());
}
}
return true;
}
public boolean setAttribute(String name, boolean value) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value);
return setAttribute(name, value, true);
}
public boolean setAttribute(String name, boolean value, boolean persistent) {
log.verbose("AnalyticsControllerImpl.setAttribute - " + name + ": " + value + (persistent ? " (persistent)" : " (transient)"));
if (!isInitializedAndEnabled() || !isAttributeNameValid(name)) {
return false;
}
AnalyticAttribute attribute = getAttribute(name);
boolean stored;
if (attribute != null) {
attribute.setBooleanValue(value);
attribute.setPersistent(persistent);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
this.agentConfiguration.getAnalyticAttributeStore().delete(attribute);
} else if (this.userAttributes.size() < 64) {
attribute = new AnalyticAttribute(name, value, persistent);
this.userAttributes.add(attribute);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
} else {
log.warning("Attribute limit exceeded: at most 64 are allowed.");
log.warning("Currently defined attributes:");
for (AnalyticAttribute attr : this.userAttributes) {
log.warning("\t" + attr.getName() + ": " + attr.valueAsString());
}
}
return true;
}
public boolean addAttributeUnchecked(AnalyticAttribute attribute, boolean persistent) {
String name = attribute.getName();
log.verbose("AnalyticsControllerImpl.setAttributeUnchecked - " + name + ": " + attribute.valueAsString() + (persistent ? " (persistent)" : " (transient)"));
if (!initialized.get()) {
log.warning("Analytics controller is not initialized!");
return false;
} else if (!this.isEnabled) {
log.warning("Analytics controller is not enabled!");
return false;
} else if (!isNameValid(name)) {
return false;
} else {
AnalyticAttribute foundAttribute = getAttribute(attribute.getName());
boolean stored;
if (foundAttribute == null) {
this.userAttributes.add(attribute);
if (attribute.isPersistent()) {
this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
}
switch (attribute.getAttributeDataType()) {
case STRING:
foundAttribute.setStringValue(attribute.getStringValue());
break;
case FLOAT:
foundAttribute.setFloatValue(attribute.getFloatValue());
break;
case BOOLEAN:
foundAttribute.setBooleanValue(attribute.getBooleanValue());
break;
}
foundAttribute.setPersistent(persistent);
if (foundAttribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(foundAttribute);
if (!stored) {
log.error("Failed to store attribute " + foundAttribute + " to attribute store.");
return stored;
}
}
this.agentConfiguration.getAnalyticAttributeStore().delete(foundAttribute);
return true;
}
}
public boolean incrementAttribute(String name, float value) {
log.verbose("AnalyticsControllerImpl.incrementAttribute - " + name + ": " + value);
return incrementAttribute(name, value, true);
}
public boolean incrementAttribute(String name, float value, boolean persistent) {
log.verbose("AnalyticsControllerImpl.incrementAttribute - " + name + ": " + value + (persistent ? " (persistent)" : " (transient)"));
if (!isInitializedAndEnabled() || !isAttributeNameValid(name)) {
return false;
}
AnalyticAttribute attribute = getAttribute(name);
boolean stored;
if (attribute != null && attribute.isFloatAttribute()) {
attribute.setFloatValue(attribute.getFloatValue() + value);
attribute.setPersistent(persistent);
if (attribute.isPersistent()) {
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
} else if (attribute != null) {
log.warning("Cannot increment attribute " + name + ": the attribute is already defined as a non-float value.");
return false;
} else if (this.userAttributes.size() < 64) {
attribute = new AnalyticAttribute(name, value, persistent);
this.userAttributes.add(attribute);
if (attribute.isPersistent()) {
this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
stored = this.agentConfiguration.getAnalyticAttributeStore().store(attribute);
if (!stored) {
log.error("Failed to store attribute " + attribute + " to attribute store.");
return stored;
}
}
this.agentConfiguration.getAnalyticAttributeStore().delete(attribute);
}
return true;
}
public boolean removeAttribute(String name) {
log.verbose("AnalyticsControllerImpl.removeAttribute - " + name);
if (!isInitializedAndEnabled()) {
return false;
}
AnalyticAttribute attribute = getAttribute(name);
if (attribute != null) {
this.userAttributes.remove(attribute);
if (attribute.isPersistent()) {
this.agentConfiguration.getAnalyticAttributeStore().delete(attribute);
}
}
return true;
}
public boolean removeAllAttributes() {
log.verbose("AnalyticsControllerImpl.removeAttributes - ");
if (isInitializedAndEnabled()) {
this.agentConfiguration.getAnalyticAttributeStore().clear();
this.userAttributes.clear();
}
return false;
}
public boolean addEvent(String name, Set eventAttributes) {
return addEvent(name, AnalyticsEventCategory.Custom, AnalyticAttribute.EVENT_TYPE_ATTRIBUTE_MOBILE, eventAttributes);
}
public boolean addEvent(String name, AnalyticsEventCategory eventCategory, String eventType, Set eventAttributes) {
log.verbose("AnalyticsControllerImpl.addEvent - " + name + ": category=" + eventCategory + ", eventType: " + eventType + ", eventAttributes:" + eventAttributes);
if (!isInitializedAndEnabled()) {
return false;
}
Set validatedAttributes = new HashSet();
for (AnalyticAttribute attribute : eventAttributes) {
if (isAttributeNameValid(attribute.getName())) {
validatedAttributes.add(attribute);
}
}
return addEvent(AnalyticsEventFactory.createEvent(name, eventCategory, eventType, validatedAttributes));
}
public boolean addEvent(AnalyticsEvent event) {
if (!isInitializedAndEnabled()) {
return false;
}
Set sessionAttributes = new HashSet();
long sessionDuration = this.agentImpl.getSessionDurationMillis();
if (0 == sessionDuration) {
log.error("Harvest instance is not running! Session duration will be invalid");
} else {
sessionAttributes.add(new AnalyticAttribute("timeSinceLoad", ((float) sessionDuration) / 1000.0f));
event.addAttributes(sessionAttributes);
}
return this.eventManager.addEvent(event);
}
public int getMaxEventPoolSize() {
return this.eventManager.getMaxEventPoolSize();
}
public void setMaxEventPoolSize(int maxSize) {
this.eventManager.setMaxEventPoolSize(maxSize);
}
public void setMaxEventBufferTime(int maxBufferTimeInSec) {
this.eventManager.setMaxEventBufferTime(maxBufferTimeInSec);
}
public int getMaxEventBufferTime() {
return this.eventManager.getMaxEventBufferTime();
}
public EventManager getEventManager() {
return this.eventManager;
}
public static AnalyticsControllerImpl getInstance() {
return instance;
}
void loadPersistentAttributes() {
log.verbose("AnalyticsControllerImpl.loadPersistentAttributes - loading userAttributes from the attribute store...");
List storedAttrs = this.agentConfiguration.getAnalyticAttributeStore().fetchAll();
log.verbose("AnalyticsControllerImpl.loadPersistentAttributes - found " + storedAttrs.size() + " userAttributes in the attribute store...");
for (AnalyticAttribute attr : storedAttrs) {
this.userAttributes.add(attr);
}
}
private AnalyticAttribute getSystemAttribute(String name) {
for (AnalyticAttribute nextAttribute : this.systemAttributes) {
if (nextAttribute.getName().equals(name)) {
return nextAttribute;
}
}
return null;
}
private AnalyticAttribute getUserAttribute(String name) {
for (AnalyticAttribute nextAttribute : this.userAttributes) {
if (nextAttribute.getName().equals(name)) {
return nextAttribute;
}
}
return null;
}
private void clear() {
log.verbose("AnalyticsControllerImpl.clear - clearing out attributes and events");
this.systemAttributes.clear();
this.userAttributes.clear();
this.eventManager.empty();
}
private boolean isAttributeNameValid(String name) {
boolean valid = isNameValid(name);
if (valid) {
valid = !isNameReserved(name);
if (!valid) {
log.error("Attribute name " + name + " is reserved for internal use and will be ignored.");
}
}
return valid;
}
private boolean isNameValid(String name) {
boolean valid = (name == null || name.equals("") || name.length() >= 256) ? false : true;
if (!valid) {
log.error("Attribute name " + name + " is null, empty, or exceeds the maximum length of " + 256 + " characters.");
}
return valid;
}
private boolean isStringValueValid(String name, String value) {
boolean valid = (value == null || value.equals("") || value.getBytes().length >= 4096) ? false : true;
if (!valid) {
log.error("Attribute value for name " + name + " is null, empty, or exceeds the maximum length of " + 4096 + " bytes.");
}
return valid;
}
private boolean isNameReserved(String name) {
boolean isReserved = reservedNames.contains(name);
if (isReserved) {
log.verbose("Name " + name + " is in the reserved names list.");
return isReserved;
}
if (isReserved || name.startsWith(NEW_RELIC_PREFIX)) {
isReserved = true;
} else {
isReserved = false;
}
if (isReserved) {
log.verbose("Name " + name + " starts with reserved prefix " + NEW_RELIC_PREFIX);
return isReserved;
}
if (isReserved || name.startsWith(NR_PREFIX)) {
isReserved = true;
} else {
isReserved = false;
}
if (isReserved) {
log.verbose("Name " + name + " starts with reserved prefix " + NR_PREFIX);
}
return isReserved;
}
public boolean recordEvent(String name, Map eventAttributes) {
log.verbose("AnalyticsControllerImpl.recordEvent - " + name + ": " + eventAttributes.size() + " attributes");
if (!isInitializedAndEnabled()) {
return false;
}
Set attributes = new HashSet();
try {
for (String key : eventAttributes.keySet()) {
Object value = eventAttributes.get(key);
try {
if (value instanceof String) {
attributes.add(new AnalyticAttribute(key, String.valueOf(value)));
} else if (value instanceof Float) {
attributes.add(new AnalyticAttribute(key, Float.valueOf(((Float) value).floatValue()).floatValue()));
} else if (value instanceof Double) {
attributes.add(new AnalyticAttribute(key, Float.valueOf(((Double) value).floatValue()).floatValue()));
} else if (value instanceof Integer) {
attributes.add(new AnalyticAttribute(key, Float.valueOf((float) ((Integer) value).intValue()).floatValue()));
} else if (value instanceof Short) {
attributes.add(new AnalyticAttribute(key, Float.valueOf((float) ((Short) value).shortValue()).floatValue()));
} else if (value instanceof Long) {
attributes.add(new AnalyticAttribute(key, Float.valueOf((float) ((Long) value).longValue()).floatValue()));
} else if (value instanceof BigDecimal) {
attributes.add(new AnalyticAttribute(key, Float.valueOf(((BigDecimal) value).floatValue()).floatValue()));
} else if (value instanceof BigInteger) {
attributes.add(new AnalyticAttribute(key, Float.valueOf(((BigInteger) value).floatValue()).floatValue()));
} else if (value instanceof Boolean) {
attributes.add(new AnalyticAttribute(key, Boolean.valueOf(((Boolean) value).booleanValue()).booleanValue()));
} else {
log.error("Unsupported event attribute type for key [" + key + "]: " + value.getClass().getName());
return false;
}
} catch (ClassCastException e) {
log.error(String.format("Error casting attribute [%s] to String or Float: ", new Object[]{key}), e);
}
}
} catch (Exception e2) {
log.error(String.format("Error occurred while recording event [%s]: ", new Object[]{name}), e2);
}
return addEvent(name, AnalyticsEventCategory.Custom, AnalyticAttribute.EVENT_TYPE_ATTRIBUTE_MOBILE, attributes);
}
private boolean isInitializedAndEnabled() {
if (!initialized.get()) {
log.warning("Analytics controller is not initialized!");
return false;
} else if (this.isEnabled) {
return true;
} else {
log.warning("Analytics controller is not enabled!");
return false;
}
}
public void setEnabled(boolean enabled) {
this.isEnabled = enabled;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy