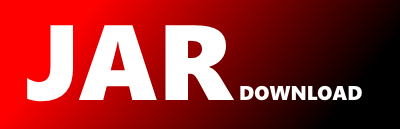
com.eg.agent.android.api.common.ConnectionState Maven / Gradle / Ivy
package com.eg.agent.android.api.common;
import java.util.concurrent.TimeUnit;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
import static java.util.concurrent.TimeUnit.SECONDS;
public final class ConnectionState
{
private final Object dataToken;
private final String crossProcessId;
private final long serverTimestamp;
private final long harvestInterval;
private final TimeUnit harvestIntervalTimeUnit;
private final long maxTransactionAge;
private final TimeUnit maxTransactionAgeTimeUnit;
private final long maxTransactionCount;
private final int stackTraceLimit;
private final int responseBodyLimit;
private final boolean collectingNetworkErrors;
private final int errorLimit;
public static final ConnectionState NULL = new ConnectionState();
private ConnectionState() {
this.dataToken = null;
this.crossProcessId = null;
this.serverTimestamp = 0L;
this.harvestInterval = 60L;
this.harvestIntervalTimeUnit = SECONDS;
this.maxTransactionAge = 600L;
this.maxTransactionAgeTimeUnit = SECONDS;
this.maxTransactionCount = 1000L;
this.stackTraceLimit = 50;
this.responseBodyLimit = 1024;
this.collectingNetworkErrors = true;
this.errorLimit = 10;
}
public ConnectionState(Object dataToken, String crossProcessId, long serverTimestamp, long harvestInterval, TimeUnit harvestIntervalTimeUnit, long maxTransactionAge, TimeUnit maxTransactionAgeTimeUnit, long maxTransactionCount, int stackTraceLimit, int responseBodyLimit, boolean collectingNetworkerrors, int errorLimit) {
this.dataToken = dataToken;
this.crossProcessId = crossProcessId;
this.serverTimestamp = serverTimestamp;
this.harvestInterval = harvestInterval;
this.harvestIntervalTimeUnit = harvestIntervalTimeUnit;
this.maxTransactionAge = maxTransactionAge;
this.maxTransactionAgeTimeUnit = maxTransactionAgeTimeUnit;
this.maxTransactionCount = maxTransactionCount;
this.stackTraceLimit = stackTraceLimit;
this.responseBodyLimit = responseBodyLimit;
this.collectingNetworkErrors = collectingNetworkerrors;
this.errorLimit = errorLimit;
}
public Object getDataToken() {
return this.dataToken;
}
public String getCrossProcessId() {
return this.crossProcessId;
}
public long getServerTimestamp() {
return this.serverTimestamp;
}
public long getHarvestIntervalInSeconds() {
return TimeUnit.SECONDS.convert(this.harvestInterval, this.harvestIntervalTimeUnit);
}
public long getHarvestIntervalInMilliseconds() {
return MILLISECONDS.convert(this.harvestInterval, this.harvestIntervalTimeUnit);
}
public long getMaxTransactionAgeInSeconds() {
return SECONDS.convert(this.maxTransactionAge, this.maxTransactionAgeTimeUnit);
}
public long getMaxTransactionAgeInMilliseconds() {
return TimeUnit.MILLISECONDS.convert(this.maxTransactionAge, this.maxTransactionAgeTimeUnit);
}
public long getMaxTransactionCount() {
return this.maxTransactionCount;
}
public int getStackTraceLimit() {
return this.stackTraceLimit;
}
public int getResponseBodyLimit() {
return this.responseBodyLimit;
}
public boolean isCollectingNetworkErrors() {
return this.collectingNetworkErrors;
}
public int getErrorLimit() {
return this.errorLimit;
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(this.dataToken);
return sb.toString();
}
}
/* Location: D:\Android_Agent\AndroidInstrumentation\SampleApp_RE_code\NewRelic_Android_Agent_5.8.3\newrelic-android-5.8.3\lib\newrelic.android.jar!\com\newrelic\agent\android\api\common\ConnectionState.class
* Java compiler version: 6 (50.0)
* JD-Core Version: 0.7.1
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy