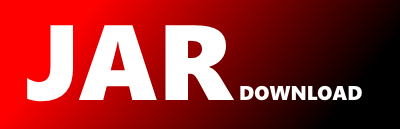
com.eg.agent.android.instrumentation.GsonInstrumentation Maven / Gradle / Ivy
package com.eg.agent.android.instrumentation;
/**
* Created by Venkateswari.J on 10/25/2017.
*/
import com.eg.agent.android.trace.TraceMachine;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonIOException;
import com.google.gson.JsonSyntaxException;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.Reader;
import java.lang.reflect.Type;
public class GsonInstrumentation
{
/*
private static final ArrayList categoryParams = new ArrayList(Arrays.asList(new String[] { "category", MetricCategory.class.getName(), "JSON" }));
*/
public GsonInstrumentation() {
}
private static final String categoryParams = "Gson";
private static final String APItype= "Gson";
@ReplaceCallSite(scope="Gson")
public static String toJson(Gson gson, Object src)
{
// TraceMachine.enterMethod("Gson#toJson", categoryParams);
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
String string = gson.toJson(src);
TraceMachine.exitMethod();
return string;
}
@ReplaceCallSite(scope="Gson")
public static String toJson(Gson gson, Object src, Type typeOfSrc) {
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
String string = gson.toJson(src, typeOfSrc);
TraceMachine.exitMethod();
return string;
}
@ReplaceCallSite(scope="Gson")
public static void toJson(Gson gson, Object src, Appendable writer) throws JsonIOException {
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
gson.toJson(src, writer);
TraceMachine.exitMethod();
}
@ReplaceCallSite(scope="Gson")
public static void toJson(Gson gson, Object src, Type typeOfSrc, Appendable writer) throws JsonIOException {
TraceMachine.enterMethod("Gson#toJson",categoryParams.toString(),APItype);
gson.toJson(src, typeOfSrc, writer);
TraceMachine.exitMethod();
}
@ReplaceCallSite(scope="Gson")
public static void toJson(Gson gson, Object src, Type typeOfSrc, JsonWriter writer) throws JsonIOException {
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
gson.toJson(src, typeOfSrc, writer);
TraceMachine.exitMethod();
}
@ReplaceCallSite(scope="Gson")
public static String toJson(Gson gson, JsonElement jsonElement) {
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
String string = gson.toJson(jsonElement);
TraceMachine.exitMethod();
return string;
}
@ReplaceCallSite(scope="Gson")
public static void toJson(Gson gson, JsonElement jsonElement, Appendable writer) throws JsonIOException {
TraceMachine.enterMethod("Gson#toJson", categoryParams.toString(),APItype);
gson.toJson(jsonElement, writer);
TraceMachine.exitMethod();
}
@ReplaceCallSite(scope="Gson")
public static void toJson(Gson gson, JsonElement jsonElement, JsonWriter writer) throws JsonIOException {
TraceMachine.enterMethod("Gson#toJson",categoryParams.toString(),APItype);
gson.toJson(jsonElement, writer);
TraceMachine.exitMethod();
}
@ReplaceCallSite(scope="Gson")
public static T fromJson(Gson gson, String json, Class classOfT) throws JsonSyntaxException {
TraceMachine.enterMethod("Gson#fromJson",categoryParams.toString(),APItype);
T object = gson.fromJson(json, classOfT);
TraceMachine.exitMethod();
return object;
}
/*
@ReplaceCallSite(scope="Gson")
*/
@ReplaceCallSite(scope="Gson")
public static T fromJson(Gson gson, Reader json, Class classOfT) throws JsonSyntaxException, JsonIOException {
TraceMachine.enterMethod("Gson#fromJson", categoryParams.toString(),APItype);
T object = gson.fromJson(json, classOfT);
TraceMachine.exitMethod();
return object;
}
@ReplaceCallSite(scope="Gson")
public static T fromJson(Gson gson, Reader json, Type typeOfT) throws JsonIOException, JsonSyntaxException {
TraceMachine.enterMethod("Gson#fromJson", categoryParams.toString(),APItype);
T object = gson.fromJson(json, typeOfT);
TraceMachine.exitMethod();
return object;
}
@ReplaceCallSite(scope="Gson")
public static T fromJson(Gson gson, JsonReader reader, Type typeOfT) throws JsonIOException, JsonSyntaxException {
TraceMachine.enterMethod("Gson#fromJson", categoryParams.toString(),APItype);
T object = gson.fromJson(reader, typeOfT);
TraceMachine.exitMethod();
return object;
}
@ReplaceCallSite(scope="Gson")
public static T fromJson(Gson gson, JsonElement json, Class classOfT) throws JsonSyntaxException {
TraceMachine.enterMethod("Gson#fromJson", categoryParams.toString(),APItype);
T object = gson.fromJson(json, classOfT);
TraceMachine.exitMethod();
return object;
}
@ReplaceCallSite(
scope = "Gson"
)
public static T fromJson(Gson gson, String json, Type typeOfT) throws JsonSyntaxException {
TraceMachine.enterMethod("Gson#fromJson", categoryParams,APItype);
T object = gson.fromJson(json, typeOfT);
TraceMachine.exitMethod();
return object;
}
/*
.fromJson(gson, jSONArray, type);
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy