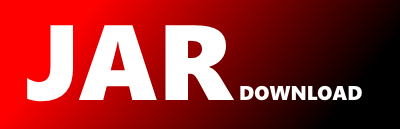
com.eg.agent.android.instrumentation.TransactionState Maven / Gradle / Ivy
package com.eg.agent.android.instrumentation;
import android.util.Log;
import com.eg.agent.android.common.TransactionData;
import com.eg.agent.android.trace.TraceMachine;
import com.eg.agent.android.util.Util;
import com.eg.google.gson.JsonObject;
import com.eg.google.gson.JsonPrimitive;
public final class TransactionState
{
private String url;
private String httpMethod;
private int statusCode;
private int errorCode;
private String errorMessage="null";
private long bytesSent;
private long bytesReceived;
private String carrier;
private String wanType;
public long getStartTime() {
return startTime;
}
public void setStartTime(long startTime) {
this.startTime = startTime;
}
public long getEndTime() {
return endTime;
}
public void setEndTime(long endTime) {
this.endTime = endTime;
}
private long startTime = System.currentTimeMillis();
private long endTime;
private String appData;
private State state;
private String contentType;
private TransactionData transactionData;
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public void setErrorCode(int errorCode) {
this.errorCode = errorCode;
}
public TransactionState()
{
this.state = State.READY;
}
private static TransactionState instance=new TransactionState();
public static TransactionState getInstance()
{
return instance;
}
public void setCarrier(String carrier) {
if (!isSent()) {
this.carrier = carrier;
TraceMachine.setCurrentTraceParam("carrier", carrier);
}
else {
Log.w("eG Agent","setCarrier(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public void setWanType(String wanType) {
if (!isSent()) {
this.wanType = wanType;
TraceMachine.setCurrentTraceParam("wan_type", wanType);
} else {
Log.w("eG Agent","setWanType(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public void setAppData(String appData)
{
if(!this.isComplete())
{
this.appData = appData;
}
else
{
Log.w("eG Agent", "setAppData(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public void setUrl(String url)
{
if(url != null)
{
if(!this.isSent())
{
this.url = url;
}
else {
Log.w("eG Agent", "setUrl(...) called on TransactionState in " + this.state.toString() + " state");
}
}
}
public void setHttpMethod(String httpMethod)
{
if(!this.isSent())
{
this.httpMethod = httpMethod;
}
else
{
Log.w("eG Agent", "setHttpMethod(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public String getUrl()
{
return this.url;
}
public String getHttpMethod()
{
return this.httpMethod;
}
public void setStatusCode(int statusCode)
{
if(!this.isComplete())
{
this.statusCode = statusCode;
}
else
{
Log.w("eG Agent", "setStatusCode(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public int getStatusCode()
{
return this.statusCode;
}
public void setErrorCode(int errorCode, String errorMessage)
{
if(!this.isComplete())
{
this.errorCode = errorCode;
this.errorMessage=errorMessage;
}
else
{
if(this.transactionData != null) {
this.transactionData.setErrorCode(errorCode);
this.transactionData.setErrorMessage(errorMessage);
this.setErrorMessage(errorMessage);
}
Log.w("eG Agent", "setErrorCode(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public int getErrorCode()
{
return this.errorCode;
}
public void setBytesSent(long bytesSent)
{
if(!this.isComplete())
{
this.bytesSent = bytesSent;
this.state = State.SENT;
}
else
{
Log.w("eG Agent", "setBytesSent(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public void setBytesReceived(long bytesReceived)
{
if(!this.isComplete())
{
this.bytesReceived = bytesReceived;
} else {
Log.w("eG Agent", "setBytesReceived(...) called on TransactionState in " + this.state.toString() + " state");
}
}
public long getBytesReceived()
{
return this.bytesReceived;
}
public long getBytesSent()
{
return this.bytesSent;
}
public JsonObject asJsonObject() {
JsonObject data = new JsonObject();
try{
data.add("url",new JsonPrimitive(this.url));
data.add("httpMethod", new JsonPrimitive(this.httpMethod));
data.add("statusCode",new JsonPrimitive(this.statusCode));
data.add("bytesSent",new JsonPrimitive(this.bytesSent));
data.add("bytesReceived",new JsonPrimitive(this.bytesReceived));
data.add("startTime",new JsonPrimitive(this.startTime));
data.add("endTime",new JsonPrimitive(this.endTime));
data.add("errorCode",new JsonPrimitive(this.errorCode));
data.add("errorMessage",new JsonPrimitive(this.errorMessage));
System.out.println("data json "+data.toString());
}
catch(Exception e){
Log.e("eG TransactionState#JSONASONJIECT",e.getMessage());
}
return data;
}
public static TransactionState newFromJson(JsonObject jsonObject)
{
TransactionState info = new TransactionState();
info.url = jsonObject.get("url").getAsString();
info.httpMethod = jsonObject.get("httpMethod").getAsString();
info.statusCode = jsonObject.get("statusCode").getAsInt();
info.errorCode = jsonObject.get("errorCode").getAsInt();
info.errorMessage = jsonObject.get("errorMessage").getAsString();
info.bytesSent = jsonObject.get("bytesSent").getAsLong();
info.bytesReceived = jsonObject.get("bytesReceived").getAsLong();
info.startTime = jsonObject.get("startTime").getAsLong();
info.endTime = jsonObject.get("endTime").getAsLong();
info.appData = jsonObject.get("appData").getAsString();
System.out.println("data info "+info.toString());
return info;
}
public TransactionData end()
{
if(!this.isComplete())
{
this.state = State.COMPLETE;
this.endTime = System.currentTimeMillis();
}
return this.toTransactionData();
}
private TransactionData toTransactionData()
{
if(!this.isComplete())
{
Log.w("eG Agent", "toTransactionData() called on incomplete TransactionState");
}
if(this.url == null)
{
Log.w("eG Agent", "Attempted to convert a TransactionState instance with no URL into a TransactionData");
return null;
}
else
{
if(this.transactionData == null)
{
this.transactionData = new TransactionData(this.url, this.httpMethod, (float)(this.endTime - this.startTime) / 1000.0F, this.statusCode, this.errorCode, this.bytesSent, this.bytesReceived, this.appData);
}
return this.transactionData;
}
}
public String toString() {
return "url=\'" + Util.encodeUri(this.url) + '\'' + ", httpMethod=\'" + this.httpMethod + '\'' + ", statusCode=" + this.statusCode + ", errorCode=" + this.errorCode + ", bytesSent=" + this.bytesSent + ", bytesReceived=" + this.bytesReceived + ", startTime=" + this.startTime + ", endTime=" + this.endTime + ",contentType=\'" + this.contentType + '\'' + ", errorMessage=\'" + this.errorMessage + '}';
}
public boolean isSent()
{
return this.state.ordinal() >= State.SENT.ordinal();
}
public boolean isComplete()
{
return this.state.ordinal() >= State.COMPLETE.ordinal();
}
private static enum State
{
READY,
SENT,
COMPLETE;
private State()
{
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy