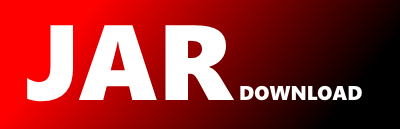
com.eg.agent.android.instrumentation.io.CountingInputStream Maven / Gradle / Ivy
package com.eg.agent.android.instrumentation.io;
import java.io.IOException;
import java.io.InputStream;
import java.nio.ByteBuffer;
public final class CountingInputStream extends InputStream implements StreamCompleteListenerSource
{
private ByteBuffer buffer;
private long count = 0L;
private boolean enableBuffering = false;
private final InputStream impl;
private final StreamCompleteListenerManager listenerManager = new StreamCompleteListenerManager();
public CountingInputStream(InputStream paramInputStream)
{
this.impl = paramInputStream;
if (this.enableBuffering)
{
//this.buffer = ByteBuffer.allocate(Agent.getResponseBodyLimit());
fillBuffer();
return;
}
this.buffer = null;
}
public CountingInputStream(InputStream paramInputStream, boolean paramBoolean)
{
this.impl = paramInputStream;
this.enableBuffering = paramBoolean;
if (paramBoolean)
{
//this.buffer = ByteBuffer.allocate(Agent.getResponseBodyLimit());
fillBuffer();
return;
}
this.buffer = null;
}
private boolean bufferEmpty()
{
return !this.buffer.hasRemaining();
}
private boolean bufferHasBytes(long paramLong)
{
return this.buffer.remaining() >= paramLong;
}
private void notifyStreamComplete()
{
if (!this.listenerManager.isComplete())
{
this.listenerManager.notifyStreamComplete(new StreamCompleteEvent(this, this.count));
}
}
private void notifyStreamError(Exception paramException)
{
if (!this.listenerManager.isComplete())
{
this.listenerManager.notifyStreamError(new StreamCompleteEvent(this, this.count, paramException));
}
}
private int readBuffer()
{
if (bufferEmpty())
{
return -1;
}
return this.buffer.get();
}
private int readBufferBytes(byte[] paramArrayOfByte)
{
return readBufferBytes(paramArrayOfByte, 0, paramArrayOfByte.length);
}
private int readBufferBytes(byte[] paramArrayOfByte, int paramInt1, int paramInt2)
{
if (bufferEmpty())
{
return -1;
}
int i = this.buffer.remaining();
this.buffer.get(paramArrayOfByte, paramInt1, paramInt2);
return i - this.buffer.remaining();
}
public void addStreamCompleteListener(StreamCompleteListener paramStreamCompleteListener)
{
this.listenerManager.addStreamCompleteListener(paramStreamCompleteListener);
}
public int available() throws IOException
{
int i = 0;
if (this.enableBuffering)
{
i = this.buffer.remaining();
}
try
{
int j = this.impl.available();
return j + i;
} catch (IOException localIOException)
{
notifyStreamError(localIOException);
throw localIOException;
}
}
public void close() throws IOException
{
try
{
this.impl.close();
notifyStreamComplete();
return;
}
catch (IOException localIOException)
{
notifyStreamError(localIOException);
throw localIOException;
}
}
public void fillBuffer() {
}
public String getBufferAsString()
{
Object localObject1 = new byte[this.buffer.limit()];
int i = 0;
localObject1 = new String((byte[]) localObject1);
return (String) localObject1;
}
public void mark(int paramInt)
{
if (!markSupported())
{
return;
}
this.impl.mark(paramInt);
}
public boolean markSupported()
{
return this.impl.markSupported();
}
public int read() throws IOException
{
return 0;
}
public int read(byte[] paramArrayOfByte) throws IOException
{
int j = 0;
int m = 0;
int k = paramArrayOfByte.length;
int i = k;
ByteBuffer localByteBuffer;
if (this.enableBuffering)
{
localByteBuffer = this.buffer;
long l = k;
try
{
if (bufferHasBytes(l))
{
i = readBufferBytes(paramArrayOfByte);
if (i >= 0)
{
this.count += i;
return i;
}
throw new IOException("readBufferBytes failed");
}
}
finally
{
}
int n = this.buffer.remaining();
i = k;
j = m;
if (n > 0)
{
j = readBufferBytes(paramArrayOfByte, 0, n);
if (j < 0)
{
throw new IOException("partial read from buffer failed");
}
i = k - j;
this.count += j;
}
}
i = this.impl.read(paramArrayOfByte, j, i);
if (i >= 0)
{
this.count += i;
return i + j;
}
if (j <= 0)
{
notifyStreamComplete();
return i;
}
return j;
}
public int read(byte[] paramArrayOfByte, int paramInt1, int paramInt2) throws IOException
{
int j = 0;
int m = 0;
int i = paramInt2;
int k = i;
if (this.enableBuffering)
{
ByteBuffer localByteBuffer = this.buffer;
long l = i;
try
{
if (bufferHasBytes(l))
{
paramInt1 = readBufferBytes(paramArrayOfByte, paramInt1, paramInt2);
if (paramInt1 >= 0)
{
this.count += paramInt1;
return paramInt1;
}
throw new IOException("readBufferBytes failed");
}
}
finally
{
}
k = this.buffer.remaining();
paramInt2 = i;
j = m;
if (k > 0)
{
j = readBufferBytes(paramArrayOfByte, paramInt1, k);
if (j < 0)
{
throw new IOException("partial read from buffer failed");
}
paramInt2 = i - j;
this.count += j;
}
k = paramInt2;
}
paramInt1 = this.impl.read(paramArrayOfByte, paramInt1 + j, k);
if (paramInt1 >= 0)
{
this.count += paramInt1;
return paramInt1 + j;
}
if (j <= 0)
{
notifyStreamComplete();
return paramInt1;
}
return j;
}
public void removeStreamCompleteListener(StreamCompleteListener paramStreamCompleteListener)
{
this.listenerManager.removeStreamCompleteListener(paramStreamCompleteListener);
}
public void reset() throws IOException
{
if (!markSupported())
{
return;
}
try
{
this.impl.reset();
return;
}
catch (IOException localIOException)
{
notifyStreamError(localIOException);
throw localIOException;
}
}
public void setBufferingEnabled(boolean paramBoolean)
{
this.enableBuffering = paramBoolean;
}
public long skip(long paramLong) throws IOException
{
long l = paramLong;
if (this.enableBuffering)
{
}
synchronized (this.buffer)
{
if (bufferHasBytes(paramLong))
{
this.buffer.position((int) paramLong);
this.count += paramLong;
return paramLong;
}
l = paramLong - this.buffer.remaining();
if (l > 0L)
{
this.buffer.position(this.buffer.remaining());
}
try
{
paramLong = this.impl.skip(l);
this.count += paramLong;
return paramLong;
}
catch (IOException localIOException)
{
notifyStreamError(localIOException);
throw localIOException;
}
//throw new IOException("partial read from buffer (skip) failed");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy