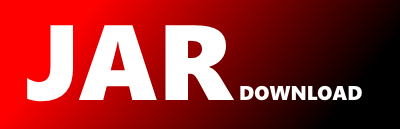
com.eg.agent.android.metric.MetricStore Maven / Gradle / Ivy
package com.eg.agent.android.metric;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.concurrent.ConcurrentHashMap;
public class MetricStore
{
private final Map> metricStore;
public MetricStore()
{
this.metricStore = new ConcurrentHashMap();
}
public void add(Metric metric) {
String scope = metric.getStringScope();
String name = metric.getName();
if (!this.metricStore.containsKey(scope)) {
this.metricStore.put(scope, new HashMap());
}
if (((Map) this.metricStore.get(scope)).containsKey(name)) {
((Metric) ((Map) this.metricStore.get(scope)).get(name)).aggregate(metric);
} else {
((Map) this.metricStore.get(scope)).put(name, metric);
}
}
public Metric get(String name) {
return get(name, "");
}
public Metric get(String name, String scope) {
try {
return (Metric)((Map)this.metricStore.get(scope == null ? "" : scope)).get(name);
} catch (NullPointerException e) {}
return null;
}
public List getAll() {
/* List metrics = new ArrayList();
for (Entry> entry : this.metricStore.entrySet()) {
for (Entry metricEntry : ((Map) entry.getValue()).entrySet()) {
metrics.add(metricEntry.getValue());
}
}
return metrics;*/
ArrayList metrics = new ArrayList();
Iterator var2 = this.metricStore.entrySet().iterator();
while(var2.hasNext()) {
Entry entry = (Entry)var2.next();
Iterator var4 = ((Map)entry.getValue()).entrySet().iterator();
while(var4.hasNext()) {
Entry metricEntry = (Entry)var4.next();
metrics.add(metricEntry.getValue());
}
}
return metrics;
}
public List getAllByScope(String scope) {
/* List metrics = new ArrayList();
try {
for (Entry metricEntry : ((Map) this.metricStore.get(scope)).entrySet()) {
metrics.add(metricEntry.getValue());
}
} catch (NullPointerException e) {
}
return metrics;*/
ArrayList metrics = new ArrayList();
try {
Iterator var3 = ((Map)this.metricStore.get(scope)).entrySet().iterator();
while(var3.hasNext()) {
Entry metricEntry = (Entry)var3.next();
metrics.add(metricEntry.getValue());
}
} catch (NullPointerException var5) {
;
}
return metrics;
}
public List getAllUnscoped() {
return getAllByScope("");
}
public void remove(Metric metric) {
String scope = metric.getStringScope();
String name = metric.getName();
if (!this.metricStore.containsKey(scope)) {
return;
}
if (!((Map) this.metricStore.get(scope)).containsKey(name)) {
return;
}
((Map) this.metricStore.get(scope)).remove(name);
}
public void removeAll(List metrics) {
synchronized (this.metricStore) {
for (Metric metric : metrics) {
remove(metric);
}
}
}
public List removeAllWithScope(String scope) {
List metrics = getAllByScope(scope);
if (!metrics.isEmpty()) {
removeAll(metrics);
}
return metrics;
}
public void clear() {
this.metricStore.clear();
}
public boolean isEmpty() {
/* 111 */ return this.metricStore.isEmpty();
}
}
/* Location: D:\Android_Agent\AndroidInstrumentation\SampleApp_RE_code\NewRelic_Android_Agent_5.8.3\newrelic-android-5.8.3\lib\newrelic.android.jar!\com\newrelic\agent\android\metric\MetricStore.class
* Java compiler version: 6 (50.0)
* JD-Core Version: 0.7.1
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy