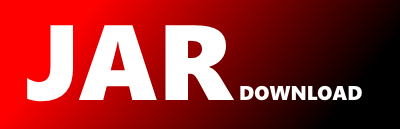
com.eg.agent.android.trace.Trace Maven / Gradle / Ivy
package com.eg.agent.android.trace;
import android.util.Log;
import com.eg.agent.android.instrumentation.MetricCategory;
import com.eg.agent.android.util.Util;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import java.util.concurrent.ConcurrentHashMap;
public class Trace {
private static final String CATEGORY_PARAMETER = "category";
//private static final AgentLog log = ;
public final UUID parentUUID;
public final UUID myUUID = new UUID(Util.getRandom().nextLong(), Util.getRandom().nextLong());
public long entryTimestamp = 0L;
public long exitTimestamp = 0L;
public long exclusiveTime = 0L;
public long childExclusiveTime = 0L;
public String metricName;
public String metricBackgroundName;
public String displayName;
public String scope;
public long threadId = 0L;
public String threadName = "main";
private volatile Map params;
private List rawAnnotationParams;
private volatile Set children;
private TraceType type = TraceType.TRACE;
private boolean isComplete = false;
public TraceMachine traceMachine;
public static String ActiviteClassName;
public Trace() {
this.parentUUID = null;
}
public Trace(String displayName, UUID parentUUID, TraceMachine traceMachine) {
this.displayName = displayName;
this.parentUUID = parentUUID;
this.traceMachine = traceMachine;
}
public void setActiviteClassName(String className) {
ActiviteClassName = className;
}
public static String getActiviteClassName() {
return ActiviteClassName;
}
public void addChild(Trace trace) {
if (this.children == null) {
synchronized (this) {
if (this.children == null) {
this.children = Collections.synchronizedSet(new HashSet());
}
}
}
this.children.add(trace.myUUID);
}
public Set getChildren() {
if (this.children == null) {
synchronized (this) {
if (this.children == null) {
this.children = Collections.synchronizedSet(new HashSet());
}
}
}
return this.children;
}
public Map getParams() {
if (this.params == null) {
synchronized (this) {
if (this.params == null) {
this.params = new ConcurrentHashMap();
}
}
}
return this.params;
}
public void setAnnotationParams(List rawAnnotationParams) {
this.rawAnnotationParams = rawAnnotationParams;
}
public Map getAnnotationParams() {
HashMap annotationParams = new HashMap();
if ((this.rawAnnotationParams != null) && (this.rawAnnotationParams.size() > 0)) {
Iterator i = this.rawAnnotationParams.iterator();
while (i.hasNext()) {
String paramName = (String) i.next();
String paramClass = (String) i.next();
String paramValue = (String) i.next();
Object param = createParameter(paramName, paramClass, paramValue);
if (param != null) {
annotationParams.put(paramName, param);
}
}
}
return annotationParams;
}
public boolean isComplete() {
return this.isComplete;
}
public void complete() throws Exception {
if (this.isComplete) {
Log.e("Attempted to double complete trace ", this.myUUID.toString());
return;
}
if (this.exitTimestamp == 0L) {
this.exitTimestamp = System.currentTimeMillis();
}
this.exclusiveTime = (getDurationAsMilliseconds() - this.childExclusiveTime);
this.isComplete = true;
try {
/*
this.traceMachine.storeCompletedTrace(this);
*/
} catch (NullPointerException e) {
throw new Exception();
}
}
public void prepareForSerialization() {
getParams().put("type", this.type.toString());
}
public TraceType getType() {
return this.type;
}
public void setType(TraceType type) {
this.type = type;
}
public long getDurationAsMilliseconds() {
return this.exitTimestamp - this.entryTimestamp;
}
public float getDurationAsSeconds() {
return (float) (this.exitTimestamp - this.entryTimestamp) / 1000.0F;
}
public MetricCategory getCategory() {
if (!getAnnotationParams().containsKey("category")) {
return null;
}
Object category = getAnnotationParams().get("category");
if (!(category instanceof MetricCategory)) {
Log.e("eG", "Category annotation parameter is not of type MetricCategory");
return null;
}
return (MetricCategory) category;
}
private static Object createParameter(String parameterName, String parameterClass, String parameterValue) {
Class clazz;
try {
clazz = Class.forName(parameterClass);
} catch (ClassNotFoundException e) {
Log.e("Unable to resolve parameter class in enterMethod: ", e.getMessage(), e);
return null;
}
if (MetricCategory.class == clazz) {
return MetricCategory.valueOf(parameterValue);
}
if (String.class == clazz) {
return parameterValue;
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy