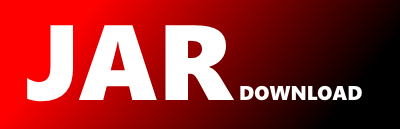
com.eg.google.gson.JsonPrimitive Maven / Gradle / Ivy
package com.eg.google.gson;
import com.eg.google.gson.internal.$Gson$Preconditions;
import com.eg.google.gson.internal.LazilyParsedNumber;
import java.math.BigDecimal;
import java.math.BigInteger;
import static java.lang.Boolean.parseBoolean;
import static java.lang.Byte.parseByte;
import static java.lang.Double.doubleToLongBits;
import static java.lang.Double.isNaN;
import static java.lang.Double.parseDouble;
import static java.lang.Integer.parseInt;
import static java.lang.Long.parseLong;
import static java.lang.Short.parseShort;
import static java.lang.String.valueOf;
public final class JsonPrimitive
extends JsonElement
{
private static final Class>[] PRIMITIVE_TYPES = { Integer.TYPE, Long.TYPE, Short.TYPE, Float.TYPE, Double.TYPE, Byte.TYPE, Boolean.TYPE, Character.TYPE, Integer.class, Long.class, Short.class, Float.class, Double.class, Byte.class, Boolean.class, Character.class };
private Object value;
public JsonPrimitive(Boolean bool)
{
setValue(bool);
}
public JsonPrimitive(Number number) {
setValue(number);
}
public JsonPrimitive(String string)
{
setValue(string);
}
public JsonPrimitive(Character c)
{
setValue(c);
}
JsonPrimitive(Object primitive) {
setValue(primitive);
}
JsonPrimitive deepCopy()
{
return this;
}
void setValue(Object primitive) {
if ((primitive instanceof Character)) {
char c = ((Character) primitive).charValue();
this.value = valueOf(c);
} else {
$Gson$Preconditions.checkArgument(((primitive instanceof Number)) || (isPrimitiveOrString(primitive)));
this.value = primitive;
}
}
public boolean isBoolean() {
return this.value instanceof Boolean;
}
Boolean getAsBooleanWrapper() {
return (Boolean) this.value;
}
public boolean getAsBoolean() {
if (isBoolean()) {
return getAsBooleanWrapper().booleanValue();
}
return parseBoolean(getAsString());
}
public boolean isNumber() {
return this.value instanceof Number;
}
public Number getAsNumber() {
return (this.value instanceof String) ? new LazilyParsedNumber((String) this.value) : (Number) this.value;
}
public boolean isString() {
return this.value instanceof String;
}
public String getAsString() {
if (isNumber())
return getAsNumber().toString();
if (isBoolean()) {
return getAsBooleanWrapper().toString();
}
return (String) this.value;
}
public double getAsDouble() {
return isNumber() ? getAsNumber().doubleValue() : parseDouble(getAsString());
}
public BigDecimal getAsBigDecimal() {
return (this.value instanceof BigDecimal) ? (BigDecimal) this.value : new BigDecimal(this.value.toString());
}
public BigInteger getAsBigInteger() {
return (this.value instanceof BigInteger) ? (BigInteger) this.value : new BigInteger(this.value.toString());
}
public float getAsFloat()
{
/* 227 */ return isNumber() ? getAsNumber().floatValue() : Float.parseFloat(getAsString());
}
public long getAsLong() {
return isNumber() ? getAsNumber().longValue() : parseLong(getAsString());
}
public short getAsShort() {
return isNumber() ? getAsNumber().shortValue() : parseShort(getAsString());
}
public int getAsInt() {
return isNumber() ? getAsNumber().intValue() : parseInt(getAsString());
}
public byte getAsByte() {
return isNumber() ? getAsNumber().byteValue() : parseByte(getAsString());
}
public char getAsCharacter()
{
/* 270 */ return getAsString().charAt(0);
}
private static boolean isPrimitiveOrString(Object target) {
if ((target instanceof String)) {
return true;
}
Class> classOfPrimitive = target.getClass();
for (Class> standardPrimitive : PRIMITIVE_TYPES) {
if (standardPrimitive.isAssignableFrom(classOfPrimitive)) {
/* 281 */
return true;
}
}
return false;
}
public int hashCode() {
if (this.value == null) {
return 31;
}
/* 293 */
if (isIntegral(this)) {
long value = getAsNumber().longValue();
return (int) (value ^ value >>> 32);
}
/* 297 */
if ((this.value instanceof Number)) {
/* 298 */
long value = doubleToLongBits(getAsNumber().doubleValue());
return (int) (value ^ value >>> 32);
}
return this.value.hashCode();
}
public boolean equals(Object obj) {
if (this == obj) {
/* 307 */
return true;
}
if ((obj == null) || (getClass() != obj.getClass())) {
return false;
}
JsonPrimitive other = (JsonPrimitive) obj;
/* 313 */
if (this.value == null) {
/* 314 */
return other.value == null;
}
if ((isIntegral(this)) && (isIntegral(other))) {
return getAsNumber().longValue() == other.getAsNumber().longValue();
}
if (((this.value instanceof Number)) && ((other.value instanceof Number))) {
double a = getAsNumber().doubleValue();
double b = other.getAsNumber().doubleValue();
return (a == b) || ((isNaN(a)) && (isNaN(b)));
}
/* 326 */
return this.value.equals(other.value);
}
private static boolean isIntegral(JsonPrimitive primitive) {
if ((primitive.value instanceof Number)) {
/* 335 */
Number number = (Number) primitive.value;
/* 336 */
return ((number instanceof BigInteger)) || ((number instanceof Long)) || ((number instanceof Integer)) || ((number instanceof Short)) || ((number instanceof Byte));
}
return false;
}
}
/* Location: D:\Android_Agent\AndroidInstrumentation\SampleApp_RE_code\NewRelic_Android_Agent_5.8.3\newrelic-android-5.8.3\lib\newrelic.android.jar!\com.google.gson\JsonPrimitive.class
* Java compiler version: 5 (49.0)
* JD-Core Version: 0.7.1
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy