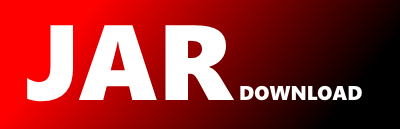
com.eg.google.gson.internal.UnsafeAllocator Maven / Gradle / Ivy
package com.eg.google.gson.internal;
import java.io.ObjectInputStream;
import java.io.ObjectStreamClass;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import static java.lang.Class.forName;
/*public abstract class UnsafeAllocator
{
public abstract T newInstance(Class paramClass)
throws Exception;
public static UnsafeAllocator create()
{
try {
Class> unsafeClass = forName("sun.misc.Unsafe");
*//*
Field f = unsafeClass.getDeclaredField("theUnsafe");
*//*
f.setAccessible(true);
final Object unsafe = f.get(null);
Method allocateInstance = unsafeClass.getMethod("allocateInstance", new Class[]{Class.class});
new UnsafeAllocator() {
public T newInstance(Class c) throws Exception {
return (T) allocateInstance.invoke(unsafe, c);
}
};
}
catch (Exception ignored)
{
try {
Method newInstance = ObjectInputStream.class.getDeclaredMethod("newInstance", new Class[]{Class.class, Class.class});
*//* 62 *//*
newInstance.setAccessible(true);
new UnsafeAllocator() {
public T newInstance(Class c) throws Exception {
return (T) this.val$newInstance.invoke(null, new Object[]{c, Object.class});
}
};
}
catch (Exception ignored)
{
try {
*//* 79 *//*
Method getConstructorId = ObjectStreamClass.class.getDeclaredMethod("getConstructorId", new Class[]{Class.class});
getConstructorId.setAccessible(true);
final int constructorId = ((Integer) getConstructorId.invoke(null, new Object[]{Object.class})).intValue();
*//* 83 *//*
Method newInstance = ObjectStreamClass.class.getDeclaredMethod("newInstance", new Class[]{Class.class, TYPE});
*//* 85 *//*
newInstance.setAccessible(true);
new UnsafeAllocator() {
public T newInstance(Class c) throws Exception {
return (T) this.val$newInstance.invoke(null, new Object[]{c, valueOf(constructorId)});
}
};
}
catch (Exception ignored) {}
}
}
*//* 97 *//* new UnsafeAllocator()
{
public T newInstance(Class c) {
*//* :0 *//* throw new UnsupportedOperationException("Cannot allocate " + c);
}
};
}
}
*/public abstract class UnsafeAllocator {
public abstract T newInstance(Class c) throws Exception;
public static UnsafeAllocator create() {
// try JVM
// public class Unsafe {
// public Object allocateInstance(Class> type);
// }
try {
Class> unsafeClass = forName("sun.misc.Unsafe");
Field f = unsafeClass.getDeclaredField("theUnsafe");
f.setAccessible(true);
final Object unsafe = f.get(null);
final Method allocateInstance = unsafeClass.getMethod("allocateInstance", Class.class);
return new UnsafeAllocator() {
@Override
@SuppressWarnings("unchecked")
public T newInstance(Class c) throws Exception {
return (T) allocateInstance.invoke(unsafe, c);
}
};
} catch (Exception ignored) {
}
// try dalvikvm, post-gingerbread
// public class ObjectStreamClass {
// private static native int getConstructorId(Class> c);
// private static native Object newInstance(Class> instantiationClass, int methodId);
// }
try {
Method getConstructorId = ObjectStreamClass.class
.getDeclaredMethod("getConstructorId", Class.class);
getConstructorId.setAccessible(true);
final int constructorId = (Integer) getConstructorId.invoke(null, Object.class);
final Method newInstance = ObjectStreamClass.class
.getDeclaredMethod("newInstance", Class.class, int.class);
newInstance.setAccessible(true);
return new UnsafeAllocator() {
@Override
@SuppressWarnings("unchecked")
public T newInstance(Class c) throws Exception {
return (T) newInstance.invoke(null, c, constructorId);
}
};
} catch (Exception ignored) {
}
// try dalvikvm, pre-gingerbread
// public class ObjectInputStream {
// private static native Object newInstance(
// Class> instantiationClass, Class> constructorClass);
// }
try {
final Method newInstance = ObjectInputStream.class
.getDeclaredMethod("newInstance", Class.class, Class.class);
newInstance.setAccessible(true);
return new UnsafeAllocator() {
@Override
@SuppressWarnings("unchecked")
public T newInstance(Class c) throws Exception {
return (T) newInstance.invoke(null, c, Object.class);
}
};
} catch (Exception ignored) {
}
// give up
return new UnsafeAllocator() {
@Override
public T newInstance(Class c) {
throw new UnsupportedOperationException("Cannot allocate " + c);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy