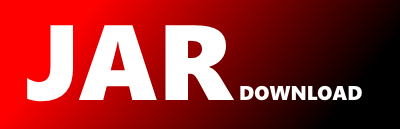
com.eg.google.gson.internal.bind.JsonTreeWriter Maven / Gradle / Ivy
package com.eg.google.gson.internal.bind;
import com.eg.google.gson.JsonArray;
import com.eg.google.gson.JsonElement;
import com.eg.google.gson.JsonNull;
import com.eg.google.gson.JsonObject;
import com.eg.google.gson.JsonPrimitive;
import com.eg.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.io.Writer;
import java.util.ArrayList;
import java.util.List;
import static java.lang.Double.isInfinite;
import static java.lang.Double.isNaN;
import static java.lang.Long.valueOf;
public final class JsonTreeWriter
extends JsonWriter
{
private static final Writer UNWRITABLE_WRITER = new Writer() {
public void write(char[] buffer, int offset, int counter) {
throw new AssertionError();
}
public void flush() throws IOException {
throw new AssertionError();
}
public void close() throws IOException {
throw new AssertionError();
}
};
private static final JsonPrimitive SENTINEL_CLOSED = new JsonPrimitive("closed");
private final List stack = new ArrayList();
private String pendingName;
private JsonElement product = JsonNull.INSTANCE;
public JsonTreeWriter() {
super(UNWRITABLE_WRITER);
}
public JsonElement get()
{
if (!this.stack.isEmpty()) {
throw new IllegalStateException("Expected one JSON element but was " + this.stack);
}
return this.product;
}
private JsonElement peek() {
return (JsonElement)this.stack.get(this.stack.size() - 1);
}
private void put(JsonElement value) {
if (this.pendingName != null) {
if ((!value.isJsonNull()) || (getSerializeNulls())) {
JsonObject object = (JsonObject) peek();
object.add(this.pendingName, value);
}
this.pendingName = null;
} else if (this.stack.isEmpty()) {
this.product = value;
} else {
JsonElement element = peek();
if ((element instanceof JsonArray)) {
((JsonArray) element).add(value);
} else {
throw new IllegalStateException();
}
}
}
public JsonWriter beginArray() throws IOException {
JsonArray array = new JsonArray();
put(array);
this.stack.add(array);
return this;
}
public JsonWriter endArray() throws IOException {
if ((this.stack.isEmpty()) || (this.pendingName != null)) {
throw new IllegalStateException();
}
JsonElement element = peek();
if ((element instanceof JsonArray)) {
this.stack.remove(this.stack.size() - 1);
return this;
}
/* 110 */
throw new IllegalStateException();
}
public JsonWriter beginObject() throws IOException {
JsonObject object = new JsonObject();
put(object);
this.stack.add(object);
return this;
}
public JsonWriter endObject() throws IOException {
if ((this.stack.isEmpty()) || (this.pendingName != null)) {
throw new IllegalStateException();
}
JsonElement element = peek();
if ((element instanceof JsonObject)) {
this.stack.remove(this.stack.size() - 1);
/* 127 */
return this;
}
throw new IllegalStateException();
}
public JsonWriter name(String name) throws IOException {
if ((this.stack.isEmpty()) || (this.pendingName != null)) {
throw new IllegalStateException();
}
JsonElement element = peek();
if ((element instanceof JsonObject)) {
this.pendingName = name;
return this;
}
throw new IllegalStateException();
}
public JsonWriter value(String value) throws IOException {
if (value == null) {
return nullValue();
}
put(new JsonPrimitive(value));
return this;
}
public JsonWriter nullValue() throws IOException {
put(JsonNull.INSTANCE);
return this;
}
public JsonWriter value(boolean value) throws IOException {
put(new JsonPrimitive(Boolean.valueOf(value)));
return this;
}
public JsonWriter value(double value) throws IOException {
if ((!isLenient()) && ((isNaN(value)) || (isInfinite(value)))) {
throw new IllegalArgumentException("JSON forbids NaN and infinities: " + value);
}
put(new JsonPrimitive(Double.valueOf(value)));
return this;
}
public JsonWriter value(long value) throws IOException {
put(new JsonPrimitive(valueOf(value)));
return this;
}
public JsonWriter value(Number value) throws IOException {
if (value == null) {
return nullValue();
}
if (!isLenient()) {
double d = value.doubleValue();
if ((isNaN(d)) || (isInfinite(d))) {
throw new IllegalArgumentException("JSON forbids NaN and infinities: " + value);
}
}
put(new JsonPrimitive(value));
return this;
}
public void flush() throws IOException
{}
public void close() throws IOException {
if (!this.stack.isEmpty()) {
throw new IOException("Incomplete document");
}
this.stack.add(SENTINEL_CLOSED);
}
}
/* Location: D:\Android_Agent\AndroidInstrumentation\SampleApp_RE_code\NewRelic_Android_Agent_5.8.3\newrelic-android-5.8.3\lib\newrelic.android.jar!\com.google.gson\internal\bind\JsonTreeWriter.class
* Java compiler version: 5 (49.0)
* JD-Core Version: 0.7.1
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy