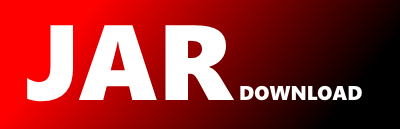
com.ejlchina.okhttps.internal.TaskExecutor Maven / Gradle / Ivy
package com.ejlchina.okhttps.internal;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import com.ejlchina.okhttps.*;
import com.ejlchina.okhttps.HttpResult.State;
public final class TaskExecutor {
private Executor ioExecutor;
private Executor mainExecutor;
private DownListener downloadListener;
private TaskListener responseListener;
private TaskListener exceptionListener;
private TaskListener completeListener;
private MsgConvertor[] msgConvertors;
public TaskExecutor(Executor ioExecutor, Executor mainExecutor, DownListener downloadListener,
TaskListener responseListener, TaskListener exceptionListener,
TaskListener completeListener, MsgConvertor[] msgConvertors) {
this.ioExecutor = ioExecutor;
this.mainExecutor = mainExecutor;
this.downloadListener = downloadListener;
this.responseListener = responseListener;
this.exceptionListener = exceptionListener;
this.completeListener = completeListener;
this.msgConvertors = msgConvertors;
}
public Executor getExecutor(boolean onIo) {
if (onIo || mainExecutor == null) {
return ioExecutor;
}
return mainExecutor;
}
public Download download(HttpTask> httpTask, File file, InputStream input, long skipBytes) {
Download download = new Download(file, input, this, skipBytes);
if (httpTask != null && downloadListener != null) {
downloadListener.listen(httpTask, download);
}
return download;
}
public void execute(Runnable command, boolean onIo) {
Executor executor = ioExecutor;
if (mainExecutor != null && !onIo) {
executor = mainExecutor;
}
executor.execute(command);
}
public void executeOnResponse(HttpTask> task, OnCallback onResponse, HttpResult result, boolean onIo) {
if (responseListener != null) {
if (responseListener.listen(task, result) && onResponse != null) {
execute(() -> onResponse.on(result), onIo);
}
} else if (onResponse != null) {
execute(() -> onResponse.on(result), onIo);
}
}
public boolean executeOnException(HttpTask> task, OnCallback onException, IOException error, boolean onIo) {
if (exceptionListener != null) {
if (exceptionListener.listen(task, error) && onException != null) {
execute(() -> onException.on(error), onIo);
}
} else if (onException != null) {
execute(() -> onException.on(error), onIo);
} else {
return false;
}
return true;
}
public void executeOnComplete(HttpTask> task, OnCallback onComplete, State state, boolean onIo) {
if (completeListener != null) {
if (completeListener.listen(task, state) && onComplete != null) {
execute(() -> onComplete.on(state), onIo);
}
} else if (onComplete != null) {
execute(() -> onComplete.on(state), onIo);
}
}
public interface ConvertFunc {
T apply(MsgConvertor convertor);
}
public static class Data {
public T data;
public String mediaType;
public Data(T data, String mediaType) {
this.data = data;
this.mediaType = mediaType;
}
}
public V doMsgConvert(ConvertFunc callable) {
return doMsgConvert(null, callable).data;
}
public Data doMsgConvert(String type, ConvertFunc callable) {
Throwable cause = null;
for (int i = msgConvertors.length - 1; i >= 0; i--) {
MsgConvertor convertor = msgConvertors[i];
String mediaType = convertor.mediaType();
if (type != null && (mediaType == null || !mediaType.contains(type))) {
continue;
}
if (callable == null && mediaType != null) {
return new Data<>(null, mediaType);
}
try {
assert callable != null;
return new Data<>(callable.apply(convertor), mediaType);
} catch (Exception e) {
if (cause != null) {
initRootCause(e, cause);
}
cause = e;
}
}
if (callable == null) {
return new Data<>(null, "application/x-www-form-urlencoded");
}
if (cause != null) {
throw new HttpException("转换失败", cause);
}
throw new HttpException("没有匹配[" + type + "]类型的转换器!");
}
private void initRootCause(Throwable throwable, Throwable cause) {
Throwable lastCause = throwable.getCause();
if (lastCause != null) {
initRootCause(lastCause, cause);
} else {
throwable.initCause(cause);
}
}
/**
* 关闭线程池
* @since OkHttps V1.0.2
*/
public void shutdown() {
if (ioExecutor != null && ioExecutor instanceof ExecutorService) {
((ExecutorService) ioExecutor).shutdown();
}
if (mainExecutor != null && mainExecutor instanceof ExecutorService) {
((ExecutorService) mainExecutor).shutdown();
}
}
public Executor getIoExecutor() {
return ioExecutor;
}
public Executor getMainExecutor() {
return mainExecutor;
}
public DownListener getDownloadListener() {
return downloadListener;
}
public TaskListener getResponseListener() {
return responseListener;
}
public TaskListener getExceptionListener() {
return exceptionListener;
}
public TaskListener getCompleteListener() {
return completeListener;
}
public MsgConvertor[] getMsgConvertors() {
return msgConvertors;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy