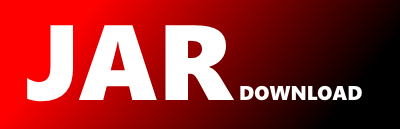
io.swagger.client.model.BouncedCategorySummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Summary of bounced categories, based on specified date range.
*/
@ApiModel(description = "Summary of bounced categories, based on specified date range.")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class BouncedCategorySummary {
@SerializedName("Spam")
private Long spam = null;
@SerializedName("BlackListed")
private Long blackListed = null;
@SerializedName("NoMailbox")
private Long noMailbox = null;
@SerializedName("GreyListed")
private Long greyListed = null;
@SerializedName("Throttled")
private Long throttled = null;
@SerializedName("Timeout")
private Long timeout = null;
@SerializedName("ConnectionProblem")
private Long connectionProblem = null;
@SerializedName("SpfProblem")
private Long spfProblem = null;
@SerializedName("AccountProblem")
private Long accountProblem = null;
@SerializedName("DnsProblem")
private Long dnsProblem = null;
@SerializedName("WhitelistingProblem")
private Long whitelistingProblem = null;
@SerializedName("CodeError")
private Long codeError = null;
@SerializedName("NotDelivered")
private Long notDelivered = null;
@SerializedName("ManualCancel")
private Long manualCancel = null;
@SerializedName("ConnectionTerminated")
private Long connectionTerminated = null;
public BouncedCategorySummary spam(Long spam) {
this.spam = spam;
return this;
}
/**
* Number of messages marked as SPAM
* @return spam
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of messages marked as SPAM")
public Long getSpam() {
return spam;
}
public void setSpam(Long spam) {
this.spam = spam;
}
public BouncedCategorySummary blackListed(Long blackListed) {
this.blackListed = blackListed;
return this;
}
/**
* Number of blacklisted messages
* @return blackListed
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of blacklisted messages")
public Long getBlackListed() {
return blackListed;
}
public void setBlackListed(Long blackListed) {
this.blackListed = blackListed;
}
public BouncedCategorySummary noMailbox(Long noMailbox) {
this.noMailbox = noMailbox;
return this;
}
/**
* Number of messages flagged with 'No Mailbox'
* @return noMailbox
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'No Mailbox'")
public Long getNoMailbox() {
return noMailbox;
}
public void setNoMailbox(Long noMailbox) {
this.noMailbox = noMailbox;
}
public BouncedCategorySummary greyListed(Long greyListed) {
this.greyListed = greyListed;
return this;
}
/**
* Number of messages flagged with 'Grey Listed'
* @return greyListed
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Grey Listed'")
public Long getGreyListed() {
return greyListed;
}
public void setGreyListed(Long greyListed) {
this.greyListed = greyListed;
}
public BouncedCategorySummary throttled(Long throttled) {
this.throttled = throttled;
return this;
}
/**
* Number of messages flagged with 'Throttled'
* @return throttled
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Throttled'")
public Long getThrottled() {
return throttled;
}
public void setThrottled(Long throttled) {
this.throttled = throttled;
}
public BouncedCategorySummary timeout(Long timeout) {
this.timeout = timeout;
return this;
}
/**
* Number of messages flagged with 'Timeout'
* @return timeout
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Timeout'")
public Long getTimeout() {
return timeout;
}
public void setTimeout(Long timeout) {
this.timeout = timeout;
}
public BouncedCategorySummary connectionProblem(Long connectionProblem) {
this.connectionProblem = connectionProblem;
return this;
}
/**
* Number of messages flagged with 'Connection Problem'
* @return connectionProblem
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Connection Problem'")
public Long getConnectionProblem() {
return connectionProblem;
}
public void setConnectionProblem(Long connectionProblem) {
this.connectionProblem = connectionProblem;
}
public BouncedCategorySummary spfProblem(Long spfProblem) {
this.spfProblem = spfProblem;
return this;
}
/**
* Number of messages flagged with 'SPF Problem'
* @return spfProblem
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'SPF Problem'")
public Long getSpfProblem() {
return spfProblem;
}
public void setSpfProblem(Long spfProblem) {
this.spfProblem = spfProblem;
}
public BouncedCategorySummary accountProblem(Long accountProblem) {
this.accountProblem = accountProblem;
return this;
}
/**
* Number of messages flagged with 'Account Problem'
* @return accountProblem
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Account Problem'")
public Long getAccountProblem() {
return accountProblem;
}
public void setAccountProblem(Long accountProblem) {
this.accountProblem = accountProblem;
}
public BouncedCategorySummary dnsProblem(Long dnsProblem) {
this.dnsProblem = dnsProblem;
return this;
}
/**
* Number of messages flagged with 'DNS Problem'
* @return dnsProblem
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'DNS Problem'")
public Long getDnsProblem() {
return dnsProblem;
}
public void setDnsProblem(Long dnsProblem) {
this.dnsProblem = dnsProblem;
}
public BouncedCategorySummary whitelistingProblem(Long whitelistingProblem) {
this.whitelistingProblem = whitelistingProblem;
return this;
}
/**
* Number of messages flagged with 'WhiteListing Problem'
* @return whitelistingProblem
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'WhiteListing Problem'")
public Long getWhitelistingProblem() {
return whitelistingProblem;
}
public void setWhitelistingProblem(Long whitelistingProblem) {
this.whitelistingProblem = whitelistingProblem;
}
public BouncedCategorySummary codeError(Long codeError) {
this.codeError = codeError;
return this;
}
/**
* Number of messages flagged with 'Code Error'
* @return codeError
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Code Error'")
public Long getCodeError() {
return codeError;
}
public void setCodeError(Long codeError) {
this.codeError = codeError;
}
public BouncedCategorySummary notDelivered(Long notDelivered) {
this.notDelivered = notDelivered;
return this;
}
/**
* Number of messages flagged with 'Not Delivered'
* @return notDelivered
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Not Delivered'")
public Long getNotDelivered() {
return notDelivered;
}
public void setNotDelivered(Long notDelivered) {
this.notDelivered = notDelivered;
}
public BouncedCategorySummary manualCancel(Long manualCancel) {
this.manualCancel = manualCancel;
return this;
}
/**
* Number of manually cancelled messages
* @return manualCancel
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of manually cancelled messages")
public Long getManualCancel() {
return manualCancel;
}
public void setManualCancel(Long manualCancel) {
this.manualCancel = manualCancel;
}
public BouncedCategorySummary connectionTerminated(Long connectionTerminated) {
this.connectionTerminated = connectionTerminated;
return this;
}
/**
* Number of messages flagged with 'Connection terminated'
* @return connectionTerminated
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Connection terminated'")
public Long getConnectionTerminated() {
return connectionTerminated;
}
public void setConnectionTerminated(Long connectionTerminated) {
this.connectionTerminated = connectionTerminated;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BouncedCategorySummary bouncedCategorySummary = (BouncedCategorySummary) o;
return Objects.equals(this.spam, bouncedCategorySummary.spam) &&
Objects.equals(this.blackListed, bouncedCategorySummary.blackListed) &&
Objects.equals(this.noMailbox, bouncedCategorySummary.noMailbox) &&
Objects.equals(this.greyListed, bouncedCategorySummary.greyListed) &&
Objects.equals(this.throttled, bouncedCategorySummary.throttled) &&
Objects.equals(this.timeout, bouncedCategorySummary.timeout) &&
Objects.equals(this.connectionProblem, bouncedCategorySummary.connectionProblem) &&
Objects.equals(this.spfProblem, bouncedCategorySummary.spfProblem) &&
Objects.equals(this.accountProblem, bouncedCategorySummary.accountProblem) &&
Objects.equals(this.dnsProblem, bouncedCategorySummary.dnsProblem) &&
Objects.equals(this.whitelistingProblem, bouncedCategorySummary.whitelistingProblem) &&
Objects.equals(this.codeError, bouncedCategorySummary.codeError) &&
Objects.equals(this.notDelivered, bouncedCategorySummary.notDelivered) &&
Objects.equals(this.manualCancel, bouncedCategorySummary.manualCancel) &&
Objects.equals(this.connectionTerminated, bouncedCategorySummary.connectionTerminated);
}
@Override
public int hashCode() {
return Objects.hash(spam, blackListed, noMailbox, greyListed, throttled, timeout, connectionProblem, spfProblem, accountProblem, dnsProblem, whitelistingProblem, codeError, notDelivered, manualCancel, connectionTerminated);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BouncedCategorySummary {\n");
sb.append(" spam: ").append(toIndentedString(spam)).append("\n");
sb.append(" blackListed: ").append(toIndentedString(blackListed)).append("\n");
sb.append(" noMailbox: ").append(toIndentedString(noMailbox)).append("\n");
sb.append(" greyListed: ").append(toIndentedString(greyListed)).append("\n");
sb.append(" throttled: ").append(toIndentedString(throttled)).append("\n");
sb.append(" timeout: ").append(toIndentedString(timeout)).append("\n");
sb.append(" connectionProblem: ").append(toIndentedString(connectionProblem)).append("\n");
sb.append(" spfProblem: ").append(toIndentedString(spfProblem)).append("\n");
sb.append(" accountProblem: ").append(toIndentedString(accountProblem)).append("\n");
sb.append(" dnsProblem: ").append(toIndentedString(dnsProblem)).append("\n");
sb.append(" whitelistingProblem: ").append(toIndentedString(whitelistingProblem)).append("\n");
sb.append(" codeError: ").append(toIndentedString(codeError)).append("\n");
sb.append(" notDelivered: ").append(toIndentedString(notDelivered)).append("\n");
sb.append(" manualCancel: ").append(toIndentedString(manualCancel)).append("\n");
sb.append(" connectionTerminated: ").append(toIndentedString(connectionTerminated)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy