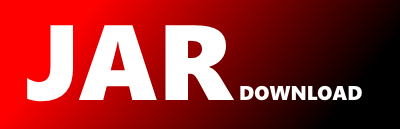
io.swagger.client.model.Contact Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.threeten.bp.OffsetDateTime;
/**
* Contact
*/
@ApiModel(description = "Contact")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class Contact {
@SerializedName("ContactScore")
private Integer contactScore = null;
@SerializedName("DateAdded")
private OffsetDateTime dateAdded = null;
@SerializedName("Email")
private String email = null;
@SerializedName("FirstName")
private String firstName = null;
@SerializedName("LastName")
private String lastName = null;
/**
* Name of status: Active, Engaged, Inactive, Abuse, Bounced, Unsubscribed.
*/
@JsonAdapter(StatusEnum.Adapter.class)
public enum StatusEnum {
TRANSACTIONAL("Transactional"),
ENGAGED("Engaged"),
ACTIVE("Active"),
BOUNCED("Bounced"),
UNSUBSCRIBED("Unsubscribed"),
ABUSE("Abuse"),
INACTIVE("Inactive"),
STALE("Stale"),
NOTCONFIRMED("NotConfirmed");
private String value;
StatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final StatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public StatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return StatusEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("Status")
private StatusEnum status = null;
@SerializedName("BouncedErrorCode")
private Integer bouncedErrorCode = null;
@SerializedName("BouncedErrorMessage")
private String bouncedErrorMessage = null;
@SerializedName("TotalSent")
private Integer totalSent = null;
@SerializedName("TotalFailed")
private Integer totalFailed = null;
@SerializedName("TotalOpened")
private Integer totalOpened = null;
@SerializedName("TotalClicked")
private Integer totalClicked = null;
@SerializedName("FirstFailedDate")
private OffsetDateTime firstFailedDate = null;
@SerializedName("LastFailedCount")
private Integer lastFailedCount = null;
@SerializedName("DateUpdated")
private OffsetDateTime dateUpdated = null;
/**
* Source of URL of payment
*/
@JsonAdapter(SourceEnum.Adapter.class)
public enum SourceEnum {
DELIVERYAPI("DeliveryApi"),
MANUALINPUT("ManualInput"),
FILEUPLOAD("FileUpload"),
WEBFORM("WebForm"),
CONTACTAPI("ContactApi");
private String value;
SourceEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SourceEnum fromValue(String text) {
for (SourceEnum b : SourceEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SourceEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SourceEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SourceEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("Source")
private SourceEnum source = null;
@SerializedName("ErrorCode")
private Integer errorCode = null;
@SerializedName("FriendlyErrorMessage")
private String friendlyErrorMessage = null;
@SerializedName("CreatedFromIP")
private String createdFromIP = null;
@SerializedName("ConsentIP")
private String consentIP = null;
@SerializedName("ConsentDate")
private OffsetDateTime consentDate = null;
/**
* Gets or Sets consentTracking
*/
@JsonAdapter(ConsentTrackingEnum.Adapter.class)
public enum ConsentTrackingEnum {
UNKNOWN("Unknown"),
ALLOW("Allow"),
DENY("Deny");
private String value;
ConsentTrackingEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ConsentTrackingEnum fromValue(String text) {
for (ConsentTrackingEnum b : ConsentTrackingEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ConsentTrackingEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ConsentTrackingEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ConsentTrackingEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("ConsentTracking")
private ConsentTrackingEnum consentTracking = null;
@SerializedName("UnsubscribedDate")
private OffsetDateTime unsubscribedDate = null;
@SerializedName("Notes")
private String notes = null;
@SerializedName("WebsiteUrl")
private String websiteUrl = null;
@SerializedName("LastOpened")
private OffsetDateTime lastOpened = null;
@SerializedName("LastClicked")
private OffsetDateTime lastClicked = null;
@SerializedName("CustomFields")
private Map customFields = new HashMap();
public Contact contactScore(Integer contactScore) {
this.contactScore = contactScore;
return this;
}
/**
* Get contactScore
* @return contactScore
**/
@ApiModelProperty(required = true, value = "")
public Integer getContactScore() {
return contactScore;
}
public void setContactScore(Integer contactScore) {
this.contactScore = contactScore;
}
public Contact dateAdded(OffsetDateTime dateAdded) {
this.dateAdded = dateAdded;
return this;
}
/**
* Date of creation in YYYY-MM-DDThh:ii:ss format
* @return dateAdded
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date of creation in YYYY-MM-DDThh:ii:ss format")
public OffsetDateTime getDateAdded() {
return dateAdded;
}
public void setDateAdded(OffsetDateTime dateAdded) {
this.dateAdded = dateAdded;
}
public Contact email(String email) {
this.email = email;
return this;
}
/**
* Proper email address.
* @return email
**/
@ApiModelProperty(example = "[email protected]", required = true, value = "Proper email address.")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Contact firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* First name.
* @return firstName
**/
@ApiModelProperty(example = "FIRSTNAME", required = true, value = "First name.")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public Contact lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Last name.
* @return lastName
**/
@ApiModelProperty(example = "LASTNAME", required = true, value = "Last name.")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public Contact status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Name of status: Active, Engaged, Inactive, Abuse, Bounced, Unsubscribed.
* @return status
**/
@ApiModelProperty(example = "Bounced", required = true, value = "Name of status: Active, Engaged, Inactive, Abuse, Bounced, Unsubscribed.")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public Contact bouncedErrorCode(Integer bouncedErrorCode) {
this.bouncedErrorCode = bouncedErrorCode;
return this;
}
/**
* RFC Error code
* @return bouncedErrorCode
**/
@ApiModelProperty(example = "550", value = "RFC Error code")
public Integer getBouncedErrorCode() {
return bouncedErrorCode;
}
public void setBouncedErrorCode(Integer bouncedErrorCode) {
this.bouncedErrorCode = bouncedErrorCode;
}
public Contact bouncedErrorMessage(String bouncedErrorMessage) {
this.bouncedErrorMessage = bouncedErrorMessage;
return this;
}
/**
* RFC error message
* @return bouncedErrorMessage
**/
@ApiModelProperty(example = "Mailbox not found", required = true, value = "RFC error message")
public String getBouncedErrorMessage() {
return bouncedErrorMessage;
}
public void setBouncedErrorMessage(String bouncedErrorMessage) {
this.bouncedErrorMessage = bouncedErrorMessage;
}
public Contact totalSent(Integer totalSent) {
this.totalSent = totalSent;
return this;
}
/**
* Total emails sent.
* @return totalSent
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails sent.")
public Integer getTotalSent() {
return totalSent;
}
public void setTotalSent(Integer totalSent) {
this.totalSent = totalSent;
}
public Contact totalFailed(Integer totalFailed) {
this.totalFailed = totalFailed;
return this;
}
/**
* Total emails failed.
* @return totalFailed
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails failed.")
public Integer getTotalFailed() {
return totalFailed;
}
public void setTotalFailed(Integer totalFailed) {
this.totalFailed = totalFailed;
}
public Contact totalOpened(Integer totalOpened) {
this.totalOpened = totalOpened;
return this;
}
/**
* Total emails opened.
* @return totalOpened
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails opened.")
public Integer getTotalOpened() {
return totalOpened;
}
public void setTotalOpened(Integer totalOpened) {
this.totalOpened = totalOpened;
}
public Contact totalClicked(Integer totalClicked) {
this.totalClicked = totalClicked;
return this;
}
/**
* Total emails clicked
* @return totalClicked
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails clicked")
public Integer getTotalClicked() {
return totalClicked;
}
public void setTotalClicked(Integer totalClicked) {
this.totalClicked = totalClicked;
}
public Contact firstFailedDate(OffsetDateTime firstFailedDate) {
this.firstFailedDate = firstFailedDate;
return this;
}
/**
* Date of first failed message
* @return firstFailedDate
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", value = "Date of first failed message")
public OffsetDateTime getFirstFailedDate() {
return firstFailedDate;
}
public void setFirstFailedDate(OffsetDateTime firstFailedDate) {
this.firstFailedDate = firstFailedDate;
}
public Contact lastFailedCount(Integer lastFailedCount) {
this.lastFailedCount = lastFailedCount;
return this;
}
/**
* Number of fails in sending to this Contact
* @return lastFailedCount
**/
@ApiModelProperty(example = "1", required = true, value = "Number of fails in sending to this Contact")
public Integer getLastFailedCount() {
return lastFailedCount;
}
public void setLastFailedCount(Integer lastFailedCount) {
this.lastFailedCount = lastFailedCount;
}
public Contact dateUpdated(OffsetDateTime dateUpdated) {
this.dateUpdated = dateUpdated;
return this;
}
/**
* Last change date
* @return dateUpdated
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Last change date")
public OffsetDateTime getDateUpdated() {
return dateUpdated;
}
public void setDateUpdated(OffsetDateTime dateUpdated) {
this.dateUpdated = dateUpdated;
}
public Contact source(SourceEnum source) {
this.source = source;
return this;
}
/**
* Source of URL of payment
* @return source
**/
@ApiModelProperty(example = "http://", required = true, value = "Source of URL of payment")
public SourceEnum getSource() {
return source;
}
public void setSource(SourceEnum source) {
this.source = source;
}
public Contact errorCode(Integer errorCode) {
this.errorCode = errorCode;
return this;
}
/**
* RFC Error code
* @return errorCode
**/
@ApiModelProperty(example = "550", value = "RFC Error code")
public Integer getErrorCode() {
return errorCode;
}
public void setErrorCode(Integer errorCode) {
this.errorCode = errorCode;
}
public Contact friendlyErrorMessage(String friendlyErrorMessage) {
this.friendlyErrorMessage = friendlyErrorMessage;
return this;
}
/**
* RFC error message
* @return friendlyErrorMessage
**/
@ApiModelProperty(example = "Mailbox not found", required = true, value = "RFC error message")
public String getFriendlyErrorMessage() {
return friendlyErrorMessage;
}
public void setFriendlyErrorMessage(String friendlyErrorMessage) {
this.friendlyErrorMessage = friendlyErrorMessage;
}
public Contact createdFromIP(String createdFromIP) {
this.createdFromIP = createdFromIP;
return this;
}
/**
* IP address
* @return createdFromIP
**/
@ApiModelProperty(example = "192.168.0.1", required = true, value = "IP address")
public String getCreatedFromIP() {
return createdFromIP;
}
public void setCreatedFromIP(String createdFromIP) {
this.createdFromIP = createdFromIP;
}
public Contact consentIP(String consentIP) {
this.consentIP = consentIP;
return this;
}
/**
* IP address of consent to send this contact(s) your email. If not provided your current public IP address is used for consent.
* @return consentIP
**/
@ApiModelProperty(example = "192.168.0.1", required = true, value = "IP address of consent to send this contact(s) your email. If not provided your current public IP address is used for consent.")
public String getConsentIP() {
return consentIP;
}
public void setConsentIP(String consentIP) {
this.consentIP = consentIP;
}
public Contact consentDate(OffsetDateTime consentDate) {
this.consentDate = consentDate;
return this;
}
/**
* Date of consent to send this contact(s) your email. If not provided current date is used for consent.
* @return consentDate
**/
@ApiModelProperty(example = "1/1/2015 0:00:00 AM", value = "Date of consent to send this contact(s) your email. If not provided current date is used for consent.")
public OffsetDateTime getConsentDate() {
return consentDate;
}
public void setConsentDate(OffsetDateTime consentDate) {
this.consentDate = consentDate;
}
public Contact consentTracking(ConsentTrackingEnum consentTracking) {
this.consentTracking = consentTracking;
return this;
}
/**
* Get consentTracking
* @return consentTracking
**/
@ApiModelProperty(required = true, value = "")
public ConsentTrackingEnum getConsentTracking() {
return consentTracking;
}
public void setConsentTracking(ConsentTrackingEnum consentTracking) {
this.consentTracking = consentTracking;
}
public Contact unsubscribedDate(OffsetDateTime unsubscribedDate) {
this.unsubscribedDate = unsubscribedDate;
return this;
}
/**
* Unsubscribed date in YYYY-MM-DD format
* @return unsubscribedDate
**/
@ApiModelProperty(example = "2001-01-01", value = "Unsubscribed date in YYYY-MM-DD format")
public OffsetDateTime getUnsubscribedDate() {
return unsubscribedDate;
}
public void setUnsubscribedDate(OffsetDateTime unsubscribedDate) {
this.unsubscribedDate = unsubscribedDate;
}
public Contact notes(String notes) {
this.notes = notes;
return this;
}
/**
* Free form field of notes
* @return notes
**/
@ApiModelProperty(example = "Contact is extremely important!", required = true, value = "Free form field of notes")
public String getNotes() {
return notes;
}
public void setNotes(String notes) {
this.notes = notes;
}
public Contact websiteUrl(String websiteUrl) {
this.websiteUrl = websiteUrl;
return this;
}
/**
* Website of contact
* @return websiteUrl
**/
@ApiModelProperty(example = "http://", required = true, value = "Website of contact")
public String getWebsiteUrl() {
return websiteUrl;
}
public void setWebsiteUrl(String websiteUrl) {
this.websiteUrl = websiteUrl;
}
public Contact lastOpened(OffsetDateTime lastOpened) {
this.lastOpened = lastOpened;
return this;
}
/**
* Date this contact last opened an email
* @return lastOpened
**/
@ApiModelProperty(example = "2014-01-01", value = "Date this contact last opened an email")
public OffsetDateTime getLastOpened() {
return lastOpened;
}
public void setLastOpened(OffsetDateTime lastOpened) {
this.lastOpened = lastOpened;
}
public Contact lastClicked(OffsetDateTime lastClicked) {
this.lastClicked = lastClicked;
return this;
}
/**
* Get lastClicked
* @return lastClicked
**/
@ApiModelProperty(value = "")
public OffsetDateTime getLastClicked() {
return lastClicked;
}
public void setLastClicked(OffsetDateTime lastClicked) {
this.lastClicked = lastClicked;
}
public Contact customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public Contact putCustomFieldsItem(String key, String customFieldsItem) {
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Get customFields
* @return customFields
**/
@ApiModelProperty(required = true, value = "")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Contact contact = (Contact) o;
return Objects.equals(this.contactScore, contact.contactScore) &&
Objects.equals(this.dateAdded, contact.dateAdded) &&
Objects.equals(this.email, contact.email) &&
Objects.equals(this.firstName, contact.firstName) &&
Objects.equals(this.lastName, contact.lastName) &&
Objects.equals(this.status, contact.status) &&
Objects.equals(this.bouncedErrorCode, contact.bouncedErrorCode) &&
Objects.equals(this.bouncedErrorMessage, contact.bouncedErrorMessage) &&
Objects.equals(this.totalSent, contact.totalSent) &&
Objects.equals(this.totalFailed, contact.totalFailed) &&
Objects.equals(this.totalOpened, contact.totalOpened) &&
Objects.equals(this.totalClicked, contact.totalClicked) &&
Objects.equals(this.firstFailedDate, contact.firstFailedDate) &&
Objects.equals(this.lastFailedCount, contact.lastFailedCount) &&
Objects.equals(this.dateUpdated, contact.dateUpdated) &&
Objects.equals(this.source, contact.source) &&
Objects.equals(this.errorCode, contact.errorCode) &&
Objects.equals(this.friendlyErrorMessage, contact.friendlyErrorMessage) &&
Objects.equals(this.createdFromIP, contact.createdFromIP) &&
Objects.equals(this.consentIP, contact.consentIP) &&
Objects.equals(this.consentDate, contact.consentDate) &&
Objects.equals(this.consentTracking, contact.consentTracking) &&
Objects.equals(this.unsubscribedDate, contact.unsubscribedDate) &&
Objects.equals(this.notes, contact.notes) &&
Objects.equals(this.websiteUrl, contact.websiteUrl) &&
Objects.equals(this.lastOpened, contact.lastOpened) &&
Objects.equals(this.lastClicked, contact.lastClicked) &&
Objects.equals(this.customFields, contact.customFields);
}
@Override
public int hashCode() {
return Objects.hash(contactScore, dateAdded, email, firstName, lastName, status, bouncedErrorCode, bouncedErrorMessage, totalSent, totalFailed, totalOpened, totalClicked, firstFailedDate, lastFailedCount, dateUpdated, source, errorCode, friendlyErrorMessage, createdFromIP, consentIP, consentDate, consentTracking, unsubscribedDate, notes, websiteUrl, lastOpened, lastClicked, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Contact {\n");
sb.append(" contactScore: ").append(toIndentedString(contactScore)).append("\n");
sb.append(" dateAdded: ").append(toIndentedString(dateAdded)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" bouncedErrorCode: ").append(toIndentedString(bouncedErrorCode)).append("\n");
sb.append(" bouncedErrorMessage: ").append(toIndentedString(bouncedErrorMessage)).append("\n");
sb.append(" totalSent: ").append(toIndentedString(totalSent)).append("\n");
sb.append(" totalFailed: ").append(toIndentedString(totalFailed)).append("\n");
sb.append(" totalOpened: ").append(toIndentedString(totalOpened)).append("\n");
sb.append(" totalClicked: ").append(toIndentedString(totalClicked)).append("\n");
sb.append(" firstFailedDate: ").append(toIndentedString(firstFailedDate)).append("\n");
sb.append(" lastFailedCount: ").append(toIndentedString(lastFailedCount)).append("\n");
sb.append(" dateUpdated: ").append(toIndentedString(dateUpdated)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append(" errorCode: ").append(toIndentedString(errorCode)).append("\n");
sb.append(" friendlyErrorMessage: ").append(toIndentedString(friendlyErrorMessage)).append("\n");
sb.append(" createdFromIP: ").append(toIndentedString(createdFromIP)).append("\n");
sb.append(" consentIP: ").append(toIndentedString(consentIP)).append("\n");
sb.append(" consentDate: ").append(toIndentedString(consentDate)).append("\n");
sb.append(" consentTracking: ").append(toIndentedString(consentTracking)).append("\n");
sb.append(" unsubscribedDate: ").append(toIndentedString(unsubscribedDate)).append("\n");
sb.append(" notes: ").append(toIndentedString(notes)).append("\n");
sb.append(" websiteUrl: ").append(toIndentedString(websiteUrl)).append("\n");
sb.append(" lastOpened: ").append(toIndentedString(lastOpened)).append("\n");
sb.append(" lastClicked: ").append(toIndentedString(lastClicked)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy