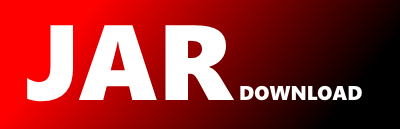
io.swagger.client.model.ContactHistory Maven / Gradle / Ivy
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* History of chosen Contact
*/
@ApiModel(description = "History of chosen Contact")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class ContactHistory {
@SerializedName("EventType")
private String eventType = null;
/**
* Numeric code of event occured on this Contact.
*/
@JsonAdapter(EventTypeValueEnum.Adapter.class)
public enum EventTypeValueEnum {
OPENED("Opened"),
CLICKED("Clicked"),
BOUNCED("Bounced"),
UNSUBSCRIBED("Unsubscribed"),
COMPLAINED("Complained"),
ACTIVATED("Activated"),
TRANSACTIONALUNSUBSCRIBED("TransactionalUnsubscribed"),
MANUALSTATUSCHANGE("ManualStatusChange"),
ACTIVATIONSENT("ActivationSent"),
DELETED("Deleted");
private String value;
EventTypeValueEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static EventTypeValueEnum fromValue(String text) {
for (EventTypeValueEnum b : EventTypeValueEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final EventTypeValueEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public EventTypeValueEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return EventTypeValueEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("EventTypeValue")
private EventTypeValueEnum eventTypeValue = null;
@SerializedName("EventDate")
private String eventDate = null;
@SerializedName("ChannelName")
private String channelName = null;
@SerializedName("TemplateName")
private String templateName = null;
@SerializedName("IPAddress")
private String ipAddress = null;
@SerializedName("Country")
private String country = null;
@SerializedName("Data")
private String data = null;
public ContactHistory eventType(String eventType) {
this.eventType = eventType;
return this;
}
/**
* Type of event occured on this Contact.
* @return eventType
**/
@ApiModelProperty(example = "Sent", required = true, value = "Type of event occured on this Contact.")
public String getEventType() {
return eventType;
}
public void setEventType(String eventType) {
this.eventType = eventType;
}
public ContactHistory eventTypeValue(EventTypeValueEnum eventTypeValue) {
this.eventTypeValue = eventTypeValue;
return this;
}
/**
* Numeric code of event occured on this Contact.
* @return eventTypeValue
**/
@ApiModelProperty(example = "1", required = true, value = "Numeric code of event occured on this Contact.")
public EventTypeValueEnum getEventTypeValue() {
return eventTypeValue;
}
public void setEventTypeValue(EventTypeValueEnum eventTypeValue) {
this.eventTypeValue = eventTypeValue;
}
public ContactHistory eventDate(String eventDate) {
this.eventDate = eventDate;
return this;
}
/**
* Formatted date of event.
* @return eventDate
**/
@ApiModelProperty(example = "1/1/2015 0:00:00 AM", required = true, value = "Formatted date of event.")
public String getEventDate() {
return eventDate;
}
public void setEventDate(String eventDate) {
this.eventDate = eventDate;
}
public ContactHistory channelName(String channelName) {
this.channelName = channelName;
return this;
}
/**
* Name of selected channel.
* @return channelName
**/
@ApiModelProperty(example = "Channel01", required = true, value = "Name of selected channel.")
public String getChannelName() {
return channelName;
}
public void setChannelName(String channelName) {
this.channelName = channelName;
}
public ContactHistory templateName(String templateName) {
this.templateName = templateName;
return this;
}
/**
* Name of template.
* @return templateName
**/
@ApiModelProperty(example = "Template01", required = true, value = "Name of template.")
public String getTemplateName() {
return templateName;
}
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
public ContactHistory ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* IP Address of the event.
* @return ipAddress
**/
@ApiModelProperty(required = true, value = "IP Address of the event.")
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public ContactHistory country(String country) {
this.country = country;
return this;
}
/**
* Country of the event.
* @return country
**/
@ApiModelProperty(required = true, value = "Country of the event.")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public ContactHistory data(String data) {
this.data = data;
return this;
}
/**
* Information about the event
* @return data
**/
@ApiModelProperty(required = true, value = "Information about the event")
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ContactHistory contactHistory = (ContactHistory) o;
return Objects.equals(this.eventType, contactHistory.eventType) &&
Objects.equals(this.eventTypeValue, contactHistory.eventTypeValue) &&
Objects.equals(this.eventDate, contactHistory.eventDate) &&
Objects.equals(this.channelName, contactHistory.channelName) &&
Objects.equals(this.templateName, contactHistory.templateName) &&
Objects.equals(this.ipAddress, contactHistory.ipAddress) &&
Objects.equals(this.country, contactHistory.country) &&
Objects.equals(this.data, contactHistory.data);
}
@Override
public int hashCode() {
return Objects.hash(eventType, eventTypeValue, eventDate, channelName, templateName, ipAddress, country, data);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ContactHistory {\n");
sb.append(" eventType: ").append(toIndentedString(eventType)).append("\n");
sb.append(" eventTypeValue: ").append(toIndentedString(eventTypeValue)).append("\n");
sb.append(" eventDate: ").append(toIndentedString(eventDate)).append("\n");
sb.append(" channelName: ").append(toIndentedString(channelName)).append("\n");
sb.append(" templateName: ").append(toIndentedString(templateName)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy