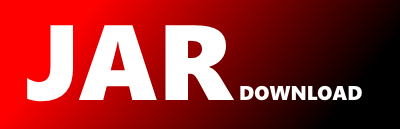
io.swagger.client.model.ContactStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.ContactUnsubscribeReasonCounts;
import java.io.IOException;
/**
*
*/
@ApiModel(description = "")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class ContactStats {
@SerializedName("Engaged")
private Long engaged = null;
@SerializedName("Active")
private Long active = null;
@SerializedName("Complaint")
private Long complaint = null;
@SerializedName("Unsubscribed")
private ContactUnsubscribeReasonCounts unsubscribed = null;
@SerializedName("Bounced")
private Long bounced = null;
@SerializedName("Inactive")
private Long inactive = null;
@SerializedName("Transactional")
private Long transactional = null;
@SerializedName("Stale")
private Long stale = null;
@SerializedName("NotConfirmed")
private Long notConfirmed = null;
public ContactStats engaged(Long engaged) {
this.engaged = engaged;
return this;
}
/**
* Number of engaged contacts
* @return engaged
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of engaged contacts")
public Long getEngaged() {
return engaged;
}
public void setEngaged(Long engaged) {
this.engaged = engaged;
}
public ContactStats active(Long active) {
this.active = active;
return this;
}
/**
* Number of active contacts
* @return active
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of active contacts")
public Long getActive() {
return active;
}
public void setActive(Long active) {
this.active = active;
}
public ContactStats complaint(Long complaint) {
this.complaint = complaint;
return this;
}
/**
* Number of complaint messages
* @return complaint
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of complaint messages")
public Long getComplaint() {
return complaint;
}
public void setComplaint(Long complaint) {
this.complaint = complaint;
}
public ContactStats unsubscribed(ContactUnsubscribeReasonCounts unsubscribed) {
this.unsubscribed = unsubscribed;
return this;
}
/**
* Get unsubscribed
* @return unsubscribed
**/
@ApiModelProperty(required = true, value = "")
public ContactUnsubscribeReasonCounts getUnsubscribed() {
return unsubscribed;
}
public void setUnsubscribed(ContactUnsubscribeReasonCounts unsubscribed) {
this.unsubscribed = unsubscribed;
}
public ContactStats bounced(Long bounced) {
this.bounced = bounced;
return this;
}
/**
* Number of bounced messages
* @return bounced
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of bounced messages")
public Long getBounced() {
return bounced;
}
public void setBounced(Long bounced) {
this.bounced = bounced;
}
public ContactStats inactive(Long inactive) {
this.inactive = inactive;
return this;
}
/**
* Number of inactive contacts
* @return inactive
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of inactive contacts")
public Long getInactive() {
return inactive;
}
public void setInactive(Long inactive) {
this.inactive = inactive;
}
public ContactStats transactional(Long transactional) {
this.transactional = transactional;
return this;
}
/**
* Number of transactional contacts
* @return transactional
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of transactional contacts")
public Long getTransactional() {
return transactional;
}
public void setTransactional(Long transactional) {
this.transactional = transactional;
}
public ContactStats stale(Long stale) {
this.stale = stale;
return this;
}
/**
* Get stale
* @return stale
**/
@ApiModelProperty(required = true, value = "")
public Long getStale() {
return stale;
}
public void setStale(Long stale) {
this.stale = stale;
}
public ContactStats notConfirmed(Long notConfirmed) {
this.notConfirmed = notConfirmed;
return this;
}
/**
* Get notConfirmed
* @return notConfirmed
**/
@ApiModelProperty(required = true, value = "")
public Long getNotConfirmed() {
return notConfirmed;
}
public void setNotConfirmed(Long notConfirmed) {
this.notConfirmed = notConfirmed;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ContactStats contactStats = (ContactStats) o;
return Objects.equals(this.engaged, contactStats.engaged) &&
Objects.equals(this.active, contactStats.active) &&
Objects.equals(this.complaint, contactStats.complaint) &&
Objects.equals(this.unsubscribed, contactStats.unsubscribed) &&
Objects.equals(this.bounced, contactStats.bounced) &&
Objects.equals(this.inactive, contactStats.inactive) &&
Objects.equals(this.transactional, contactStats.transactional) &&
Objects.equals(this.stale, contactStats.stale) &&
Objects.equals(this.notConfirmed, contactStats.notConfirmed);
}
@Override
public int hashCode() {
return Objects.hash(engaged, active, complaint, unsubscribed, bounced, inactive, transactional, stale, notConfirmed);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ContactStats {\n");
sb.append(" engaged: ").append(toIndentedString(engaged)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" complaint: ").append(toIndentedString(complaint)).append("\n");
sb.append(" unsubscribed: ").append(toIndentedString(unsubscribed)).append("\n");
sb.append(" bounced: ").append(toIndentedString(bounced)).append("\n");
sb.append(" inactive: ").append(toIndentedString(inactive)).append("\n");
sb.append(" transactional: ").append(toIndentedString(transactional)).append("\n");
sb.append(" stale: ").append(toIndentedString(stale)).append("\n");
sb.append(" notConfirmed: ").append(toIndentedString(notConfirmed)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy