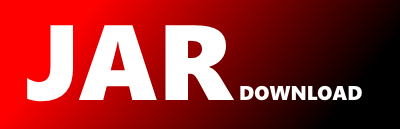
io.swagger.client.model.DailyLogStatusSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Daily summary of log status, based on specified date range.
*/
@ApiModel(description = "Daily summary of log status, based on specified date range.")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class DailyLogStatusSummary {
@SerializedName("Date")
private String date = null;
@SerializedName("Email")
private Integer email = null;
@SerializedName("Sms")
private Integer sms = null;
@SerializedName("Delivered")
private Integer delivered = null;
@SerializedName("Opened")
private Integer opened = null;
@SerializedName("Clicked")
private Integer clicked = null;
@SerializedName("Unsubscribed")
private Integer unsubscribed = null;
@SerializedName("Complaint")
private Integer complaint = null;
@SerializedName("Bounced")
private Integer bounced = null;
@SerializedName("Inbound")
private Integer inbound = null;
@SerializedName("ManualCancel")
private Integer manualCancel = null;
@SerializedName("NotDelivered")
private Integer notDelivered = null;
public DailyLogStatusSummary date(String date) {
this.date = date;
return this;
}
/**
* Date in YYYY-MM-DDThh:ii:ss format
* @return date
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date in YYYY-MM-DDThh:ii:ss format")
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public DailyLogStatusSummary email(Integer email) {
this.email = email;
return this;
}
/**
* Proper email address.
* @return email
**/
@ApiModelProperty(example = "[email protected]", required = true, value = "Proper email address.")
public Integer getEmail() {
return email;
}
public void setEmail(Integer email) {
this.email = email;
}
public DailyLogStatusSummary sms(Integer sms) {
this.sms = sms;
return this;
}
/**
* Number of SMS
* @return sms
**/
@ApiModelProperty(example = "12", required = true, value = "Number of SMS")
public Integer getSms() {
return sms;
}
public void setSms(Integer sms) {
this.sms = sms;
}
public DailyLogStatusSummary delivered(Integer delivered) {
this.delivered = delivered;
return this;
}
/**
* Number of delivered messages
* @return delivered
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of delivered messages")
public Integer getDelivered() {
return delivered;
}
public void setDelivered(Integer delivered) {
this.delivered = delivered;
}
public DailyLogStatusSummary opened(Integer opened) {
this.opened = opened;
return this;
}
/**
* Number of opened messages
* @return opened
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of opened messages")
public Integer getOpened() {
return opened;
}
public void setOpened(Integer opened) {
this.opened = opened;
}
public DailyLogStatusSummary clicked(Integer clicked) {
this.clicked = clicked;
return this;
}
/**
* Number of clicked messages
* @return clicked
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of clicked messages")
public Integer getClicked() {
return clicked;
}
public void setClicked(Integer clicked) {
this.clicked = clicked;
}
public DailyLogStatusSummary unsubscribed(Integer unsubscribed) {
this.unsubscribed = unsubscribed;
return this;
}
/**
* Number of unsubscribed messages
* @return unsubscribed
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of unsubscribed messages")
public Integer getUnsubscribed() {
return unsubscribed;
}
public void setUnsubscribed(Integer unsubscribed) {
this.unsubscribed = unsubscribed;
}
public DailyLogStatusSummary complaint(Integer complaint) {
this.complaint = complaint;
return this;
}
/**
* Number of complaint messages
* @return complaint
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of complaint messages")
public Integer getComplaint() {
return complaint;
}
public void setComplaint(Integer complaint) {
this.complaint = complaint;
}
public DailyLogStatusSummary bounced(Integer bounced) {
this.bounced = bounced;
return this;
}
/**
* Number of bounced messages
* @return bounced
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of bounced messages")
public Integer getBounced() {
return bounced;
}
public void setBounced(Integer bounced) {
this.bounced = bounced;
}
public DailyLogStatusSummary inbound(Integer inbound) {
this.inbound = inbound;
return this;
}
/**
* Number of inbound messages
* @return inbound
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of inbound messages")
public Integer getInbound() {
return inbound;
}
public void setInbound(Integer inbound) {
this.inbound = inbound;
}
public DailyLogStatusSummary manualCancel(Integer manualCancel) {
this.manualCancel = manualCancel;
return this;
}
/**
* Number of manually cancelled messages
* @return manualCancel
**/
@ApiModelProperty(example = "1000", required = true, value = "Number of manually cancelled messages")
public Integer getManualCancel() {
return manualCancel;
}
public void setManualCancel(Integer manualCancel) {
this.manualCancel = manualCancel;
}
public DailyLogStatusSummary notDelivered(Integer notDelivered) {
this.notDelivered = notDelivered;
return this;
}
/**
* Number of messages flagged with 'Not Delivered'
* @return notDelivered
**/
@ApiModelProperty(example = "0", required = true, value = "Number of messages flagged with 'Not Delivered'")
public Integer getNotDelivered() {
return notDelivered;
}
public void setNotDelivered(Integer notDelivered) {
this.notDelivered = notDelivered;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DailyLogStatusSummary dailyLogStatusSummary = (DailyLogStatusSummary) o;
return Objects.equals(this.date, dailyLogStatusSummary.date) &&
Objects.equals(this.email, dailyLogStatusSummary.email) &&
Objects.equals(this.sms, dailyLogStatusSummary.sms) &&
Objects.equals(this.delivered, dailyLogStatusSummary.delivered) &&
Objects.equals(this.opened, dailyLogStatusSummary.opened) &&
Objects.equals(this.clicked, dailyLogStatusSummary.clicked) &&
Objects.equals(this.unsubscribed, dailyLogStatusSummary.unsubscribed) &&
Objects.equals(this.complaint, dailyLogStatusSummary.complaint) &&
Objects.equals(this.bounced, dailyLogStatusSummary.bounced) &&
Objects.equals(this.inbound, dailyLogStatusSummary.inbound) &&
Objects.equals(this.manualCancel, dailyLogStatusSummary.manualCancel) &&
Objects.equals(this.notDelivered, dailyLogStatusSummary.notDelivered);
}
@Override
public int hashCode() {
return Objects.hash(date, email, sms, delivered, opened, clicked, unsubscribed, complaint, bounced, inbound, manualCancel, notDelivered);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DailyLogStatusSummary {\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" sms: ").append(toIndentedString(sms)).append("\n");
sb.append(" delivered: ").append(toIndentedString(delivered)).append("\n");
sb.append(" opened: ").append(toIndentedString(opened)).append("\n");
sb.append(" clicked: ").append(toIndentedString(clicked)).append("\n");
sb.append(" unsubscribed: ").append(toIndentedString(unsubscribed)).append("\n");
sb.append(" complaint: ").append(toIndentedString(complaint)).append("\n");
sb.append(" bounced: ").append(toIndentedString(bounced)).append("\n");
sb.append(" inbound: ").append(toIndentedString(inbound)).append("\n");
sb.append(" manualCancel: ").append(toIndentedString(manualCancel)).append("\n");
sb.append(" notDelivered: ").append(toIndentedString(notDelivered)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy