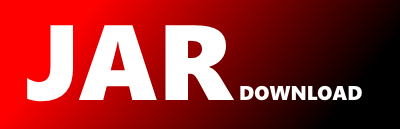
io.swagger.client.model.EmailJobStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.EmailJobFailedStatus;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* EmailJobStatus
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class EmailJobStatus {
@SerializedName("ID")
private String ID = null;
@SerializedName("Status")
private String status = null;
@SerializedName("RecipientsCount")
private Integer recipientsCount = null;
@SerializedName("Failed")
private List failed = new ArrayList();
@SerializedName("FailedCount")
private Integer failedCount = null;
@SerializedName("Sent")
private List sent = new ArrayList();
@SerializedName("SentCount")
private Integer sentCount = null;
@SerializedName("Delivered")
private List delivered = new ArrayList();
@SerializedName("DeliveredCount")
private Integer deliveredCount = null;
@SerializedName("Pending")
private List pending = new ArrayList();
@SerializedName("PendingCount")
private Integer pendingCount = null;
@SerializedName("Opened")
private List opened = new ArrayList();
@SerializedName("OpenedCount")
private Integer openedCount = null;
@SerializedName("Clicked")
private List clicked = new ArrayList();
@SerializedName("ClickedCount")
private Integer clickedCount = null;
@SerializedName("Unsubscribed")
private List unsubscribed = new ArrayList();
@SerializedName("UnsubscribedCount")
private Integer unsubscribedCount = null;
@SerializedName("AbuseReports")
private List abuseReports = new ArrayList();
@SerializedName("AbuseReportsCount")
private Integer abuseReportsCount = null;
@SerializedName("MessageIDs")
private List messageIDs = new ArrayList();
public EmailJobStatus ID(String ID) {
this.ID = ID;
return this;
}
/**
* ID number of your attachment
* @return ID
**/
@ApiModelProperty(example = "123456", required = true, value = "ID number of your attachment")
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
public EmailJobStatus status(String status) {
this.status = status;
return this;
}
/**
* Name of status: submitted, complete, in_progress
* @return status
**/
@ApiModelProperty(required = true, value = "Name of status: submitted, complete, in_progress")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public EmailJobStatus recipientsCount(Integer recipientsCount) {
this.recipientsCount = recipientsCount;
return this;
}
/**
* Get recipientsCount
* @return recipientsCount
**/
@ApiModelProperty(required = true, value = "")
public Integer getRecipientsCount() {
return recipientsCount;
}
public void setRecipientsCount(Integer recipientsCount) {
this.recipientsCount = recipientsCount;
}
public EmailJobStatus failed(List failed) {
this.failed = failed;
return this;
}
public EmailJobStatus addFailedItem(EmailJobFailedStatus failedItem) {
this.failed.add(failedItem);
return this;
}
/**
* Get failed
* @return failed
**/
@ApiModelProperty(required = true, value = "")
public List getFailed() {
return failed;
}
public void setFailed(List failed) {
this.failed = failed;
}
public EmailJobStatus failedCount(Integer failedCount) {
this.failedCount = failedCount;
return this;
}
/**
* Total emails failed.
* @return failedCount
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails failed.")
public Integer getFailedCount() {
return failedCount;
}
public void setFailedCount(Integer failedCount) {
this.failedCount = failedCount;
}
public EmailJobStatus sent(List sent) {
this.sent = sent;
return this;
}
public EmailJobStatus addSentItem(String sentItem) {
this.sent.add(sentItem);
return this;
}
/**
* Get sent
* @return sent
**/
@ApiModelProperty(required = true, value = "")
public List getSent() {
return sent;
}
public void setSent(List sent) {
this.sent = sent;
}
public EmailJobStatus sentCount(Integer sentCount) {
this.sentCount = sentCount;
return this;
}
/**
* Total emails sent.
* @return sentCount
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails sent.")
public Integer getSentCount() {
return sentCount;
}
public void setSentCount(Integer sentCount) {
this.sentCount = sentCount;
}
public EmailJobStatus delivered(List delivered) {
this.delivered = delivered;
return this;
}
public EmailJobStatus addDeliveredItem(String deliveredItem) {
this.delivered.add(deliveredItem);
return this;
}
/**
* Number of delivered messages
* @return delivered
**/
@ApiModelProperty(required = true, value = "Number of delivered messages")
public List getDelivered() {
return delivered;
}
public void setDelivered(List delivered) {
this.delivered = delivered;
}
public EmailJobStatus deliveredCount(Integer deliveredCount) {
this.deliveredCount = deliveredCount;
return this;
}
/**
* Get deliveredCount
* @return deliveredCount
**/
@ApiModelProperty(required = true, value = "")
public Integer getDeliveredCount() {
return deliveredCount;
}
public void setDeliveredCount(Integer deliveredCount) {
this.deliveredCount = deliveredCount;
}
public EmailJobStatus pending(List pending) {
this.pending = pending;
return this;
}
public EmailJobStatus addPendingItem(String pendingItem) {
this.pending.add(pendingItem);
return this;
}
/**
* Get pending
* @return pending
**/
@ApiModelProperty(required = true, value = "")
public List getPending() {
return pending;
}
public void setPending(List pending) {
this.pending = pending;
}
public EmailJobStatus pendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
return this;
}
/**
* Get pendingCount
* @return pendingCount
**/
@ApiModelProperty(required = true, value = "")
public Integer getPendingCount() {
return pendingCount;
}
public void setPendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
}
public EmailJobStatus opened(List opened) {
this.opened = opened;
return this;
}
public EmailJobStatus addOpenedItem(String openedItem) {
this.opened.add(openedItem);
return this;
}
/**
* Number of opened messages
* @return opened
**/
@ApiModelProperty(required = true, value = "Number of opened messages")
public List getOpened() {
return opened;
}
public void setOpened(List opened) {
this.opened = opened;
}
public EmailJobStatus openedCount(Integer openedCount) {
this.openedCount = openedCount;
return this;
}
/**
* Total emails opened.
* @return openedCount
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails opened.")
public Integer getOpenedCount() {
return openedCount;
}
public void setOpenedCount(Integer openedCount) {
this.openedCount = openedCount;
}
public EmailJobStatus clicked(List clicked) {
this.clicked = clicked;
return this;
}
public EmailJobStatus addClickedItem(String clickedItem) {
this.clicked.add(clickedItem);
return this;
}
/**
* Number of clicked messages
* @return clicked
**/
@ApiModelProperty(required = true, value = "Number of clicked messages")
public List getClicked() {
return clicked;
}
public void setClicked(List clicked) {
this.clicked = clicked;
}
public EmailJobStatus clickedCount(Integer clickedCount) {
this.clickedCount = clickedCount;
return this;
}
/**
* Total emails clicked
* @return clickedCount
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails clicked")
public Integer getClickedCount() {
return clickedCount;
}
public void setClickedCount(Integer clickedCount) {
this.clickedCount = clickedCount;
}
public EmailJobStatus unsubscribed(List unsubscribed) {
this.unsubscribed = unsubscribed;
return this;
}
public EmailJobStatus addUnsubscribedItem(String unsubscribedItem) {
this.unsubscribed.add(unsubscribedItem);
return this;
}
/**
* Number of unsubscribed messages
* @return unsubscribed
**/
@ApiModelProperty(required = true, value = "Number of unsubscribed messages")
public List getUnsubscribed() {
return unsubscribed;
}
public void setUnsubscribed(List unsubscribed) {
this.unsubscribed = unsubscribed;
}
public EmailJobStatus unsubscribedCount(Integer unsubscribedCount) {
this.unsubscribedCount = unsubscribedCount;
return this;
}
/**
* Total emails unsubscribed
* @return unsubscribedCount
**/
@ApiModelProperty(example = "1000", required = true, value = "Total emails unsubscribed")
public Integer getUnsubscribedCount() {
return unsubscribedCount;
}
public void setUnsubscribedCount(Integer unsubscribedCount) {
this.unsubscribedCount = unsubscribedCount;
}
public EmailJobStatus abuseReports(List abuseReports) {
this.abuseReports = abuseReports;
return this;
}
public EmailJobStatus addAbuseReportsItem(String abuseReportsItem) {
this.abuseReports.add(abuseReportsItem);
return this;
}
/**
* Get abuseReports
* @return abuseReports
**/
@ApiModelProperty(required = true, value = "")
public List getAbuseReports() {
return abuseReports;
}
public void setAbuseReports(List abuseReports) {
this.abuseReports = abuseReports;
}
public EmailJobStatus abuseReportsCount(Integer abuseReportsCount) {
this.abuseReportsCount = abuseReportsCount;
return this;
}
/**
* Get abuseReportsCount
* @return abuseReportsCount
**/
@ApiModelProperty(required = true, value = "")
public Integer getAbuseReportsCount() {
return abuseReportsCount;
}
public void setAbuseReportsCount(Integer abuseReportsCount) {
this.abuseReportsCount = abuseReportsCount;
}
public EmailJobStatus messageIDs(List messageIDs) {
this.messageIDs = messageIDs;
return this;
}
public EmailJobStatus addMessageIDsItem(String messageIDsItem) {
this.messageIDs.add(messageIDsItem);
return this;
}
/**
* List of all MessageIDs for this job.
* @return messageIDs
**/
@ApiModelProperty(required = true, value = "List of all MessageIDs for this job.")
public List getMessageIDs() {
return messageIDs;
}
public void setMessageIDs(List messageIDs) {
this.messageIDs = messageIDs;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailJobStatus emailJobStatus = (EmailJobStatus) o;
return Objects.equals(this.ID, emailJobStatus.ID) &&
Objects.equals(this.status, emailJobStatus.status) &&
Objects.equals(this.recipientsCount, emailJobStatus.recipientsCount) &&
Objects.equals(this.failed, emailJobStatus.failed) &&
Objects.equals(this.failedCount, emailJobStatus.failedCount) &&
Objects.equals(this.sent, emailJobStatus.sent) &&
Objects.equals(this.sentCount, emailJobStatus.sentCount) &&
Objects.equals(this.delivered, emailJobStatus.delivered) &&
Objects.equals(this.deliveredCount, emailJobStatus.deliveredCount) &&
Objects.equals(this.pending, emailJobStatus.pending) &&
Objects.equals(this.pendingCount, emailJobStatus.pendingCount) &&
Objects.equals(this.opened, emailJobStatus.opened) &&
Objects.equals(this.openedCount, emailJobStatus.openedCount) &&
Objects.equals(this.clicked, emailJobStatus.clicked) &&
Objects.equals(this.clickedCount, emailJobStatus.clickedCount) &&
Objects.equals(this.unsubscribed, emailJobStatus.unsubscribed) &&
Objects.equals(this.unsubscribedCount, emailJobStatus.unsubscribedCount) &&
Objects.equals(this.abuseReports, emailJobStatus.abuseReports) &&
Objects.equals(this.abuseReportsCount, emailJobStatus.abuseReportsCount) &&
Objects.equals(this.messageIDs, emailJobStatus.messageIDs);
}
@Override
public int hashCode() {
return Objects.hash(ID, status, recipientsCount, failed, failedCount, sent, sentCount, delivered, deliveredCount, pending, pendingCount, opened, openedCount, clicked, clickedCount, unsubscribed, unsubscribedCount, abuseReports, abuseReportsCount, messageIDs);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailJobStatus {\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" recipientsCount: ").append(toIndentedString(recipientsCount)).append("\n");
sb.append(" failed: ").append(toIndentedString(failed)).append("\n");
sb.append(" failedCount: ").append(toIndentedString(failedCount)).append("\n");
sb.append(" sent: ").append(toIndentedString(sent)).append("\n");
sb.append(" sentCount: ").append(toIndentedString(sentCount)).append("\n");
sb.append(" delivered: ").append(toIndentedString(delivered)).append("\n");
sb.append(" deliveredCount: ").append(toIndentedString(deliveredCount)).append("\n");
sb.append(" pending: ").append(toIndentedString(pending)).append("\n");
sb.append(" pendingCount: ").append(toIndentedString(pendingCount)).append("\n");
sb.append(" opened: ").append(toIndentedString(opened)).append("\n");
sb.append(" openedCount: ").append(toIndentedString(openedCount)).append("\n");
sb.append(" clicked: ").append(toIndentedString(clicked)).append("\n");
sb.append(" clickedCount: ").append(toIndentedString(clickedCount)).append("\n");
sb.append(" unsubscribed: ").append(toIndentedString(unsubscribed)).append("\n");
sb.append(" unsubscribedCount: ").append(toIndentedString(unsubscribedCount)).append("\n");
sb.append(" abuseReports: ").append(toIndentedString(abuseReports)).append("\n");
sb.append(" abuseReportsCount: ").append(toIndentedString(abuseReportsCount)).append("\n");
sb.append(" messageIDs: ").append(toIndentedString(messageIDs)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy