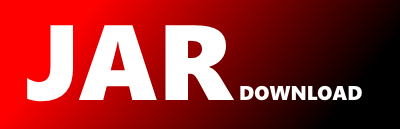
io.swagger.client.model.EmailMergeMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import io.swagger.client.model.Body;
import io.swagger.client.model.EncodingSettings;
import io.swagger.client.model.MergeRecipient;
import io.swagger.client.model.MessageAttachment;
import io.swagger.client.model.Options;
import io.swagger.client.model.Sender;
import io.swagger.client.model.TrackingOptions;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* EmailMergeMessage
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class EmailMergeMessage {
@SerializedName("Recipients")
private MergeRecipient recipients = null;
@SerializedName("Body")
private Body body = null;
@SerializedName("Sender")
private Sender sender = null;
@SerializedName("TemplateName")
private String templateName = null;
@SerializedName("Attachments")
private List attachments = new ArrayList();
@SerializedName("Tracking")
private TrackingOptions tracking = null;
@SerializedName("MergeFields")
private Map mergeFields = new HashMap();
@SerializedName("Headers")
private Map headers = new HashMap();
@SerializedName("Postback")
private String postback = null;
@SerializedName("Encoding")
private EncodingSettings encoding = null;
@SerializedName("Options")
private Options options = null;
public EmailMergeMessage recipients(MergeRecipient recipients) {
this.recipients = recipients;
return this;
}
/**
* Get recipients
* @return recipients
**/
@ApiModelProperty(required = true, value = "")
public MergeRecipient getRecipients() {
return recipients;
}
public void setRecipients(MergeRecipient recipients) {
this.recipients = recipients;
}
public EmailMergeMessage body(Body body) {
this.body = body;
return this;
}
/**
* Get body
* @return body
**/
@ApiModelProperty(required = true, value = "")
public Body getBody() {
return body;
}
public void setBody(Body body) {
this.body = body;
}
public EmailMergeMessage sender(Sender sender) {
this.sender = sender;
return this;
}
/**
* Get sender
* @return sender
**/
@ApiModelProperty(required = true, value = "")
public Sender getSender() {
return sender;
}
public void setSender(Sender sender) {
this.sender = sender;
}
public EmailMergeMessage templateName(String templateName) {
this.templateName = templateName;
return this;
}
/**
* Name of template.
* @return templateName
**/
@ApiModelProperty(example = "Template01", required = true, value = "Name of template.")
public String getTemplateName() {
return templateName;
}
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
public EmailMergeMessage attachments(List attachments) {
this.attachments = attachments;
return this;
}
public EmailMergeMessage addAttachmentsItem(MessageAttachment attachmentsItem) {
this.attachments.add(attachmentsItem);
return this;
}
/**
* Attachment files. These files should be provided with the POST multipart file upload, not directly in the request's URL. Should also include merge CSV file
* @return attachments
**/
@ApiModelProperty(required = true, value = "Attachment files. These files should be provided with the POST multipart file upload, not directly in the request's URL. Should also include merge CSV file")
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public EmailMergeMessage tracking(TrackingOptions tracking) {
this.tracking = tracking;
return this;
}
/**
* Get tracking
* @return tracking
**/
@ApiModelProperty(required = true, value = "")
public TrackingOptions getTracking() {
return tracking;
}
public void setTracking(TrackingOptions tracking) {
this.tracking = tracking;
}
public EmailMergeMessage mergeFields(Map mergeFields) {
this.mergeFields = mergeFields;
return this;
}
public EmailMergeMessage putMergeFieldsItem(String key, String mergeFieldsItem) {
this.mergeFields.put(key, mergeFieldsItem);
return this;
}
/**
* Get mergeFields
* @return mergeFields
**/
@ApiModelProperty(required = true, value = "")
public Map getMergeFields() {
return mergeFields;
}
public void setMergeFields(Map mergeFields) {
this.mergeFields = mergeFields;
}
public EmailMergeMessage headers(Map headers) {
this.headers = headers;
return this;
}
public EmailMergeMessage putHeadersItem(String key, String headersItem) {
this.headers.put(key, headersItem);
return this;
}
/**
* Get headers
* @return headers
**/
@ApiModelProperty(required = true, value = "")
public Map getHeaders() {
return headers;
}
public void setHeaders(Map headers) {
this.headers = headers;
}
public EmailMergeMessage postback(String postback) {
this.postback = postback;
return this;
}
/**
* Get postback
* @return postback
**/
@ApiModelProperty(required = true, value = "")
public String getPostback() {
return postback;
}
public void setPostback(String postback) {
this.postback = postback;
}
public EmailMergeMessage encoding(EncodingSettings encoding) {
this.encoding = encoding;
return this;
}
/**
* Get encoding
* @return encoding
**/
@ApiModelProperty(required = true, value = "")
public EncodingSettings getEncoding() {
return encoding;
}
public void setEncoding(EncodingSettings encoding) {
this.encoding = encoding;
}
public EmailMergeMessage options(Options options) {
this.options = options;
return this;
}
/**
* Get options
* @return options
**/
@ApiModelProperty(required = true, value = "")
public Options getOptions() {
return options;
}
public void setOptions(Options options) {
this.options = options;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailMergeMessage emailMergeMessage = (EmailMergeMessage) o;
return Objects.equals(this.recipients, emailMergeMessage.recipients) &&
Objects.equals(this.body, emailMergeMessage.body) &&
Objects.equals(this.sender, emailMergeMessage.sender) &&
Objects.equals(this.templateName, emailMergeMessage.templateName) &&
Objects.equals(this.attachments, emailMergeMessage.attachments) &&
Objects.equals(this.tracking, emailMergeMessage.tracking) &&
Objects.equals(this.mergeFields, emailMergeMessage.mergeFields) &&
Objects.equals(this.headers, emailMergeMessage.headers) &&
Objects.equals(this.postback, emailMergeMessage.postback) &&
Objects.equals(this.encoding, emailMergeMessage.encoding) &&
Objects.equals(this.options, emailMergeMessage.options);
}
@Override
public int hashCode() {
return Objects.hash(recipients, body, sender, templateName, attachments, tracking, mergeFields, headers, postback, encoding, options);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class EmailMergeMessage {\n");
sb.append(" recipients: ").append(toIndentedString(recipients)).append("\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" sender: ").append(toIndentedString(sender)).append("\n");
sb.append(" templateName: ").append(toIndentedString(templateName)).append("\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" tracking: ").append(toIndentedString(tracking)).append("\n");
sb.append(" mergeFields: ").append(toIndentedString(mergeFields)).append("\n");
sb.append(" headers: ").append(toIndentedString(headers)).append("\n");
sb.append(" postback: ").append(toIndentedString(postback)).append("\n");
sb.append(" encoding: ").append(toIndentedString(encoding)).append("\n");
sb.append(" options: ").append(toIndentedString(options)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy