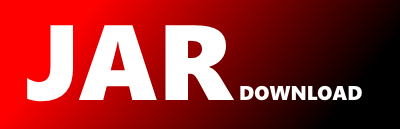
io.swagger.client.model.Recipient Maven / Gradle / Ivy
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Detailed information about message recipient
*/
@ApiModel(description = "Detailed information about message recipient")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class Recipient {
@SerializedName("IsSms")
private Boolean isSms = null;
@SerializedName("MsgID")
private String msgID = null;
@SerializedName("To")
private String to = null;
@SerializedName("Status")
private String status = null;
@SerializedName("Channel")
private String channel = null;
@SerializedName("Date")
private String date = null;
@SerializedName("DateSent")
private String dateSent = null;
@SerializedName("DateOpened")
private String dateOpened = null;
@SerializedName("DateClicked")
private String dateClicked = null;
@SerializedName("Message")
private String message = null;
@SerializedName("ShowCategory")
private Boolean showCategory = null;
@SerializedName("MessageCategory")
private String messageCategory = null;
/**
* ID of message category
*/
@JsonAdapter(MessageCategoryIDEnum.Adapter.class)
public enum MessageCategoryIDEnum {
UNKNOWN("Unknown"),
IGNORE("Ignore"),
SPAM("Spam"),
BLACKLISTED("BlackListed"),
NOMAILBOX("NoMailbox"),
GREYLISTED("GreyListed"),
THROTTLED("Throttled"),
TIMEOUT("Timeout"),
CONNECTIONPROBLEM("ConnectionProblem"),
SPFPROBLEM("SPFProblem"),
ACCOUNTPROBLEM("AccountProblem"),
DNSPROBLEM("DNSProblem"),
NOTDELIVEREDCANCELLED("NotDeliveredCancelled"),
CODEERROR("CodeError"),
MANUALCANCEL("ManualCancel"),
CONNECTIONTERMINATED("ConnectionTerminated"),
NOTDELIVERED("NotDelivered");
private String value;
MessageCategoryIDEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MessageCategoryIDEnum fromValue(String text) {
for (MessageCategoryIDEnum b : MessageCategoryIDEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MessageCategoryIDEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MessageCategoryIDEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MessageCategoryIDEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("MessageCategoryID")
private MessageCategoryIDEnum messageCategoryID = null;
@SerializedName("StatusChangeDate")
private String statusChangeDate = null;
@SerializedName("NextTryOn")
private String nextTryOn = null;
@SerializedName("Subject")
private String subject = null;
@SerializedName("FromEmail")
private String fromEmail = null;
@SerializedName("EnvelopeFrom")
private String envelopeFrom = null;
@SerializedName("JobID")
private String jobID = null;
@SerializedName("SmsUpdateRequired")
private Boolean smsUpdateRequired = null;
@SerializedName("TextMessage")
private String textMessage = null;
@SerializedName("MessageSid")
private String messageSid = null;
@SerializedName("ContactLastError")
private String contactLastError = null;
@SerializedName("IPAddress")
private String ipAddress = null;
public Recipient isSms(Boolean isSms) {
this.isSms = isSms;
return this;
}
/**
* True, if message is SMS. Otherwise, false
* @return isSms
**/
@ApiModelProperty(example = "true", required = true, value = "True, if message is SMS. Otherwise, false")
public Boolean isIsSms() {
return isSms;
}
public void setIsSms(Boolean isSms) {
this.isSms = isSms;
}
public Recipient msgID(String msgID) {
this.msgID = msgID;
return this;
}
/**
* ID number of selected message.
* @return msgID
**/
@ApiModelProperty(example = "ABCDE_9RPhSWiaJq_ab1g1", required = true, value = "ID number of selected message.")
public String getMsgID() {
return msgID;
}
public void setMsgID(String msgID) {
this.msgID = msgID;
}
public Recipient to(String to) {
this.to = to;
return this;
}
/**
* Ending date for search in YYYY-MM-DDThh:mm:ss format.
* @return to
**/
@ApiModelProperty(example = "2001-01-01T01:01:01", required = true, value = "Ending date for search in YYYY-MM-DDThh:mm:ss format.")
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public Recipient status(String status) {
this.status = status;
return this;
}
/**
* Name of recipient's status: Submitted, ReadyToSend, WaitingToRetry, Sending, Bounced, Sent, Opened, Clicked, Unsubscribed, AbuseReport
* @return status
**/
@ApiModelProperty(required = true, value = "Name of recipient's status: Submitted, ReadyToSend, WaitingToRetry, Sending, Bounced, Sent, Opened, Clicked, Unsubscribed, AbuseReport")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Recipient channel(String channel) {
this.channel = channel;
return this;
}
/**
* Name of selected Channel.
* @return channel
**/
@ApiModelProperty(example = "Channel01", required = true, value = "Name of selected Channel.")
public String getChannel() {
return channel;
}
public void setChannel(String channel) {
this.channel = channel;
}
public Recipient date(String date) {
this.date = date;
return this;
}
/**
* Creation date
* @return date
**/
@ApiModelProperty(required = true, value = "Creation date")
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public Recipient dateSent(String dateSent) {
this.dateSent = dateSent;
return this;
}
/**
* Date when the email was sent
* @return dateSent
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date when the email was sent")
public String getDateSent() {
return dateSent;
}
public void setDateSent(String dateSent) {
this.dateSent = dateSent;
}
public Recipient dateOpened(String dateOpened) {
this.dateOpened = dateOpened;
return this;
}
/**
* Date when the email changed the status to 'opened'
* @return dateOpened
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date when the email changed the status to 'opened'")
public String getDateOpened() {
return dateOpened;
}
public void setDateOpened(String dateOpened) {
this.dateOpened = dateOpened;
}
public Recipient dateClicked(String dateClicked) {
this.dateClicked = dateClicked;
return this;
}
/**
* Date when the email changed the status to 'clicked'
* @return dateClicked
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date when the email changed the status to 'clicked'")
public String getDateClicked() {
return dateClicked;
}
public void setDateClicked(String dateClicked) {
this.dateClicked = dateClicked;
}
public Recipient message(String message) {
this.message = message;
return this;
}
/**
* Content of message, HTML encoded
* @return message
**/
@ApiModelProperty(example = "Lorem ipsum", required = true, value = "Content of message, HTML encoded")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Recipient showCategory(Boolean showCategory) {
this.showCategory = showCategory;
return this;
}
/**
* True, if message category should be shown. Otherwise, false
* @return showCategory
**/
@ApiModelProperty(example = "true", required = true, value = "True, if message category should be shown. Otherwise, false")
public Boolean isShowCategory() {
return showCategory;
}
public void setShowCategory(Boolean showCategory) {
this.showCategory = showCategory;
}
public Recipient messageCategory(String messageCategory) {
this.messageCategory = messageCategory;
return this;
}
/**
* Name of message category
* @return messageCategory
**/
@ApiModelProperty(required = true, value = "Name of message category")
public String getMessageCategory() {
return messageCategory;
}
public void setMessageCategory(String messageCategory) {
this.messageCategory = messageCategory;
}
public Recipient messageCategoryID(MessageCategoryIDEnum messageCategoryID) {
this.messageCategoryID = messageCategoryID;
return this;
}
/**
* ID of message category
* @return messageCategoryID
**/
@ApiModelProperty(value = "ID of message category")
public MessageCategoryIDEnum getMessageCategoryID() {
return messageCategoryID;
}
public void setMessageCategoryID(MessageCategoryIDEnum messageCategoryID) {
this.messageCategoryID = messageCategoryID;
}
public Recipient statusChangeDate(String statusChangeDate) {
this.statusChangeDate = statusChangeDate;
return this;
}
/**
* Date of last status change.
* @return statusChangeDate
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date of last status change.")
public String getStatusChangeDate() {
return statusChangeDate;
}
public void setStatusChangeDate(String statusChangeDate) {
this.statusChangeDate = statusChangeDate;
}
public Recipient nextTryOn(String nextTryOn) {
this.nextTryOn = nextTryOn;
return this;
}
/**
* Date of next try
* @return nextTryOn
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", required = true, value = "Date of next try")
public String getNextTryOn() {
return nextTryOn;
}
public void setNextTryOn(String nextTryOn) {
this.nextTryOn = nextTryOn;
}
public Recipient subject(String subject) {
this.subject = subject;
return this;
}
/**
* Default subject of email.
* @return subject
**/
@ApiModelProperty(example = "Hello!", required = true, value = "Default subject of email.")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public Recipient fromEmail(String fromEmail) {
this.fromEmail = fromEmail;
return this;
}
/**
* Default From: email address.
* @return fromEmail
**/
@ApiModelProperty(example = "[email protected]", required = true, value = "Default From: email address.")
public String getFromEmail() {
return fromEmail;
}
public void setFromEmail(String fromEmail) {
this.fromEmail = fromEmail;
}
public Recipient envelopeFrom(String envelopeFrom) {
this.envelopeFrom = envelopeFrom;
return this;
}
/**
* Get envelopeFrom
* @return envelopeFrom
**/
@ApiModelProperty(required = true, value = "")
public String getEnvelopeFrom() {
return envelopeFrom;
}
public void setEnvelopeFrom(String envelopeFrom) {
this.envelopeFrom = envelopeFrom;
}
public Recipient jobID(String jobID) {
this.jobID = jobID;
return this;
}
/**
* ID of certain mail job
* @return jobID
**/
@ApiModelProperty(required = true, value = "ID of certain mail job")
public String getJobID() {
return jobID;
}
public void setJobID(String jobID) {
this.jobID = jobID;
}
public Recipient smsUpdateRequired(Boolean smsUpdateRequired) {
this.smsUpdateRequired = smsUpdateRequired;
return this;
}
/**
* True, if message is a SMS and status is not yet confirmed. Otherwise, false
* @return smsUpdateRequired
**/
@ApiModelProperty(example = "false", required = true, value = "True, if message is a SMS and status is not yet confirmed. Otherwise, false")
public Boolean isSmsUpdateRequired() {
return smsUpdateRequired;
}
public void setSmsUpdateRequired(Boolean smsUpdateRequired) {
this.smsUpdateRequired = smsUpdateRequired;
}
public Recipient textMessage(String textMessage) {
this.textMessage = textMessage;
return this;
}
/**
* Content of message
* @return textMessage
**/
@ApiModelProperty(example = "Lorem ipsum", required = true, value = "Content of message")
public String getTextMessage() {
return textMessage;
}
public void setTextMessage(String textMessage) {
this.textMessage = textMessage;
}
public Recipient messageSid(String messageSid) {
this.messageSid = messageSid;
return this;
}
/**
* Comma separated ID numbers of messages.
* @return messageSid
**/
@ApiModelProperty(example = "12345678,1234567890", required = true, value = "Comma separated ID numbers of messages.")
public String getMessageSid() {
return messageSid;
}
public void setMessageSid(String messageSid) {
this.messageSid = messageSid;
}
public Recipient contactLastError(String contactLastError) {
this.contactLastError = contactLastError;
return this;
}
/**
* Recipient's last bounce error because of which this e-mail was suppressed
* @return contactLastError
**/
@ApiModelProperty(example = "The email address does not exist", required = true, value = "Recipient's last bounce error because of which this e-mail was suppressed")
public String getContactLastError() {
return contactLastError;
}
public void setContactLastError(String contactLastError) {
this.contactLastError = contactLastError;
}
public Recipient ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* Get ipAddress
* @return ipAddress
**/
@ApiModelProperty(required = true, value = "")
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Recipient recipient = (Recipient) o;
return Objects.equals(this.isSms, recipient.isSms) &&
Objects.equals(this.msgID, recipient.msgID) &&
Objects.equals(this.to, recipient.to) &&
Objects.equals(this.status, recipient.status) &&
Objects.equals(this.channel, recipient.channel) &&
Objects.equals(this.date, recipient.date) &&
Objects.equals(this.dateSent, recipient.dateSent) &&
Objects.equals(this.dateOpened, recipient.dateOpened) &&
Objects.equals(this.dateClicked, recipient.dateClicked) &&
Objects.equals(this.message, recipient.message) &&
Objects.equals(this.showCategory, recipient.showCategory) &&
Objects.equals(this.messageCategory, recipient.messageCategory) &&
Objects.equals(this.messageCategoryID, recipient.messageCategoryID) &&
Objects.equals(this.statusChangeDate, recipient.statusChangeDate) &&
Objects.equals(this.nextTryOn, recipient.nextTryOn) &&
Objects.equals(this.subject, recipient.subject) &&
Objects.equals(this.fromEmail, recipient.fromEmail) &&
Objects.equals(this.envelopeFrom, recipient.envelopeFrom) &&
Objects.equals(this.jobID, recipient.jobID) &&
Objects.equals(this.smsUpdateRequired, recipient.smsUpdateRequired) &&
Objects.equals(this.textMessage, recipient.textMessage) &&
Objects.equals(this.messageSid, recipient.messageSid) &&
Objects.equals(this.contactLastError, recipient.contactLastError) &&
Objects.equals(this.ipAddress, recipient.ipAddress);
}
@Override
public int hashCode() {
return Objects.hash(isSms, msgID, to, status, channel, date, dateSent, dateOpened, dateClicked, message, showCategory, messageCategory, messageCategoryID, statusChangeDate, nextTryOn, subject, fromEmail, envelopeFrom, jobID, smsUpdateRequired, textMessage, messageSid, contactLastError, ipAddress);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Recipient {\n");
sb.append(" isSms: ").append(toIndentedString(isSms)).append("\n");
sb.append(" msgID: ").append(toIndentedString(msgID)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" channel: ").append(toIndentedString(channel)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" dateSent: ").append(toIndentedString(dateSent)).append("\n");
sb.append(" dateOpened: ").append(toIndentedString(dateOpened)).append("\n");
sb.append(" dateClicked: ").append(toIndentedString(dateClicked)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" showCategory: ").append(toIndentedString(showCategory)).append("\n");
sb.append(" messageCategory: ").append(toIndentedString(messageCategory)).append("\n");
sb.append(" messageCategoryID: ").append(toIndentedString(messageCategoryID)).append("\n");
sb.append(" statusChangeDate: ").append(toIndentedString(statusChangeDate)).append("\n");
sb.append(" nextTryOn: ").append(toIndentedString(nextTryOn)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" fromEmail: ").append(toIndentedString(fromEmail)).append("\n");
sb.append(" envelopeFrom: ").append(toIndentedString(envelopeFrom)).append("\n");
sb.append(" jobID: ").append(toIndentedString(jobID)).append("\n");
sb.append(" smsUpdateRequired: ").append(toIndentedString(smsUpdateRequired)).append("\n");
sb.append(" textMessage: ").append(toIndentedString(textMessage)).append("\n");
sb.append(" messageSid: ").append(toIndentedString(messageSid)).append("\n");
sb.append(" contactLastError: ").append(toIndentedString(contactLastError)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy