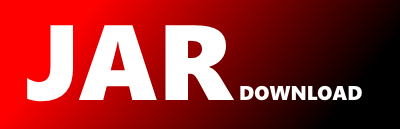
io.swagger.client.model.SubAccountSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Detailed account settings.
*/
@ApiModel(description = "Detailed account settings.")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class SubAccountSettings {
@SerializedName("Email")
private String email = null;
@SerializedName("RequiresEmailCredits")
private Boolean requiresEmailCredits = null;
@SerializedName("MonthlyRefillCredits")
private Double monthlyRefillCredits = null;
@SerializedName("EmailSizeLimit")
private Integer emailSizeLimit = null;
@SerializedName("DailySendLimit")
private Integer dailySendLimit = null;
@SerializedName("MaxContacts")
private Integer maxContacts = null;
@SerializedName("EnablePrivateIPRequest")
private Boolean enablePrivateIPRequest = null;
@SerializedName("EnableContactFeatures")
private Boolean enableContactFeatures = null;
/**
* Sending permission setting for account
*/
@JsonAdapter(SendingPermissionEnum.Adapter.class)
public enum SendingPermissionEnum {
NONE("None"),
SMTP("Smtp"),
HTTPAPI("HttpApi"),
SMTPANDHTTPAPI("SmtpAndHttpApi"),
INTERFACE("Interface"),
SMTPANDINTERFACE("SmtpAndInterface"),
HTTPAPIANDINTERFACE("HttpApiAndInterface"),
USEACCESSLEVEL("UseAccessLevel"),
ALL("All");
private String value;
SendingPermissionEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static SendingPermissionEnum fromValue(String text) {
for (SendingPermissionEnum b : SendingPermissionEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final SendingPermissionEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public SendingPermissionEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return SendingPermissionEnum.fromValue(String.valueOf(value));
}
}
}
@SerializedName("SendingPermission")
private SendingPermissionEnum sendingPermission = null;
@SerializedName("PoolName")
private String poolName = null;
@SerializedName("PublicAccountID")
private String publicAccountID = null;
@SerializedName("Allow2FA")
private Boolean allow2FA = null;
public SubAccountSettings email(String email) {
this.email = email;
return this;
}
/**
* Proper email address.
* @return email
**/
@ApiModelProperty(example = "[email protected]", required = true, value = "Proper email address.")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public SubAccountSettings requiresEmailCredits(Boolean requiresEmailCredits) {
this.requiresEmailCredits = requiresEmailCredits;
return this;
}
/**
* True, if account needs credits to send emails. Otherwise, false
* @return requiresEmailCredits
**/
@ApiModelProperty(example = "true", required = true, value = "True, if account needs credits to send emails. Otherwise, false")
public Boolean isRequiresEmailCredits() {
return requiresEmailCredits;
}
public void setRequiresEmailCredits(Boolean requiresEmailCredits) {
this.requiresEmailCredits = requiresEmailCredits;
}
public SubAccountSettings monthlyRefillCredits(Double monthlyRefillCredits) {
this.monthlyRefillCredits = monthlyRefillCredits;
return this;
}
/**
* Amount of credits added to account automatically
* @return monthlyRefillCredits
**/
@ApiModelProperty(example = "1000.0", required = true, value = "Amount of credits added to account automatically")
public Double getMonthlyRefillCredits() {
return monthlyRefillCredits;
}
public void setMonthlyRefillCredits(Double monthlyRefillCredits) {
this.monthlyRefillCredits = monthlyRefillCredits;
}
public SubAccountSettings emailSizeLimit(Integer emailSizeLimit) {
this.emailSizeLimit = emailSizeLimit;
return this;
}
/**
* Maximum size of email including attachments in MB's
* @return emailSizeLimit
**/
@ApiModelProperty(example = "10", required = true, value = "Maximum size of email including attachments in MB's")
public Integer getEmailSizeLimit() {
return emailSizeLimit;
}
public void setEmailSizeLimit(Integer emailSizeLimit) {
this.emailSizeLimit = emailSizeLimit;
}
public SubAccountSettings dailySendLimit(Integer dailySendLimit) {
this.dailySendLimit = dailySendLimit;
return this;
}
/**
* Amount of emails account can send daily
* @return dailySendLimit
**/
@ApiModelProperty(example = "100000", required = true, value = "Amount of emails account can send daily")
public Integer getDailySendLimit() {
return dailySendLimit;
}
public void setDailySendLimit(Integer dailySendLimit) {
this.dailySendLimit = dailySendLimit;
}
public SubAccountSettings maxContacts(Integer maxContacts) {
this.maxContacts = maxContacts;
return this;
}
/**
* Maximum number of contacts the account can have
* @return maxContacts
**/
@ApiModelProperty(example = "100000", required = true, value = "Maximum number of contacts the account can have")
public Integer getMaxContacts() {
return maxContacts;
}
public void setMaxContacts(Integer maxContacts) {
this.maxContacts = maxContacts;
}
public SubAccountSettings enablePrivateIPRequest(Boolean enablePrivateIPRequest) {
this.enablePrivateIPRequest = enablePrivateIPRequest;
return this;
}
/**
* True, if account can request for private IP on its own. Otherwise, false
* @return enablePrivateIPRequest
**/
@ApiModelProperty(example = "true", required = true, value = "True, if account can request for private IP on its own. Otherwise, false")
public Boolean isEnablePrivateIPRequest() {
return enablePrivateIPRequest;
}
public void setEnablePrivateIPRequest(Boolean enablePrivateIPRequest) {
this.enablePrivateIPRequest = enablePrivateIPRequest;
}
public SubAccountSettings enableContactFeatures(Boolean enableContactFeatures) {
this.enableContactFeatures = enableContactFeatures;
return this;
}
/**
* True, if you want to use Contact Delivery Tools. Otherwise, false
* @return enableContactFeatures
**/
@ApiModelProperty(required = true, value = "True, if you want to use Contact Delivery Tools. Otherwise, false")
public Boolean isEnableContactFeatures() {
return enableContactFeatures;
}
public void setEnableContactFeatures(Boolean enableContactFeatures) {
this.enableContactFeatures = enableContactFeatures;
}
public SubAccountSettings sendingPermission(SendingPermissionEnum sendingPermission) {
this.sendingPermission = sendingPermission;
return this;
}
/**
* Sending permission setting for account
* @return sendingPermission
**/
@ApiModelProperty(example = "All", required = true, value = "Sending permission setting for account")
public SendingPermissionEnum getSendingPermission() {
return sendingPermission;
}
public void setSendingPermission(SendingPermissionEnum sendingPermission) {
this.sendingPermission = sendingPermission;
}
public SubAccountSettings poolName(String poolName) {
this.poolName = poolName;
return this;
}
/**
* Name of your custom IP Pool to be used in the sending process
* @return poolName
**/
@ApiModelProperty(example = "My Custom Pool", required = true, value = "Name of your custom IP Pool to be used in the sending process")
public String getPoolName() {
return poolName;
}
public void setPoolName(String poolName) {
this.poolName = poolName;
}
public SubAccountSettings publicAccountID(String publicAccountID) {
this.publicAccountID = publicAccountID;
return this;
}
/**
* Public key for limited access to your account such as contact/add so you can use it safely on public websites.
* @return publicAccountID
**/
@ApiModelProperty(example = "EB3EBB7A-C20D-4D39-8F2F-5E6842F58E6F", required = true, value = "Public key for limited access to your account such as contact/add so you can use it safely on public websites.")
public String getPublicAccountID() {
return publicAccountID;
}
public void setPublicAccountID(String publicAccountID) {
this.publicAccountID = publicAccountID;
}
public SubAccountSettings allow2FA(Boolean allow2FA) {
this.allow2FA = allow2FA;
return this;
}
/**
* Get allow2FA
* @return allow2FA
**/
@ApiModelProperty(example = "false", value = "")
public Boolean isAllow2FA() {
return allow2FA;
}
public void setAllow2FA(Boolean allow2FA) {
this.allow2FA = allow2FA;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubAccountSettings subAccountSettings = (SubAccountSettings) o;
return Objects.equals(this.email, subAccountSettings.email) &&
Objects.equals(this.requiresEmailCredits, subAccountSettings.requiresEmailCredits) &&
Objects.equals(this.monthlyRefillCredits, subAccountSettings.monthlyRefillCredits) &&
Objects.equals(this.emailSizeLimit, subAccountSettings.emailSizeLimit) &&
Objects.equals(this.dailySendLimit, subAccountSettings.dailySendLimit) &&
Objects.equals(this.maxContacts, subAccountSettings.maxContacts) &&
Objects.equals(this.enablePrivateIPRequest, subAccountSettings.enablePrivateIPRequest) &&
Objects.equals(this.enableContactFeatures, subAccountSettings.enableContactFeatures) &&
Objects.equals(this.sendingPermission, subAccountSettings.sendingPermission) &&
Objects.equals(this.poolName, subAccountSettings.poolName) &&
Objects.equals(this.publicAccountID, subAccountSettings.publicAccountID) &&
Objects.equals(this.allow2FA, subAccountSettings.allow2FA);
}
@Override
public int hashCode() {
return Objects.hash(email, requiresEmailCredits, monthlyRefillCredits, emailSizeLimit, dailySendLimit, maxContacts, enablePrivateIPRequest, enableContactFeatures, sendingPermission, poolName, publicAccountID, allow2FA);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubAccountSettings {\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" requiresEmailCredits: ").append(toIndentedString(requiresEmailCredits)).append("\n");
sb.append(" monthlyRefillCredits: ").append(toIndentedString(monthlyRefillCredits)).append("\n");
sb.append(" emailSizeLimit: ").append(toIndentedString(emailSizeLimit)).append("\n");
sb.append(" dailySendLimit: ").append(toIndentedString(dailySendLimit)).append("\n");
sb.append(" maxContacts: ").append(toIndentedString(maxContacts)).append("\n");
sb.append(" enablePrivateIPRequest: ").append(toIndentedString(enablePrivateIPRequest)).append("\n");
sb.append(" enableContactFeatures: ").append(toIndentedString(enableContactFeatures)).append("\n");
sb.append(" sendingPermission: ").append(toIndentedString(sendingPermission)).append("\n");
sb.append(" poolName: ").append(toIndentedString(poolName)).append("\n");
sb.append(" publicAccountID: ").append(toIndentedString(publicAccountID)).append("\n");
sb.append(" allow2FA: ").append(toIndentedString(allow2FA)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy