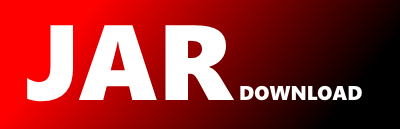
io.swagger.client.model.Webhook Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticemail-RESTful-API Show documentation
Show all versions of elasticemail-RESTful-API Show documentation
Send your emails with ElasticEmail API
The newest version!
/*
* elasticemail_Restful_api
* Send your emails with ElasticEmail API
*
* OpenAPI spec version: 3.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import org.threeten.bp.OffsetDateTime;
/**
* Notification webhook setting
*/
@ApiModel(description = "Notification webhook setting")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2019-03-29T15:32:30.861Z")
public class Webhook {
@SerializedName("WebhookID")
private String webhookID = null;
@SerializedName("Name")
private String name = null;
@SerializedName("DateCreated")
private OffsetDateTime dateCreated = null;
@SerializedName("DateUpdated")
private OffsetDateTime dateUpdated = null;
@SerializedName("URL")
private String URL = null;
@SerializedName("NotifyOncePerEmail")
private Boolean notifyOncePerEmail = null;
@SerializedName("NotificationForSent")
private Boolean notificationForSent = null;
@SerializedName("NotificationForOpened")
private Boolean notificationForOpened = null;
@SerializedName("NotificationForClicked")
private Boolean notificationForClicked = null;
@SerializedName("NotificationForUnsubscribed")
private Boolean notificationForUnsubscribed = null;
@SerializedName("NotificationForAbuseReport")
private Boolean notificationForAbuseReport = null;
@SerializedName("NotificationForError")
private Boolean notificationForError = null;
public Webhook webhookID(String webhookID) {
this.webhookID = webhookID;
return this;
}
/**
* Public webhook ID
* @return webhookID
**/
@ApiModelProperty(required = true, value = "Public webhook ID")
public String getWebhookID() {
return webhookID;
}
public void setWebhookID(String webhookID) {
this.webhookID = webhookID;
}
public Webhook name(String name) {
this.name = name;
return this;
}
/**
* Filename
* @return name
**/
@ApiModelProperty(example = "attachment.txt", required = true, value = "Filename")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Webhook dateCreated(OffsetDateTime dateCreated) {
this.dateCreated = dateCreated;
return this;
}
/**
* Creation date.
* @return dateCreated
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", value = "Creation date.")
public OffsetDateTime getDateCreated() {
return dateCreated;
}
public void setDateCreated(OffsetDateTime dateCreated) {
this.dateCreated = dateCreated;
}
public Webhook dateUpdated(OffsetDateTime dateUpdated) {
this.dateUpdated = dateUpdated;
return this;
}
/**
* Last change date
* @return dateUpdated
**/
@ApiModelProperty(example = "2001-01-01T12:00:00", value = "Last change date")
public OffsetDateTime getDateUpdated() {
return dateUpdated;
}
public void setDateUpdated(OffsetDateTime dateUpdated) {
this.dateUpdated = dateUpdated;
}
public Webhook URL(String URL) {
this.URL = URL;
return this;
}
/**
* URL of notification.
* @return URL
**/
@ApiModelProperty(example = "http://address.for.notification.com", required = true, value = "URL of notification.")
public String getURL() {
return URL;
}
public void setURL(String URL) {
this.URL = URL;
}
public Webhook notifyOncePerEmail(Boolean notifyOncePerEmail) {
this.notifyOncePerEmail = notifyOncePerEmail;
return this;
}
/**
* Get notifyOncePerEmail
* @return notifyOncePerEmail
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotifyOncePerEmail() {
return notifyOncePerEmail;
}
public void setNotifyOncePerEmail(Boolean notifyOncePerEmail) {
this.notifyOncePerEmail = notifyOncePerEmail;
}
public Webhook notificationForSent(Boolean notificationForSent) {
this.notificationForSent = notificationForSent;
return this;
}
/**
* Get notificationForSent
* @return notificationForSent
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForSent() {
return notificationForSent;
}
public void setNotificationForSent(Boolean notificationForSent) {
this.notificationForSent = notificationForSent;
}
public Webhook notificationForOpened(Boolean notificationForOpened) {
this.notificationForOpened = notificationForOpened;
return this;
}
/**
* Get notificationForOpened
* @return notificationForOpened
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForOpened() {
return notificationForOpened;
}
public void setNotificationForOpened(Boolean notificationForOpened) {
this.notificationForOpened = notificationForOpened;
}
public Webhook notificationForClicked(Boolean notificationForClicked) {
this.notificationForClicked = notificationForClicked;
return this;
}
/**
* Get notificationForClicked
* @return notificationForClicked
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForClicked() {
return notificationForClicked;
}
public void setNotificationForClicked(Boolean notificationForClicked) {
this.notificationForClicked = notificationForClicked;
}
public Webhook notificationForUnsubscribed(Boolean notificationForUnsubscribed) {
this.notificationForUnsubscribed = notificationForUnsubscribed;
return this;
}
/**
* Get notificationForUnsubscribed
* @return notificationForUnsubscribed
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForUnsubscribed() {
return notificationForUnsubscribed;
}
public void setNotificationForUnsubscribed(Boolean notificationForUnsubscribed) {
this.notificationForUnsubscribed = notificationForUnsubscribed;
}
public Webhook notificationForAbuseReport(Boolean notificationForAbuseReport) {
this.notificationForAbuseReport = notificationForAbuseReport;
return this;
}
/**
* Get notificationForAbuseReport
* @return notificationForAbuseReport
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForAbuseReport() {
return notificationForAbuseReport;
}
public void setNotificationForAbuseReport(Boolean notificationForAbuseReport) {
this.notificationForAbuseReport = notificationForAbuseReport;
}
public Webhook notificationForError(Boolean notificationForError) {
this.notificationForError = notificationForError;
return this;
}
/**
* Get notificationForError
* @return notificationForError
**/
@ApiModelProperty(required = true, value = "")
public Boolean isNotificationForError() {
return notificationForError;
}
public void setNotificationForError(Boolean notificationForError) {
this.notificationForError = notificationForError;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Webhook webhook = (Webhook) o;
return Objects.equals(this.webhookID, webhook.webhookID) &&
Objects.equals(this.name, webhook.name) &&
Objects.equals(this.dateCreated, webhook.dateCreated) &&
Objects.equals(this.dateUpdated, webhook.dateUpdated) &&
Objects.equals(this.URL, webhook.URL) &&
Objects.equals(this.notifyOncePerEmail, webhook.notifyOncePerEmail) &&
Objects.equals(this.notificationForSent, webhook.notificationForSent) &&
Objects.equals(this.notificationForOpened, webhook.notificationForOpened) &&
Objects.equals(this.notificationForClicked, webhook.notificationForClicked) &&
Objects.equals(this.notificationForUnsubscribed, webhook.notificationForUnsubscribed) &&
Objects.equals(this.notificationForAbuseReport, webhook.notificationForAbuseReport) &&
Objects.equals(this.notificationForError, webhook.notificationForError);
}
@Override
public int hashCode() {
return Objects.hash(webhookID, name, dateCreated, dateUpdated, URL, notifyOncePerEmail, notificationForSent, notificationForOpened, notificationForClicked, notificationForUnsubscribed, notificationForAbuseReport, notificationForError);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Webhook {\n");
sb.append(" webhookID: ").append(toIndentedString(webhookID)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" dateCreated: ").append(toIndentedString(dateCreated)).append("\n");
sb.append(" dateUpdated: ").append(toIndentedString(dateUpdated)).append("\n");
sb.append(" URL: ").append(toIndentedString(URL)).append("\n");
sb.append(" notifyOncePerEmail: ").append(toIndentedString(notifyOncePerEmail)).append("\n");
sb.append(" notificationForSent: ").append(toIndentedString(notificationForSent)).append("\n");
sb.append(" notificationForOpened: ").append(toIndentedString(notificationForOpened)).append("\n");
sb.append(" notificationForClicked: ").append(toIndentedString(notificationForClicked)).append("\n");
sb.append(" notificationForUnsubscribed: ").append(toIndentedString(notificationForUnsubscribed)).append("\n");
sb.append(" notificationForAbuseReport: ").append(toIndentedString(notificationForAbuseReport)).append("\n");
sb.append(" notificationForError: ").append(toIndentedString(notificationForError)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy