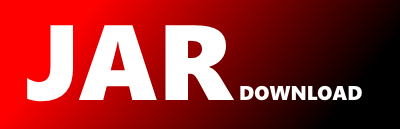
com.elastisys.scale.cloudpool.aws.commons.requests.ec2.GetSpotInstanceRequests Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudpool.aws.commons Show documentation
Show all versions of cloudpool.aws.commons Show documentation
Common utility classes for building Amazon AWS-based cloud pools.
package com.elastisys.scale.cloudpool.aws.commons.requests.ec2;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.Callable;
import com.amazonaws.AmazonClientException;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.services.ec2.model.DescribeSpotInstanceRequestsRequest;
import com.amazonaws.services.ec2.model.DescribeSpotInstanceRequestsResult;
import com.amazonaws.services.ec2.model.Filter;
import com.amazonaws.services.ec2.model.SpotInstanceRequest;
import com.amazonaws.services.ec2.model.SpotInstanceState;
import com.elastisys.scale.commons.net.retryable.Retryable;
import com.elastisys.scale.commons.net.retryable.Retryers;
/**
* A {@link Callable} task that, when executed, requests meta data for AWS EC2
* {@link SpotInstanceRequest}s in a region.
*
* The query can be limited to only return meta data for a particular group of
* {@link SpotInstanceRequest}s. Furthermore, query {@link Filter}s can be
* supplied to further narrow down the result set. Note that without
* {@link Filter}s, the result may contain requests in all states: open, active,
* cancelled, etc.
*
* If a set of spot instance request ids is specified, the query will be retried
* until meta data could be retrieved for all requested instances. This behavior
* is useful to handle the eventually consistent semantics of the EC2 API.
*
* AWS limits the number of filter values to some number (at the time of
* writing, that number is 200). For a detailed description of supported
* {@link Filter}s refer to the Amazon EC2 API.
*
* The query can be limited to only return meta data for a particular group of
* {@link SpotInstanceRequest}s via {@link Filter}s. Note that without
* {@link Filter}s, the result may contain {@link SpotInstanceRequest}s in all
* {@link SpotInstanceState}s (open, active, closed, cancelled, etc).
*
* For a detailed description of supported {@link Filter}s refer to the Amazon EC2 API.
*
* Note that due to the eventual consistency semantics of the Amazon API, a recently created EC2
* instance or spot instance request may not be immediately available for
* tagging. Therefore, it might be wise to use a retry strategy (with
* exponential back-off) when tagging a recently created resource.
*
* @see Retryable
* @see Retryers
*/
public class GetSpotInstanceRequests
extends AmazonEc2Request> {
/**
* A list of spot request ids of interest to limit the query to. If
* specified, meta data will only be fetched for these instances. If
* null
or empty list, meta data will be fetched for all spot
* requests.
*/
private final Collection spotRequestIds;
/**
* An (optional) list of filter to narrow the query. Only
* {@link SpotInstanceRequest}s matching the given filters will be returned.
*/
private final Collection filters;
/**
* Constructs a new {@link GetSpotInstanceRequests} task that will fetch all
* {@link SpotInstanceRequest}s in the region.
*
* @param awsCredentials
* The AWS security credentials to the account.
* @param region
* The AWS region of interest.
* @param clientConfig
* Client configuration options such as connection timeout, etc.
*/
public GetSpotInstanceRequests(AWSCredentials awsCredentials, String region,
ClientConfiguration clientConfig) {
this(awsCredentials, region, clientConfig, null);
}
/**
* Constructs a new {@link GetSpotInstanceRequests} task that will fetch all
* {@link SpotInstanceRequest}s in the region that match any of the
* specified filters.
*
* @param awsCredentials
* The AWS security credentials to the account.
* @param region
* The AWS region of interest.
* @param clientConfig
* Client configuration options such as connection timeout, etc.
* @param filters
* A list of filter to narrow the query. Only
* {@link SpotInstanceRequest}s matching the given filters will
* be returned. May be null
(no filters).
*/
public GetSpotInstanceRequests(AWSCredentials awsCredentials, String region,
ClientConfiguration clientConfig, Collection filters) {
this(awsCredentials, region, clientConfig, null, filters);
}
/**
* Constructs a new {@link GetSpotInstanceRequests} task that will only
* fetch meta data for the given spot instance request ids that match the
* given filters.
*
* @param awsCredentials
* The AWS security credentials to the account.
* @param region
* The AWS region of interest.
* @param clientConfig
* Client configuration options such as connection timeout, etc.
* @param spotRequestIds
* The spot request ids of interest. May be null
.
* @param filters
* A list of filter to narrow the query. Only
* {@link SpotInstanceRequest}s matching the given filters will
* be returned. May be null
(no filters).
*/
public GetSpotInstanceRequests(AWSCredentials awsCredentials, String region,
ClientConfiguration clientConfig, Collection spotRequestIds,
Collection filters) {
super(awsCredentials, region, clientConfig);
this.spotRequestIds = spotRequestIds;
this.filters = filters;
}
@Override
public List call() throws AmazonClientException {
DescribeSpotInstanceRequestsRequest request = new DescribeSpotInstanceRequestsRequest();
if (this.spotRequestIds != null) {
request.withSpotInstanceRequestIds(this.spotRequestIds);
}
if (this.filters != null) {
request.withFilters(this.filters);
}
DescribeSpotInstanceRequestsResult result = getClient().getApi()
.describeSpotInstanceRequests(request);
return result.getSpotInstanceRequests();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy