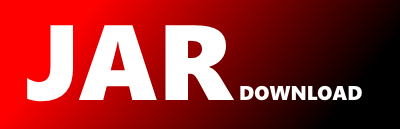
com.elastisys.scale.cloudpool.splitter.requests.RequestFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudpool.splitter Show documentation
Show all versions of cloudpool.splitter Show documentation
A cloud pool that uses a configured list of child cloud pools to carry out operations.
The newest version!
package com.elastisys.scale.cloudpool.splitter.requests;
import java.util.concurrent.Callable;
import com.elastisys.scale.cloudpool.api.CloudPool;
import com.elastisys.scale.cloudpool.api.types.MachinePool;
import com.elastisys.scale.cloudpool.api.types.MembershipStatus;
import com.elastisys.scale.cloudpool.api.types.PoolSizeSummary;
import com.elastisys.scale.cloudpool.api.types.ServiceState;
import com.elastisys.scale.cloudpool.splitter.Splitter;
import com.elastisys.scale.cloudpool.splitter.config.PrioritizedCloudPool;
/**
* A strategy for creating {@link Callable} tasks for carrying out operations
* against remote {@link PrioritizedCloudPool}s.
*
* @see Splitter
*/
public interface RequestFactory {
/**
* Creates a {@link Callable} that calls {@link CloudPool#getMachinePool()}
* on the remote cloud pool.
*
* @param cloudPool
* @return
*/
Callable newGetMachinePoolRequest(
PrioritizedCloudPool cloudPool);
/**
* Creates a {@link Callable} that calls {@link CloudPool#getPoolSize()} on
* the remote cloud pool.
*
* @param cloudPool
* @return
*/
Callable newGetPoolSizeRequest(
PrioritizedCloudPool cloudPool);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#setDesiredSize(int)} on the remote cloud pool.
*
* @param cloudPool
* @param desiredSize
* @return
*/
Callable newSetDesiredSizeRequest(PrioritizedCloudPool cloudPool,
int desiredSize);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#terminateMachine(String, boolean)} on the remote cloud
* pool.
*
* @param cloudPool
* @param machineId
* @param decrementDesiredSize
* @return
*/
Callable newTerminateMachineRequest(PrioritizedCloudPool cloudPool,
String machineId, boolean decrementDesiredSize);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#setServiceState(String, ServiceState)} on the remote
* cloud pool.
*
* @param cloudPool
* @param machineId
* @param serviceState
* @return
*/
Callable newSetServiceStateRequest(PrioritizedCloudPool cloudPool,
String machineId, ServiceState serviceState);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#setMembershipStatus(String, MembershipStatus)} on the
* remote cloud pool.
*
* @param cloudPool
* @param machineId
* @param membershipStatus
* @return
*/
Callable newSetMembershipStatusRequest(
PrioritizedCloudPool cloudPool, String machineId,
MembershipStatus membershipStatus);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#attachMachine(String)} on the remote cloud pool.
*
* @param cloudPool
* @param machineId
* @return
*/
Callable newAttachMachineRequest(PrioritizedCloudPool cloudPool,
String machineId);
/**
* Creates a {@link Callable} that calls
* {@link CloudPool#detachMachine(String, boolean)} on the remote cloud
* pool.
*
* @param cloudPool
* @param machineId
* @param decrementDesiredSize
* @return
*/
Callable newDetachMachineRequest(PrioritizedCloudPool cloudPool,
String machineId, boolean decrementDesiredSize);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy