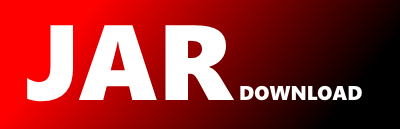
com.electronwill.nightconfig.json.JsonParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json_android Show documentation
Show all versions of json_android Show documentation
Powerful, easy-to-use and multi-language configuration library for the JVM - json_android module
package com.electronwill.nightconfig.json;
import com.electronwill.nightconfig.core.Config;
import com.electronwill.nightconfig.core.ConfigFormat;
import com.electronwill.nightconfig.core.io.*;
import com.electronwill.nightconfig.core.utils.FastStringReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.List;
/**
* A JSON parser.
*
* @author TheElectronWill
*/
public final class JsonParser implements ConfigParser {
private static final char[] SPACES = {' ', '\t', '\n', '\r'};
private static final char[] TRUE_LAST = {'r', 'u', 'e'}, FALSE_LAST = {'a', 'l', 's', 'e'};
private static final char[] NULL_LAST = {'u', 'l', 'l'};
private static final char[] NUMBER_END = {',', '}', ']', ' ', '\t', '\n', '\r'};
private final ConfigFormat configFormat;
private boolean emptyDataAccepted = false;
public JsonParser() {
this(JsonFormat.fancyInstance());
}
JsonParser(ConfigFormat configFormat) {
this.configFormat = configFormat;
}
@Override
public ConfigFormat getFormat() {
return configFormat;
}
/**
* @return true if the parser accepts empty data as a valid input, false otherwise (default)
*/
public boolean isEmptyDataAccepted() {
return emptyDataAccepted;
}
/**
* Enables or disables the acceptance of empty input data. False by default. If set to true,
* the parser will return an empty config (or an empty list in the case of a
* {@link #parseList(Reader)} call) when the input is empty.
*
* @param emptyDataAccepted true to accept empty data as a valid input, false to reject it
*/
public JsonParser setEmptyDataAccepted(boolean emptyDataAccepted) {
this.emptyDataAccepted = emptyDataAccepted;
return this;
}
/**
* Parses a JSON document, either a JSON object (parsed to a JsonConfig) or a JSON array
* (parsed to a List).
*
* @param json the data to parse
* @return either a JsonConfig or a List, depending on the document's type
*/
public Object parseDocument(String json) {
return parseDocument(new FastStringReader(json));
}
/**
* Parses a JSON document, either a JSON object (parsed to a JsonConfig) or a JSON array
* (parsed to a List).
*
* @param reader the Reader to parse
* @return either a JsonConfig or a List, depending on the document's type
*/
public Object parseDocument(Reader reader) {
CharacterInput input = new ReaderInput(reader);
if (input.peek() == -1) {
if (emptyDataAccepted) {
// If data is empty && we accept empty data => return empty config
return configFormat.createConfig();
} else {
throw new ParsingException("No json data: input is empty");
}
}
char firstChar = input.readCharAndSkip(SPACES);
if (firstChar == '{') {
return parseObject(input, configFormat.createConfig(), ParsingMode.MERGE);
}
if (firstChar == '[') {
return parseArray(input, new ArrayList<>(), ParsingMode.MERGE);
}
throw new ParsingException("Invalid first character for a json document: " + firstChar);
}
/**
* Parses a JSON object to a Config.
*/
@Override
public Config parse(Reader reader) {
Config config = JsonFormat.minimalInstance().createConfig();
parse(reader, config, ParsingMode.MERGE);
return config;
}
/**
* Parses a JSON object to a Config.
*/
@Override
public void parse(Reader reader, Config destination, ParsingMode parsingMode) {
CharacterInput input = new ReaderInput(reader);
if (input.peek() == -1) {
if (emptyDataAccepted) {
// If data is empty && we accept empty data => let the config as it is
return;
} else {
throw new ParsingException("No json data: input is empty");
}
}
char firstChar = input.readCharAndSkip(SPACES);
if (firstChar != '{') {
throw new ParsingException("Invalid first character for a json object: " + firstChar);
}
parsingMode.prepareParsing(destination);
parseObject(input, destination, parsingMode);
}
/**
* Parses a JSON array to a List.
*
* @param json the data to parse
* @return a List with the content of the parsed array
*/
public List parseList(String json) {
return parseList(new FastStringReader(json));
}
/**
* Parses a JSON array to a List.
*
* @param reader the Reader to parse
* @return a List with the content of the parsed array
*/
public List parseList(Reader reader) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy