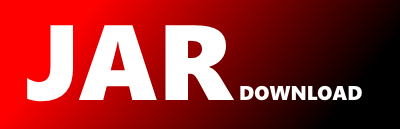
com.elephantdrummer.executor.base.ExecutorBase Maven / Gradle / Ivy
package com.elephantdrummer.executor.base;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Method;
import java.util.Date;
import java.util.concurrent.Callable;
import com.elephantdrummer.annotation.DrummerJob;
import com.elephantdrummer.annotation.apperance.SilentJob;
import com.elephantdrummer.exception.JobFinishedStatus;
import com.elephantdrummer.executor.base.structure.DrummerJobProvider;
import com.elephantdrummer.executor.base.structure.IJob;
import com.elephantdrummer.model.PtExecutor;
import com.elephantdrummer.model.PtJob;
import com.elephantdrummer.model.PtJobApplication;
import com.elephantdrummer.observer.base.ObserverBase;
import com.elephantdrummer.tool.HistoryBuffer;
import com.elephantdrummer.trigger.base.DrumTrigger;
/**
* Copyright 2018 Elephant Software Klaudiusz Wojtkowiak e-mail: [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
public abstract class ExecutorBase extends ObserverBase implements Callable,IJob{
protected HistoryBuffer historyBuffer;
private DrumTrigger trigger;
private Boolean silent=null;
private DrummerJobProvider jobLogicProvider;
private String jobClassName;
private Method jobMethod;
private PtJob ptJob;
private int poolSize=1;
private JobData jobData;
private boolean enabled=true;
private boolean initiated=false;
private boolean clusterSingleExecution=false;
private boolean abandoned=false;
private PtExecutor ptExecutor=null;
private volatile boolean underExecution;
private int clusterrecords=0;
private boolean skipExecutionWhenPreviousJobIsRunning=false;
private boolean cacheThreads;
public void init(DrummerJob drummerjobannotation,Method method, DrummerJobProvider joblogicclass,DrumTrigger trig){
setInitiated(true);
setPoolSize(drummerjobannotation.threads());
setCacheThreads(drummerjobannotation.cacheThreads());
setClusterSingleExecution(drummerjobannotation.clusterSingleExecution());
setSkipExecutionWhenPreviousJobIsRunning(drummerjobannotation.skipExecutionWhenPreviousJobIsRunning());
setTrigger(trig);
setJobClassName(joblogicclass.getClass().getName());
setJobMethod(method);
setJobLogicProvider(joblogicclass);
//inaczej gdy jest dao
ptExecutor=new PtExecutor();
ptExecutor.setName(this.getClass().getName());
}
protected final void startJobProcedure(){
setAbandoned(false);
if (getJobLogicProvider()==null==false){
getJobLogicProvider().beforeDrummerJobExecution();
}
if (Boolean.TRUE.equals(getSilent())==false){
getJobData().start();
PtJobApplication tp=getJobData().getPtJobPlatform();
getJobData().setExecutionComment(null);
if (isClusterSingleExecution()){
// clusterrecords=ptjobapplicationdao.updateForSingleClusterExecution(tp);
}
// tp=ptjobapplicationdao.findById(tp.getPtJobApplicationId());
getJobData().setPtJobPlatform(tp);
// tp.setLastStartDate(getJobData().getStartDate());
//
// if (isClusterSingleExecution()==false){
// tp.setStatus(JobStatus.EXECUTION.toString());
// }else{
// if (clusterrecords==0==false){
// tp.setStatus(JobStatus.EXECUTION.toString());
// }else{
// tp.setStatus(JobStatus.ABANDONED.toString());
// setAbandoned(true);
// }
// }
// tp.setPtExecutorId(ptExecutor.getId());
// ptjobapplicationdao.updateTransactional(tp);
}
}
protected final void finishJobProcedure(){
if (Boolean.TRUE.equals(getSilent())==false){
// jobFinishedTrigger.fire(getJobData().finish());
PtJobApplication tp=getJobData().getPtJobPlatform();
if (tp==null) tp=new PtJobApplication();
tp.setLastFinishDate(getJobData().getFinishDate());
tp.setStatus(JobStatus.WAITING.toString());
tp.setNextExecutionDate(getTrigger().getAfterNextRunTime(new Date()));
tp.setPtExecutorId(ptExecutor.getId());
if (isAbandoned()){
getJobData().setStatus(JobFinishedStatus.ABANDONED);
}
// ptjobapplicationdao.updateTransactional(tp);
// if (isClusterSingleExecution()&&clusterrecords>0){
// ptjobapplicationdao.clearForSingleClusterExecution(tp);
// }
if (isAbandoned()){
getJobData().setStatus(JobFinishedStatus.ABANDONED);
}
}
//Beware of null
if (Boolean.TRUE.equals(getSilent())==false){
getJobData().setTrigger(trigger);
// historyBuffer.addJob(getJobData());
}
if (getJobLogicProvider()==null==false){
getJobLogicProvider().afterDrummerJobExecution();
}
}
protected abstract JobType getJobType();
public JobData getJobData() {
if (jobData==null){
jobData=new JobData()
.setName(getJobClassName())
.setType(getJobType())
.setClazz(getJobLogicProvider().getClass());
jobData.setPtJob(new PtJob());
// if (isInitiated()==false) this.init();
}
return jobData;
}
protected void setJobData(JobData jobData) {
this.jobData = jobData;
}
@Override
public Class extends DrummerJobProvider> observeClass() {
return getJobLogicProvider().getClass();
}
private void setTrigger(DrumTrigger trig){
trigger=trig;
}
public DrumTrigger getTrigger(){
return trigger;
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
protected boolean isInitiated() {
return initiated;
}
private void setInitiated(boolean initiated) {
this.initiated = initiated;
}
private Boolean getSilent() {
if (silent==null){
setSilent(new Boolean(getJobMethod().isAnnotationPresent(SilentJob.class)));
}
return silent;
}
private void setSilent(Boolean silent) {
this.silent = silent;
}
protected void setJobExecutionComment(String comment){
if (comment==null==false&&comment.length()>511){
comment=comment.substring(0, 511);
}
getJobData().setExecutionComment(comment);
}
protected void handleThrowable(Throwable t){
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
t.printStackTrace(pw);
String comment=sw.toString(); // stack trace as a string
setJobExecutionComment(comment);
}
public DrummerJobProvider getJobLogicProvider() {
return jobLogicProvider;
}
private void setJobLogicProvider(DrummerJobProvider jobLogicProvider) {
this.jobLogicProvider = jobLogicProvider;
}
private String getJobClassName() {
return jobClassName;
}
private void setJobClassName(String jobClassName) {
this.jobClassName = jobClassName;
}
private Method getJobMethod() {
return jobMethod;
}
private void setJobMethod(Method jobMethod) {
this.jobMethod = jobMethod;
}
protected int getPoolSize() {
return poolSize;
}
private void setPoolSize(int poolSize) {
this.poolSize = poolSize;
}
public void destroy(){
// List listtp=ptjobapplicationdao.findByProperties(IPtJobApplicationDao.PT_JOB__ID, getPtJob().getPtJobId(), IPtJobApplicationDao.PT_APPLICATION_ID, platformInformation.getPtPlatform().getPtApplicationId());
//
// if (listtp==null==false&& listtp.isEmpty()==false){
//
// for (PtJobApplication tp:listtp){
// if (Boolean.TRUE.equals(getSilent())==false){
// tp.setStatus(JobStatus.DISABLED.toString());
// tp.setTriggerStep(new BigDecimal(getTrigger().getStep()));
// tp.setTriggerShift(new BigDecimal(getTrigger().getShift()*-1));
// if (tp.getForcedTriggerStep()==null==false&&tp.getForcedTriggerStep().longValue()>0) getTrigger().setStep(tp.getForcedTriggerStep().longValue());
// if (tp.getForcedTriggerShift()==null==false&&tp.getForcedTriggerShift().longValue()>0) getTrigger().setShift(tp.getForcedTriggerShift().longValue());
// tp.setPtExecutorId(ptExecutor.getId());
// tp.setNextExecutionDate(null);
//
// ptjobapplicationdao.updateTransactional(tp);
// }
// getJobData().setPtJobPlatform(tp);
// }
// }
getJobLogicProvider().preDestroy();
}
private boolean isClusterSingleExecution() {
return clusterSingleExecution;
}
private void setClusterSingleExecution(boolean clusterSingleExecution) {
this.clusterSingleExecution = clusterSingleExecution;
}
protected boolean isAbandoned() {
return abandoned;
}
private void setAbandoned(boolean abandoned) {
this.abandoned = abandoned;
}
protected boolean isUnderExecution() {
return underExecution;
}
protected void setUnderExecution(boolean underExecution) {
this.underExecution = underExecution;
}
protected boolean isSkipExecutionWhenPreviousJobIsRunning() {
return skipExecutionWhenPreviousJobIsRunning;
}
protected void setSkipExecutionWhenPreviousJobIsRunning(boolean skipExecutionWhenPreviousJobIsRunning) {
this.skipExecutionWhenPreviousJobIsRunning = skipExecutionWhenPreviousJobIsRunning;
}
public PtJob getPtJob() {
return ptJob;
}
private void setPtJob(PtJob ptJob) {
this.ptJob = ptJob;
}
public boolean isCacheThreads() {
return cacheThreads;
}
public void setCacheThreads(boolean cacheThreads) {
this.cacheThreads = cacheThreads;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy