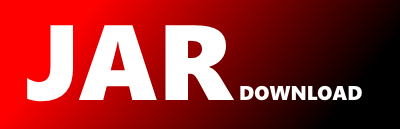
com.eligible.model.Payer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eligible-java Show documentation
Show all versions of eligible-java Show documentation
Java bindings for Eligible APIs (https://eligible.com).
package com.eligible.model;
import com.eligible.exception.APIConnectionException;
import com.eligible.exception.APIException;
import com.eligible.exception.AuthenticationException;
import com.eligible.exception.InvalidRequestException;
import com.eligible.model.payer.Endpoint;
import com.eligible.net.APIResource;
import com.eligible.net.RequestMethod;
import com.eligible.net.RequestOptions;
import com.google.gson.reflect.TypeToken;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
@Getter
@EqualsAndHashCode(callSuper=false)
public class Payer extends APIResource {
String payerId;
List names;
String createdAt;
String updatedAt;
List supportedEndpoints;
String eligibleId;
public static Payer retrieve(String id)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return retrieve(id, null);
}
public static List all()
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(null, null);
}
public static List all(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(params, null);
}
public static List searchOptions()
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return searchOptions((RequestOptions) null);
}
public static SearchOptions searchOptions(String payerId)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return searchOptions(payerId, null);
}
public static Payer retrieve(String id, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return request(RequestMethod.GET, instanceURL(Payer.class, id), null, Payer.class, options);
}
public static List all(RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(null, options);
}
public static List all(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
Type listType = new TypeToken>(){}.getType();
return request(RequestMethod.GET, classURL(Payer.class), params, listType, options);
}
public static List searchOptions(RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return SearchOptions.all(options);
}
public static SearchOptions searchOptions(String payerId, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return SearchOptions.retrieve(payerId, options);
}
public String getId() {
return getPayerId();
}
@Getter
@EqualsAndHashCode(callSuper=false)
public static class SearchOptions extends APIResource {
String payerId;
List> searchOptions;
String eligibleId;
public static List all()
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(null);
}
public static SearchOptions retrieve(String payerId)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return retrieve(payerId, null);
}
public static List all(RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
Type listType = new TypeToken>(){}.getType();
return request(RequestMethod.GET, classURL(SearchOptions.class), null, listType, options);
}
public static SearchOptions retrieve(String payerId, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return request(RequestMethod.GET, instanceURL(SearchOptions.class, payerId), null, SearchOptions.class, options);
}
protected static String classURL(Class> clazz) {
return String.format("%s/%s", APIResource.classURL(Payer.class), className(clazz));
}
protected static String instanceURL(Class> clazz, String id) throws InvalidRequestException {
return String.format("%s/%s", APIResource.instanceURL(Payer.class, id), className(clazz));
}
public String getId() {
return getPayerId();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy