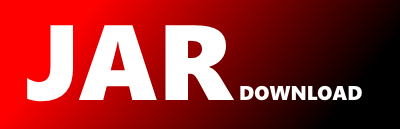
com.eligible.json.deserializer.DatesDeserializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eligible-java Show documentation
Show all versions of eligible-java Show documentation
Java bindings for Eligible APIs (https://eligible.com).
package com.eligible.json.deserializer;
import com.eligible.model.coverage.Date;
import com.eligible.model.coverage.Dates;
import com.eligible.net.APIResource;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonParseException;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
/**
* {@link JsonDeserializer} for deserializing {@link Dates}.
*/
public class DatesDeserializer implements JsonDeserializer {
private static final Type DATE_LIST_TYPE = new TypeToken>() { }.getType();
/** {@inheritDoc} */
public Dates deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context)
throws JsonParseException {
final Gson gson = APIResource.GSON;
// Dates could be a list of Date.class, or a list of list of Date.class. Merging all list into one
if (json.isJsonArray()) {
Dates collection = new Dates();
collection.setData(new ArrayList());
JsonArray jsonDates = json.getAsJsonArray();
for (JsonElement jsonDate : jsonDates) {
if (jsonDate.isJsonArray()) {
List dates = gson.fromJson(jsonDate, DATE_LIST_TYPE);
collection.addAll(dates);
} else {
Date date = gson.fromJson(jsonDate, Date.class);
collection.add(date);
}
}
return collection;
} else {
throw new IllegalArgumentException("Can not deserialize class Dates. Expected an array but got: " + json);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy