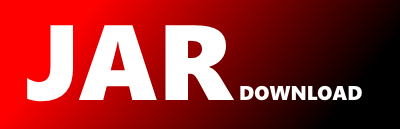
com.eligible.model.Coverage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eligible-java Show documentation
Show all versions of eligible-java Show documentation
Java bindings for Eligible APIs (https://eligible.com).
package com.eligible.model;
import com.eligible.exception.APIConnectionException;
import com.eligible.exception.APIException;
import com.eligible.exception.AuthenticationException;
import com.eligible.exception.InvalidRequestException;
import com.eligible.model.coverage.Demographics;
import com.eligible.model.coverage.Insurance;
import com.eligible.model.coverage.Plan;
import com.eligible.model.coverage.Service;
import com.eligible.model.coverage.costestimates.CostEstimate;
import com.eligible.model.coverage.costestimates.SearchOptions;
import com.eligible.model.coverage.costestimates.Warning;
import com.eligible.model.coverage.medicare.DateRange;
import com.eligible.model.coverage.medicare.HistoricDetails;
import com.eligible.model.coverage.medicare.RequestedProcedureCode;
import com.eligible.model.coverage.medicare.RequestedServiceType;
import com.eligible.net.APIResource;
import com.eligible.net.RequestMethod;
import com.eligible.net.RequestOptions;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import java.util.List;
import java.util.Map;
@Getter
@EqualsAndHashCode(callSuper=false)
public class Coverage extends APIResource {
String createdAt;
String eligibleId;
List knownIssues;
Demographics demographics;
Insurance insurance;
Plan plan;
List services;
public static Coverage all(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(params, null);
}
public static Medicare medicare(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return medicare(params, null);
}
public static CostEstimates costEstimate(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return costEstimate(params, null);
}
public static Coverage all(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
String url = String.format("%s/all", singleClassURL(Coverage.class));
return request(RequestMethod.GET, url, params, Coverage.class, options);
}
public static Medicare medicare(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return Medicare.all(params, options);
}
public static CostEstimates costEstimate(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return CostEstimates.all(params, options);
}
public String getId() {
return getEligibleId();
}
@Getter
@EqualsAndHashCode(callSuper=false)
public static class Medicare extends APIResource {
String createdAt;
String eligibleId;
List knownIssues;
String dateOfDeath;
String lastName;
String firstName;
String middleName;
String memberId;
String groupId;
String groupName;
String dob;
String gender;
String relationship;
String relationshipCode;
Address address;
String payerName;
String payerId;
String planNumber;
DateRange eligibiltyDates;
DateRange eligibilityDates;
DateRange inactivityDates;
Map planTypes;
Map planDetails;
List requestedServiceTypes;
List requestedProcedureCodes;
HistoricDetails history;
public static Medicare all(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(params, null);
}
public static Medicare all(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
String url = String.format("%s/%s", singleClassURL(Coverage.class), className(Medicare.class));
return request(RequestMethod.GET, url, params, Medicare.class, options);
}
public String getId() {
return getEligibleId();
}
}
@Getter
@EqualsAndHashCode(callSuper=false)
public static class CostEstimates extends APIResource {
String createdAt;
String eligibleId;
List knownIssues;
Demographics demographics;
Insurance insurance;
Plan plan;
List services;
SearchOptions searchOptions;
List costEstimates;
List warnings;
Boolean success;
public static CostEstimates all(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
return all(params, null);
}
public static CostEstimates all(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, APIException {
String url = String.format("%s/%s", singleClassURL(Coverage.class), className(CostEstimates.class));
return request(RequestMethod.GET, url, params, CostEstimates.class, options);
}
public String getId() {
return getEligibleId();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy